JAVA PPROGRAM I have provided the failed test cases and the inputs as a screenshot. Please Modify this program with further modifications as listed below: The program must pass the test case when uploaded to Hypergrade. ALSO, take out the following in the program: System.out.println("Program terminated."); because the test case does not need it. And change this in the program: Please enter the file name or type QUIT to exit:\n so it reperats once for every test case. import java.io.*; import java.util.ArrayList; import java.util.Scanner; public class SymmetricalNameMatcher { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { // Prompt the user to enter a file name or 'QUIT' to exit. System.out.print("Please enter the file name or type QUIT to exit:\n"); fileName = scanner.nextLine(); if (fileName.equalsIgnoreCase("QUIT")) { // If the user types 'QUIT', terminate the program. break; } File file = new File(fileName); if (!file.exists()) { // If the file doesn't exist, display an error message and continue to the next iteration. System.out.println("File '" + fileName + "' is not found."); continue; } if (file.length() == 0) { // If the file is empty, display an error message and continue to the next iteration. System.out.println("File '" + fileName + "' is empty."); continue; } ArrayList names = new ArrayList<>(); int lineCount = 0; try (BufferedReader reader = new BufferedReader(new FileReader(file))) { String line; // Read names from the file and store them in the 'names' ArrayList. while ((line = reader.readLine()) != null) { names.add(line); lineCount++; } } catch (IOException e) { e.printStackTrace(); } int matches = 0; // Compare names in symmetrical positions. for (int i = 0; i < lineCount / 2; i++) { String name1 = names.get(i); String name2 = names.get(lineCount - 1 - i); if (name1.equals(name2)) { // If a match is found, print the match details. System.out.println("Match found: '" + name1 + "' on lines " + (i + 1) + " and " + (lineCount - i) + "."); matches++; } } if (matches == 0) { // If no matches were found, display a message. System.out.println("No matches found."); } else { // Display the total number of matches found. System.out.println("Total of " + matches + " matches found."); } } while (true); // Close the scanner. scanner.close(); } } Test Case 1 Please enter the file name or type QUIT to exit:\n input1.txtENTER Match found: 'Michael' on lines 1 and 8.\n Match found: 'Cassandra' on lines 3 and 6.\n Total of 2 matches found.\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2.txtENTER Match found: 'Michael' on lines 1 and 9.\n Match found: 'Cassandra' on lines 3 and 7.\n Total of 2 matches found.\n Test Case 3 Please enter the file name or type QUIT to exit:\n input3.txtENTER File 'input3.txt' is empty.\n Test Case 4 Please enter the file name or type QUIT to exit:\n input4.txtENTER Match found: 'Michael' on lines 1 and 16.\n Match found: 'Joshua' on lines 2 and 15.\n Match found: 'Cassandra' on lines 3 and 14.\n Match found: 'Joseph' on lines 4 and 13.\n Match found: 'William' on lines 5 and 12.\n Match found: 'Matthew' on lines 6 and 11.\n Match found: 'James' on lines 7 and 10.\n Match found: 'Steven' on lines 8 and 9.\n Total of 8 matches found.\n Test Case 5 Please enter the file name or type QUIT to exit:\n input5.txtENTER File 'input5.txt' is not found.\n Please re-enter the file name or type QUIT to exit:\n input1.txtENTER Match found: 'Michael' on lines 1 and 8.\n Match found: 'Cassandra' on lines 3 and 6.\n Total of 2 matches found.\n Test Case 6 Please enter the file name or type QUIT to exit:\n qUiTENTER Test Case 7 Please enter the file name or type QUIT to exit:\n input5.txtENTER File 'input5.txt' is not found.\n Please re-enter the file name or type QUIT to exit:\n quitENTER
System.out.println("Program terminated."); because the test case does not need it. And change this in the program: Please enter the file name or type QUIT to exit:\n so it reperats once for every test case.
import java.io.*;
import java.util.ArrayList;
import java.util.Scanner;
public class SymmetricalNameMatcher {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String fileName;
do {
// Prompt the user to enter a file name or 'QUIT' to exit.
System.out.print("Please enter the file name or type QUIT to exit:\n");
fileName = scanner.nextLine();
if (fileName.equalsIgnoreCase("QUIT")) {
// If the user types 'QUIT', terminate the program.
break;
}
File file = new File(fileName);
if (!file.exists()) {
// If the file doesn't exist, display an error message and continue to the next iteration.
System.out.println("File '" + fileName + "' is not found.");
continue;
}
if (file.length() == 0) {
// If the file is empty, display an error message and continue to the next iteration.
System.out.println("File '" + fileName + "' is empty.");
continue;
}
ArrayList<String> names = new ArrayList<>();
int lineCount = 0;
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line;
// Read names from the file and store them in the 'names' ArrayList.
while ((line = reader.readLine()) != null) {
names.add(line);
lineCount++;
}
} catch (IOException e) {
e.printStackTrace();
}
int matches = 0;
// Compare names in symmetrical positions.
for (int i = 0; i < lineCount / 2; i++) {
String name1 = names.get(i);
String name2 = names.get(lineCount - 1 - i);
if (name1.equals(name2)) {
// If a match is found, print the match details.
System.out.println("Match found: '" + name1 + "' on lines " + (i + 1) + " and " + (lineCount - i) + ".");
matches++;
}
}
if (matches == 0) {
// If no matches were found, display a message.
System.out.println("No matches found.");
} else {
// Display the total number of matches found.
System.out.println("Total of " + matches + " matches found.");
}
} while (true);
// Close the scanner.
scanner.close();
}
}
Test Case 1
input1.txtENTER
Match found: 'Michael' on lines 1 and 8.\n
Match found: 'Cassandra' on lines 3 and 6.\n
Total of 2 matches found.\n
Test Case 2
input2.txtENTER
Match found: 'Michael' on lines 1 and 9.\n
Match found: 'Cassandra' on lines 3 and 7.\n
Total of 2 matches found.\n
Test Case 3
input3.txtENTER
File 'input3.txt' is empty.\n
Test Case 4
input4.txtENTER
Match found: 'Michael' on lines 1 and 16.\n
Match found: 'Joshua' on lines 2 and 15.\n
Match found: 'Cassandra' on lines 3 and 14.\n
Match found: 'Joseph' on lines 4 and 13.\n
Match found: 'William' on lines 5 and 12.\n
Match found: 'Matthew' on lines 6 and 11.\n
Match found: 'James' on lines 7 and 10.\n
Match found: 'Steven' on lines 8 and 9.\n
Total of 8 matches found.\n
Test Case 5
input5.txtENTER
File 'input5.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.txtENTER
Match found: 'Michael' on lines 1 and 8.\n
Match found: 'Cassandra' on lines 3 and 6.\n
Total of 2 matches found.\n
Test Case 6
qUiTENTER
Test Case 7
input5.txtENTER
File 'input5.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
quitENTER



Step by step
Solved in 4 steps with 3 images

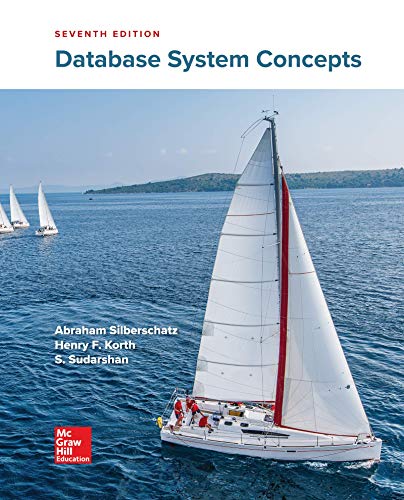
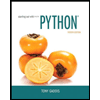
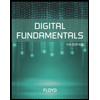
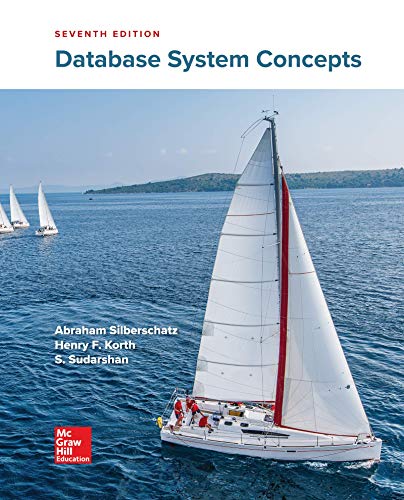
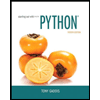
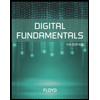
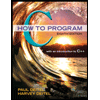
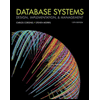
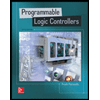