lease modify my program so that the output will print like what is in the picture. Thank you in advance.
Please modify my program so that the output will print like what is in the picture. Thank you in advance.
import java.util.*;
public class sales
{
public static void main(String[] args)
{
Scanner kbd = new Scanner(System.in);
String[] names;
String[] address;
String[] age;
// String[] age;
int n = 0;
boolean successfulRead=false;
do
{
try
{
System.out.println("How many names will be sorted: ");
n = Integer.parseInt(kbd.nextLine());
if (n >=0 )
{
successfulRead = true;
}
else
{
System.out.println("The amount cannot be a negative amount");
successfulRead = false;
}
}
catch (NumberFormatException e)
{
successfulRead = false;
System.out.println("You have enetered an invalid amount");
}
} while (!successfulRead);
names = new String[n];
address = new String[n];
age = new String[n];
// Populate array names
for (int z = 0; z < names.length; z++)
{
System.out.print("Enter name of salesmen " + (z + 1) + ": ");
names[z] = kbd.nextLine();
}
for (int z = 0; z < address.length; z++)
{
System.out.print("Enter address of salesmen " + (z + 1) + ": ");
address[z]= kbd.nextLine();
}
for (int z = 0; z < age.length; z++)
{
System.out.print("Enter age of salesmen " + (z + 1) + ": ");
age[z]= kbd.nextLine();
}
// Sort the names array
balloonSortArray2(names);
//Show elements of names array
System.out.print("Sorted name of salesmen ");
showElements(names);
// Sort the address array
balloonSortArray2(address);
//Show elements of address array
System.out.print("Sorted address of salesmen ");
showElements(address);
// Sort the age array
balloonSortArray2(age);
//Show elements of age array
System.out.print("Sorted age of salesmen ");
showElements(age);
}
public static void balloonSortArray2(String[] given)
{
for (int x = 0; x < given.length - 1; x++)
{
for (int y = x + 1; y < given.length; y++)
{
if (given[x].compareTo(given[y]) > 0)
{
String temp = given[x];
given[x] = given[y];
given[y] = temp;
} // end of if
} // end of second for
} // end of first for
} // end of method
public static void showElements(String[] array)
{
System.out.print("Sorted Name \t\t Sorted Address \t\t Sorted age");
for (int x = 0; x < array.length; x++)
System.out.println(array[x]);
}
}


Step by step
Solved in 2 steps with 1 images

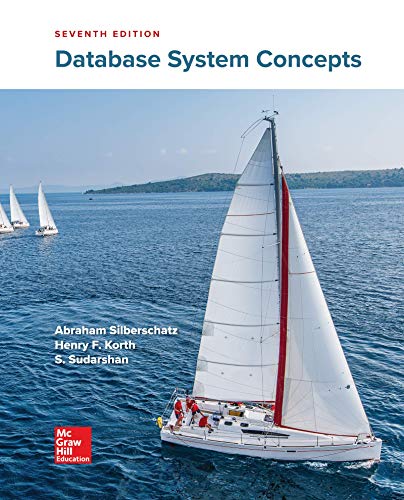
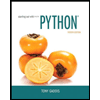
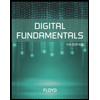
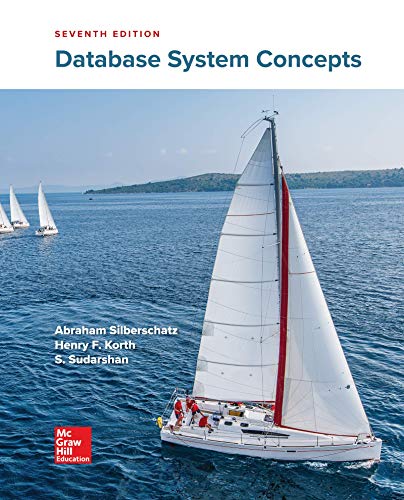
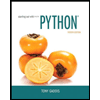
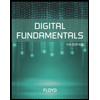
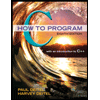
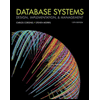
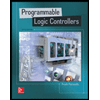