+ is my answer correct ? Write a function to convert a given string : Answer: #include #include using namespace std; void toUpper(string &str) { for (int i = 0; i < str.length(); i++) { str[i] = toupper(str[i]); } } void toLower(string &str) { for (int i = 0; i < str.length(); i++) { str[i] = tolower(str[i]); } } int main() { string str = "khaled"; toUpper(str); cout << str << endl; str = "MOHAMMED"; toLower(str); cout << str << endl; return 0; } Write a function to calculate the sum of the even number from 1 - 100. Answer: #include using namespace std; int sumEven() { int sum = 0; for (int i = 1; i <= 100; i++) { if (i % 2 == 0) { sum += i; } } return sum; } int main() { cout << sumEven
C++
is my answer correct ?
- Write a function to convert a given string :
Answer:
#include <iostream>
#include <string>
using namespace std;
void toUpper(string &str)
{
for (int i = 0; i < str.length(); i++)
{
str[i] = toupper(str[i]);
}
}
void toLower(string &str)
{
for (int i = 0; i < str.length(); i++)
{
str[i] = tolower(str[i]);
}
}
int main()
{
string str = "khaled";
toUpper(str);
cout << str << endl;
str = "MOHAMMED";
toLower(str);
cout << str << endl;
return 0;
}
- Write a function to calculate the sum of the even number from 1 - 100.
Answer:
#include <iostream>
using namespace std;
int sumEven() {
int sum = 0;
for (int i = 1; i <= 100; i++) {
if (i % 2 == 0) {
sum += i;
}
}
return sum;
}
int main() {
cout << sumEven() << endl;
return 0;
}

Step by step
Solved in 2 steps with 2 images

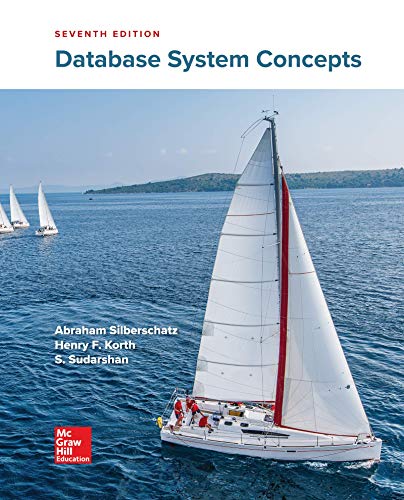
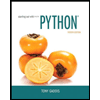
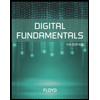
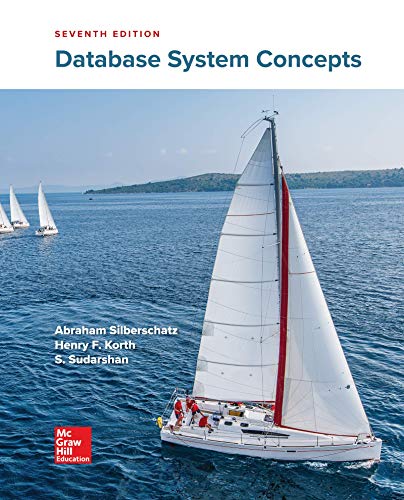
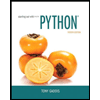
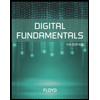
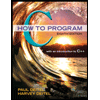
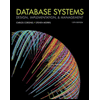
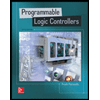