Let's work on some basic skills by creating a program that contains a few experiments with arrays. If you are asked to print out a value, be sure to print a label that indicates what the value represents, unless otherwise indicated. For example, if you are asked to print a variable z, the output might look like this: z: 8.0 Create a new Eclipse project. Download the source code file Array_Lab1a.java. Import the source code file into your project. Add your name and section number in the comment block near the top, and then add Java statements that perform each of the following tasks: a) Create an array x of 20 random double values, each between 1.0 and 100.0 (i.e., declare/create the array, then populate it with 20 random doubles). b) Output the number of items in the array by printing the expression x.length. c) Output the first array item, x[0]. d) Output the last array item. Be careful to choose the right index. e) Print the expression x [x.length - 1]. Why is this value the same as in part (d)? f) Use a for loop to print all the values in the array without labels. g) Use a loop to print all the values in the array with labels to indicate what each element is. h) Use a for loop to print all the values in the array in reverse order with labels
Let's work on some basic skills by creating a program that contains a few experiments with arrays. If you are asked to print out a value, be sure to print a label that indicates what the value represents, unless otherwise indicated. For example, if you are asked to print a variable z, the output might look like this: z: 8.0 Create a new Eclipse project. Download the source code file Array_Lab1a.java. Import the source code file into your project. Add your name and section number in the comment block near the top, and then add Java statements that perform each of the following tasks: a) Create an array x of 20 random double values, each between 1.0 and 100.0 (i.e., declare/create the array, then populate it with 20 random doubles). b) Output the number of items in the array by printing the expression x.length. c) Output the first array item, x[0]. d) Output the last array item. Be careful to choose the right index. e) Print the expression x [x.length - 1]. Why is this value the same as in part (d)? f) Use a for loop to print all the values in the array without labels. g) Use a loop to print all the values in the array with labels to indicate what each element is. h) Use a for loop to print all the values in the array in reverse order with labels
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Lab 23.1: Basic Array Skills
Let's work on some basic skills by creating a
program that contains a few experiments
with arrays. If you are asked to print out a
value, be sure to print a label that indicates
what the value represents, unless otherwise
indicated. For example, if you are asked to
print a variable z, the output might look like
this:
z: 8.0
Create a new Eclipse project. Download the
source code file Array_Lab1a.java. Import
the source code file into your project. Add
your name and section number in the
comment block near the top, and
then add Java statements that perform each
of the following tasks:
a) Create an array x of 20 random
double values, each between 1.0 and
100.0 (i.e., declare/create the array,
then populate it with 20 random
doubles).
b) Output the number of items in the
array by printing the expression
x.length.
c) Output the first array item, x[0].
d) Output the last array item. Be careful
to choose the right index.
e) Print the expression x [x.length
1]. Why is this value the same as in
part (d)?
f) Use a for loop to print all the values in
the array without labels.
g) Use a loop to print all the values in
the array with labels to indicate what
each element is.
h) Use a for loop to print all the values
in the array in reverse order with labels](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F44ae875a-f598-455b-b111-f9246b312123%2Fea33b08c-ae70-415c-8887-affbf59774c8%2F36fqcg9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Lab 23.1: Basic Array Skills
Let's work on some basic skills by creating a
program that contains a few experiments
with arrays. If you are asked to print out a
value, be sure to print a label that indicates
what the value represents, unless otherwise
indicated. For example, if you are asked to
print a variable z, the output might look like
this:
z: 8.0
Create a new Eclipse project. Download the
source code file Array_Lab1a.java. Import
the source code file into your project. Add
your name and section number in the
comment block near the top, and
then add Java statements that perform each
of the following tasks:
a) Create an array x of 20 random
double values, each between 1.0 and
100.0 (i.e., declare/create the array,
then populate it with 20 random
doubles).
b) Output the number of items in the
array by printing the expression
x.length.
c) Output the first array item, x[0].
d) Output the last array item. Be careful
to choose the right index.
e) Print the expression x [x.length
1]. Why is this value the same as in
part (d)?
f) Use a for loop to print all the values in
the array without labels.
g) Use a loop to print all the values in
the array with labels to indicate what
each element is.
h) Use a for loop to print all the values
in the array in reverse order with labels

Transcribed Image Text:to indicate what each element is.
Lab 23.2: More Array Skills and
Array Algorithms
Let's continue to work on our fundamental
array skills with a few more experiments. As
in Lab 23.1, if you are asked to print out a
value, be sure to print a label that indicates
what the value represents, unless otherwise
indicated. For example, if you are asked to
print a variable z, the output might look like
this:
z: 8.0
Download the source code file
Array_Lab1b.java into your project. Import
the source code file into your project. Add
your name and section number in the
comment block near the top, and then add
Java statements that perform each of the
following tasks:
a) Declare/create an array x of 20
random doubles (as in part (a) of Lab
23.1).
b) Use a for loop to compute and
output the total of all the elements in
array x.
c) Compute and output the minimum
value in x. Your code should find this
value by:
1. setting a double variable (e.g., names
smallest) to the first array element
2. using a loop and examining each
element in the array, starting with
the second array element:
If that element is smaller than
smallest, reset smallest to that
element.
d) Repeat part (c), but output the
minimum value and its index in the
array (instead of just the minimum
value).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
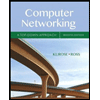
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
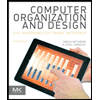
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
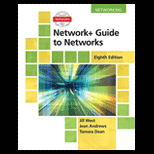
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
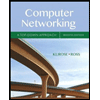
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
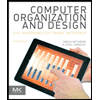
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
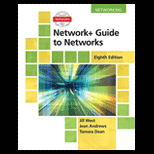
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
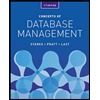
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
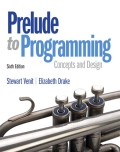
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
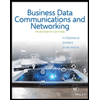
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY