Does the below program use recursion to create a sierpinski triangle? Souce code: package application; public class Triangle { publicstaticvoid main(String [] args) { TriangleFrame tf = new TriangleFrame(); } } package application; import java.awt.*; import javax.swing.*; public class SierpinskiGasket extends JPanel { public SierpinskiGasket() { } public void drawTriangle (Graphics g, int x, int y, int width, int height) { if (height == 1) { g.drawRect(x, y, width, height); } else { drawTriangle(g, x + (width / 4), y, width / 2, height / 2); drawTriangle(g, x, y + (height / 2), width / 2, height / 2); drawTriangle(g, x + (width / 2), y + (height / 2), width/ 2, height / 2); } } public void paintComponent (Graphics g) { super.paintComponent(g); drawTriangle(g, 0, 0, this.getWidth(), this.getHeight()); } }
Does the below program use recursion to create a sierpinski triangle?
Souce code:
package application;
public class Triangle {
publicstaticvoid main(String [] args) {
TriangleFrame tf = new TriangleFrame();
}
}
package application;
import java.awt.*;
import javax.swing.*;
public class SierpinskiGasket extends JPanel {
public SierpinskiGasket() {
}
public void drawTriangle (Graphics g, int x, int y, int width, int height) {
if (height == 1) {
g.drawRect(x, y, width, height);
}
else {
drawTriangle(g, x + (width / 4), y, width / 2, height / 2);
drawTriangle(g, x, y + (height / 2), width / 2, height / 2);
drawTriangle(g, x + (width / 2), y + (height / 2), width/ 2, height / 2);
}
}
public void paintComponent (Graphics g) {
super.paintComponent(g);
drawTriangle(g, 0, 0, this.getWidth(), this.getHeight());
}
}

The Sierpinski triangle is a well-known fractal pattern that can be created by recursively subdividing an equilateral triangle into smaller equilateral triangles. It exhibits self-similar and intricate geometric properties.
Step by step
Solved in 3 steps

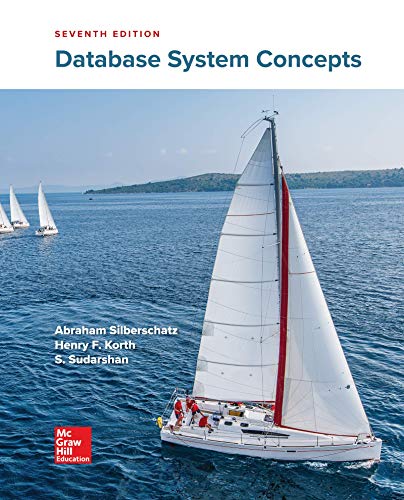
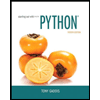
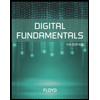
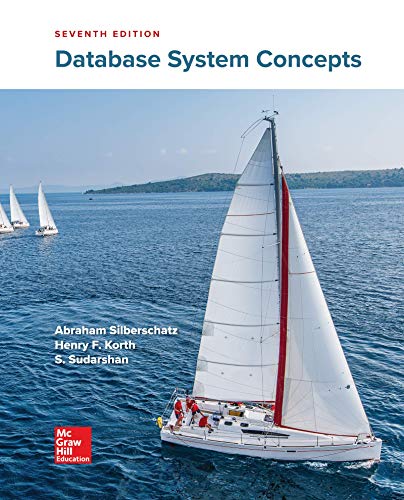
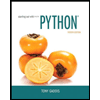
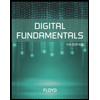
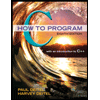
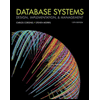
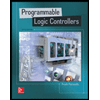