InfoProcessorTest.java import java.util.*; /** * * Class to process and extract information from a list of lines. * */ public class InfoProcessorTest { /** * * List of lines to process. * */ private ArrayList lines =new ArrayList(); /** * * Creates InfoProcessor with given list of lines. * * @param lines to process * */ public InfoProcessorTest(ArrayList lines) { this.lines = lines; } /** * * Gets the course name from the list of lines. * * First, finds the line that starts with "Course:". * * Second, gets the String on the very next line, which should be the course * name. * * Third, returns the String from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the course name * * * * Example(s): * * - If the ArrayList lines contains: "Course:" and "CIT590", and we * call * * getCourseName(), we'll get "CIT590". * * * * - If the ArrayList lines contains: "Course:" and "CIT 593", and we * call * * getCourseName(), we'll get "CIT 593". * * * * @return course name * */ public String getCourseName() { return getNextStringStartsWith("Course"); } /** * * Gets the course ID from the list of lines. * * First, finds the line that starts with "CourseID:". * * Second, gets the String on the very next line, which should be the course ID. * * Third, casts the String to an int and returns it from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the course ID * * * * Example(s): * * - If the ArrayList lines contains: "CourseID:" and "590", and we call * * getCourseId(), we'll get the int 590. * * * * - If the ArrayList lines contains: "CourseID" and "593", and we call * * getCourseId(), we'll get the int 593. * * * * @return course ID * */ publicint getCourseId() { String courseId = getNextStringStartsWith("CourseID:"); int cid = Integer.parseInt(courseId); return cid; } /** * * Gets the student ID from the list of lines. * * First, finds the line that starts with "StudentID:". * * Second, gets the String on the very next line, which should be the student * ID. * * Third, casts the String to an int and returns it from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the student ID * * * * Example(s): * * - If the ArrayList lines contains: "StudentID:" and "101", and we * call * * getStudentId(), we'll get the int 101. * * * * - If the ArrayList lines contains: "StudentID" and "5554", and we * call * * getStudentId(), we'll get the int 5554. * * * * @return student ID * */ publicint getStudentId() { String studentId = getNextStringStartsWith("CourseID:"); int sid = Integer.parseInt(studentId); return sid; } /** * * To be used by "getCourseName", "getCourseId", and "getStudentId" methods. * * * * Gets the String that follows the line that starts with the given String. * * Gets and returns the String on the very next line. * * If the given String doesn't exist, should return null. * * * * Example(s): * * - If an ArrayList lines contains: "hello" and "world", and we call * * getNextStringStartsWith("hello"), we'll get the String "world". * * * * - If an ArrayList lines contains: "Course" and "CIT590", and we call * * getNextStringStartsWith("Course"), we'll get the String "CIT590". * * * * - If an ArrayList lines contains: "hello" and "world", and we call * * getNextStringStartsWith("goodbye"), we'll get null. * * * * @param str to look for in lines * * @return String from the very next line * */ String getNextStringStartsWith(String str) { Iterator iterator = lines.iterator(); while (iterator.hasNext() &&!iterator.next().equals(str)) { iterator.next(); } return iterator.next(); }
InfoProcessorTest.java import java.util.*; /** * * Class to process and extract information from a list of lines. * */ public class InfoProcessorTest { /** * * List of lines to process. * */ private ArrayList lines =new ArrayList(); /** * * Creates InfoProcessor with given list of lines. * * @param lines to process * */ public InfoProcessorTest(ArrayList lines) { this.lines = lines; } /** * * Gets the course name from the list of lines. * * First, finds the line that starts with "Course:". * * Second, gets the String on the very next line, which should be the course * name. * * Third, returns the String from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the course name * * * * Example(s): * * - If the ArrayList lines contains: "Course:" and "CIT590", and we * call * * getCourseName(), we'll get "CIT590". * * * * - If the ArrayList lines contains: "Course:" and "CIT 593", and we * call * * getCourseName(), we'll get "CIT 593". * * * * @return course name * */ public String getCourseName() { return getNextStringStartsWith("Course"); } /** * * Gets the course ID from the list of lines. * * First, finds the line that starts with "CourseID:". * * Second, gets the String on the very next line, which should be the course ID. * * Third, casts the String to an int and returns it from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the course ID * * * * Example(s): * * - If the ArrayList lines contains: "CourseID:" and "590", and we call * * getCourseId(), we'll get the int 590. * * * * - If the ArrayList lines contains: "CourseID" and "593", and we call * * getCourseId(), we'll get the int 593. * * * * @return course ID * */ publicint getCourseId() { String courseId = getNextStringStartsWith("CourseID:"); int cid = Integer.parseInt(courseId); return cid; } /** * * Gets the student ID from the list of lines. * * First, finds the line that starts with "StudentID:". * * Second, gets the String on the very next line, which should be the student * ID. * * Third, casts the String to an int and returns it from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the student ID * * * * Example(s): * * - If the ArrayList lines contains: "StudentID:" and "101", and we * call * * getStudentId(), we'll get the int 101. * * * * - If the ArrayList lines contains: "StudentID" and "5554", and we * call * * getStudentId(), we'll get the int 5554. * * * * @return student ID * */ publicint getStudentId() { String studentId = getNextStringStartsWith("CourseID:"); int sid = Integer.parseInt(studentId); return sid; } /** * * To be used by "getCourseName", "getCourseId", and "getStudentId" methods. * * * * Gets the String that follows the line that starts with the given String. * * Gets and returns the String on the very next line. * * If the given String doesn't exist, should return null. * * * * Example(s): * * - If an ArrayList lines contains: "hello" and "world", and we call * * getNextStringStartsWith("hello"), we'll get the String "world". * * * * - If an ArrayList lines contains: "Course" and "CIT590", and we call * * getNextStringStartsWith("Course"), we'll get the String "CIT590". * * * * - If an ArrayList lines contains: "hello" and "world", and we call * * getNextStringStartsWith("goodbye"), we'll get null. * * * * @param str to look for in lines * * @return String from the very next line * */ String getNextStringStartsWith(String str) { Iterator iterator = lines.iterator(); while (iterator.hasNext() &&!iterator.next().equals(str)) { iterator.next(); } return iterator.next(); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
InfoProcessorTest.java
import java.util.*;
/**
*
* Class to process and extract information from a list of lines.
*
*/
public class InfoProcessorTest {
/**
*
* List of lines to process.
*
*/
private ArrayList<String> lines =new ArrayList<String>();
/**
*
* Creates InfoProcessor with given list of lines.
*
* @param lines to process
*
*/
public InfoProcessorTest(ArrayList<String> lines) {
this.lines = lines;
}
/**
*
* Gets the course name from the list of lines.
*
* First, finds the line that starts with "Course:".
*
* Second, gets the String on the very next line, which should be the course
* name.
*
* Third, returns the String from the method.
*
*
*
* Hint(s):
*
* - Use the getNextStringStartsWith(String str) method to find the course name
*
*
*
* Example(s):
*
* - If the ArrayList<String> lines contains: "Course:" and "CIT590", and we
* call
*
* getCourseName(), we'll get "CIT590".
*
*
*
* - If the ArrayList<String> lines contains: "Course:" and "CIT 593", and we
* call
*
* getCourseName(), we'll get "CIT 593".
*
*
*
* @return course name
*
*/
public String getCourseName() {
return getNextStringStartsWith("Course");
}
/**
*
* Gets the course ID from the list of lines.
*
* First, finds the line that starts with "CourseID:".
*
* Second, gets the String on the very next line, which should be the course ID.
*
* Third, casts the String to an int and returns it from the method.
*
*
*
* Hint(s):
*
* - Use the getNextStringStartsWith(String str) method to find the course ID
*
*
*
* Example(s):
*
* - If the ArrayList<String> lines contains: "CourseID:" and "590", and we call
*
* getCourseId(), we'll get the int 590.
*
*
*
* - If the ArrayList<String> lines contains: "CourseID" and "593", and we call
*
* getCourseId(), we'll get the int 593.
*
*
*
* @return course ID
*
*/
publicint getCourseId() {
String courseId = getNextStringStartsWith("CourseID:");
int cid = Integer.parseInt(courseId);
return cid;
}
/**
*
* Gets the student ID from the list of lines.
*
* First, finds the line that starts with "StudentID:".
*
* Second, gets the String on the very next line, which should be the student
* ID.
*
* Third, casts the String to an int and returns it from the method.
*
*
*
* Hint(s):
*
* - Use the getNextStringStartsWith(String str) method to find the student ID
*
*
*
* Example(s):
*
* - If the ArrayList<String> lines contains: "StudentID:" and "101", and we
* call
*
* getStudentId(), we'll get the int 101.
*
*
*
* - If the ArrayList<String> lines contains: "StudentID" and "5554", and we
* call
*
* getStudentId(), we'll get the int 5554.
*
*
*
* @return student ID
*
*/
publicint getStudentId() {
String studentId = getNextStringStartsWith("CourseID:");
int sid = Integer.parseInt(studentId);
return sid;
}
/**
*
* To be used by "getCourseName", "getCourseId", and "getStudentId" methods.
*
*
*
* Gets the String that follows the line that starts with the given String.
*
* Gets and returns the String on the very next line.
*
* If the given String doesn't exist, should return null.
*
*
*
* Example(s):
*
* - If an ArrayList<String> lines contains: "hello" and "world", and we call
*
* getNextStringStartsWith("hello"), we'll get the String "world".
*
*
*
* - If an ArrayList<String> lines contains: "Course" and "CIT590", and we call
*
* getNextStringStartsWith("Course"), we'll get the String "CIT590".
*
*
*
* - If an ArrayList<String> lines contains: "hello" and "world", and we call
*
* getNextStringStartsWith("goodbye"), we'll get null.
*
*
*
* @param str to look for in lines
*
* @return String from the very next line
*
*/
String getNextStringStartsWith(String str) {
Iterator<String> iterator = lines.iterator();
while (iterator.hasNext() &&!iterator.next().equals(str)) {
iterator.next();
}
return iterator.next();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
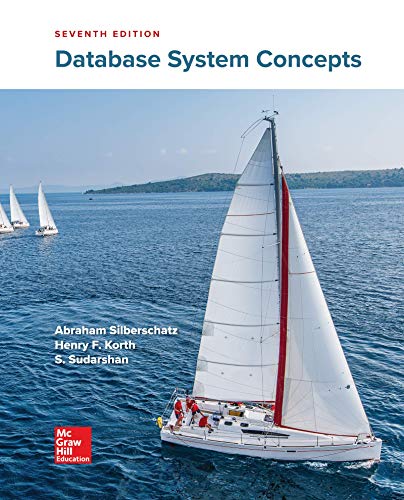
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
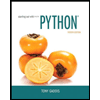
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
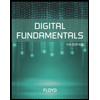
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
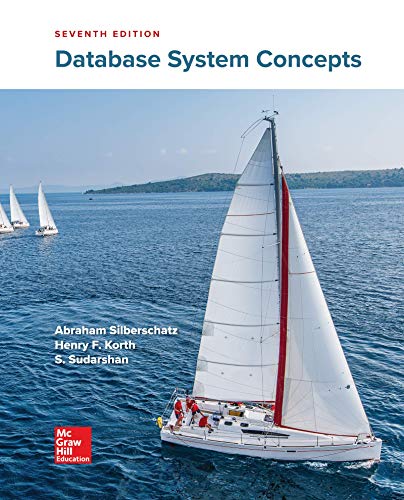
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
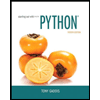
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
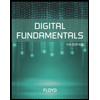
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
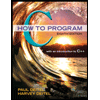
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
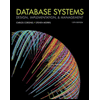
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
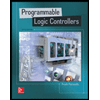
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education