Inheritance, Polymporhism, and Dynamic Dispatch Please answer the following questions about type conformance and dynamic binding. Please copy/paste each table into your answer area, then fill in your answers in the tables for A, B and C. Here are some class definitions: public class Game{ public void players() { System.out.println(“1 or more”); } } public class Chess extends Game { public void boardSize() { System.out.println(“8 by 8”); } public void players() { System.out.println(“2”); } } public class ThreeDChess extends Chess{ public void boardSize() {System.out.println(“7 levels”); } public void hasMoveableBoard() { System.out.println(“true”); } } Assume that these statements have already executed: Game g = new Game(); Chess c = new Chess(); ThreeDChess t = new ThreeDChess(); Object og = g; Game gc = c; Game gt = t; // Add new statements here ONE AT A TIME A) Complete the following table (copy/paste into your answer): Variable Static Type Dynamic Type og gc gt B) Explain what would happen if each of the following statements were inserted ONE AT A TIME in the spot marked above. Complete the following table for each statement: Will the statement compile? If the answer is no, stop. If yes, what are the static and dynamic types of the variable declared? Statement Will it compile? (Y/N) If it compiles, static type? If it compiles, dynamic type? Game gx = gc; Game gx = og; Game gx = (Game)og; Chess cx = (Chess)gt; ThreeDChess tx = (ThreeDChess)gc; C) Finally, explain what would happen if each of the following statements were inserted ONE AT A TIME in the spot marked above. Answer the following questions for each statement: Will the statement compile? If yes, would the statement throw a ClassCastException? If no, then explain what output is shown Statement Compiles? Throws ClassCastException? Output? gc.players(); og.players(); t.players(); ((ThreeDChess)gc).hasMoveableBoard();
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Inheritance, Polymporhism, and Dynamic Dispatch
Please answer the following questions about type conformance and dynamic binding. Please copy/paste each table into your answer area, then fill in your answers in the tables for A, B and C.
Here are some class definitions:
public class Game{
public void players() { System.out.println(“1 or more”); }
}
public class Chess extends Game {
public void boardSize() { System.out.println(“8 by 8”); }
public void players() { System.out.println(“2”); }
}
public class ThreeDChess extends Chess{
public void boardSize() {System.out.println(“7 levels”); }
public void hasMoveableBoard() { System.out.println(“true”); }
}
Assume that these statements have already executed:
Game g = new Game();
Chess c = new Chess();
ThreeDChess t = new ThreeDChess();
Object og = g;
Game gc = c;
Game gt = t;
// Add new statements here ONE AT A TIME
A) Complete the following table (copy/paste into your answer):
Variable | Static Type | Dynamic Type |
og | ||
gc | ||
gt |
B) Explain what would happen if each of the following statements were inserted ONE AT A TIME in the spot marked above. Complete the following table for each statement:
- Will the statement compile? If the answer is no, stop.
- If yes, what are the static and dynamic types of the variable declared?
Statement | Will it compile? (Y/N) |
If it compiles, |
If it compiles, dynamic type? |
Game gx = gc; | |||
Game gx = og; | |||
Game gx = (Game)og; | |||
Chess cx = (Chess)gt; | |||
ThreeDChess tx = (ThreeDChess)gc; |
C) Finally, explain what would happen if each of the following statements were inserted ONE AT A TIME in the spot marked above. Answer the following questions for each statement:
- Will the statement compile?
- If yes, would the statement throw a ClassCastException?
- If no, then explain what output is shown
Statement | Compiles? | Throws ClassCastException? | Output? |
gc.players(); | |||
og.players(); | |||
t.players(); | |||
((ThreeDChess)gc).hasMoveableBoard(); |

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

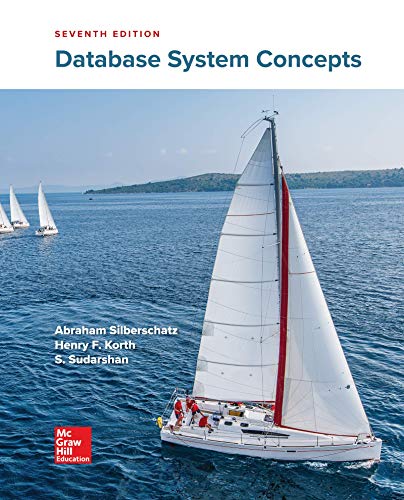
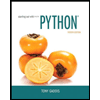
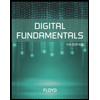
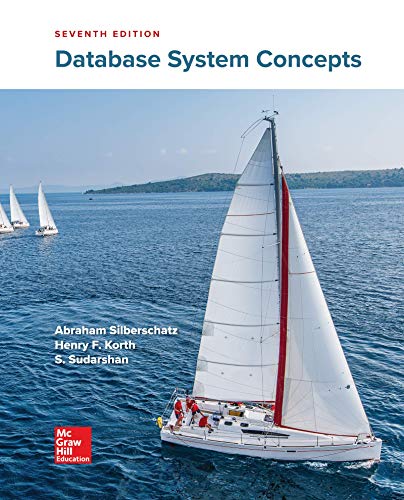
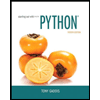
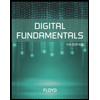
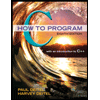
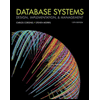
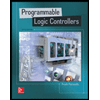