import java.util.ArrayList; public class BasicArrayList { // main method public static void main(String[] args) { // create an arraylist of type Integer ArrayList basic = new ArrayList(); // using loop add elements for(int i=0;i<4;i++){ basic.add(i+1); } // using loop set elements for(int i=0;i
API documentation link ------> https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/ArrayList.html
PREVIOUS CODE -
import java.util.ArrayList;
public class BasicArrayList {
// main method
public static void main(String[] args) {
// create an arraylist of type Integer
ArrayList<Integer> basic = new ArrayList<Integer>();
// using loop add elements
for(int i=0;i<4;i++){
basic.add(i+1);
}
// using loop set elements
for(int i=0;i<basic.size();i++){
basic.set(i,(basic.get(i)*5));
}
// using loop print elements
for(int i=0;i<basic.size();i++){
System.out.println(basic.get(i));
}
}
}
Q1)
a)Consider how to remove elements from the ArrayList. Give two different lines of code that would both remove 5 from the ArrayList.
b)What do you think the contents of the ArrayList will look like after the 5 has been removed? (Sketch or list)
c)Now update the BasicArrayList program to remove the 5 (index 1) from the ArrayList. Add this step before the last loop that prints out the contents fo the ArrayList. Examine the output and compare it to your answer in the previous question? Were there any surprises (or bugs) in your code?
d) Now go back and examine the API documentation for the ArrayList class (Links to an external site.).
i) Give an example line of code, calling an ArrayList method that would help you search the ArrayList for a specific element.
ii) What will this method return if the element is not found?
e)Referring back to the API documentation for the ArrayList class (Links to an external site.),
i) Give an example line of code that calls an ArrayList method that would remove all elements from the ArrayList.
ii) Give an example line of code that calls an ArrayList method that would check if the ArrayList has no elements in it.
f)
Update your BasicArrayList program by adding the following:
- before the last for-loop, add a statement to set the element in position 0 to 1
- after the last for-loop add code to s
- search the ArrayList for the number 5 and display the index if found
- search the ArrayList for the number 15 and display the index if found
- check if the array is empty and print an appropriate message (e.g., "ArrayList is empty/not empty")
.
Recalling from the previous activity, the contents of the ArrayList after step 5 on the last question (where we converted the BasicArray program to BasicArrayList and a new element is added to position 0), are as follows:
| 0 | 5 | 10 | 15 | 20 |
|----|----|----|----|----|
In this table, each cell represents an element in the ArrayList at positions 0 through 4.
For further reading and more advanced methods, refer to the ArrayList API documentation linked above.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6cf78908-a1f9-4330-9ece-f4021df10613%2F2b96e1d7-f756-4c43-95c7-2b75f5a2514e%2F0ft8c0f_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

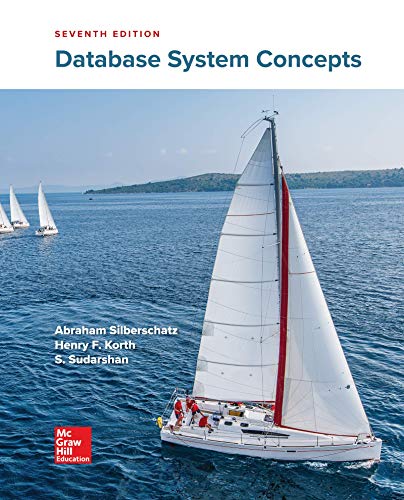
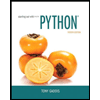
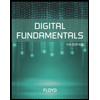
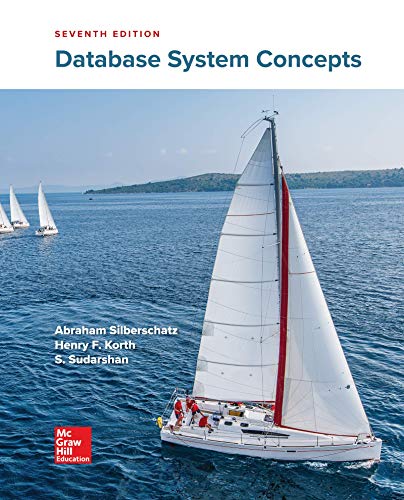
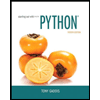
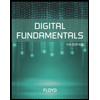
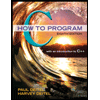
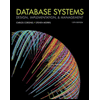
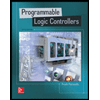