In Java Write a program that asks the user to enter how many automobiles are to be described, and for each automobile, it (the driver) inputs the user’s selection of make and color. Then the driver outputs color and make. Although you could do this easily using a simple procedural program, do it by using a main method in class AutomobileDriver to instantiate an object called auto from a class Automobile. Make your classes conform to the following UML class diagram: Let Automobile methods setMake and setColor do the prompting and inputting for make and color, and include input verification which asks again if a selection is illegal. Let Automobile methods printColor and printMake print color and make on one line. In AutomobileDriver, after asking auto to call setMake and setColor, use auto.printColor().printMake(); to chain the two printing methods. Write code so that the program can produce the following display: Sample session: How many cars do you want to consider? 2 Select Buick, Chevrolet, or Pontiac (b,c,p): x The only valid selections are 'b', 'c', or 'p' Select Buick, Chevrolet, or Pontiac (b,c,p): p Select blue, green, or red (b,g,r): r red Pontiac Select Buick, Chevrolet, or Pontiac (b,c,p): c Select blue, green, or red (b,g,r): g green Chevrolet The driver class: import java.util.Scanner; public class AutomobileDriver { public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); int n; // quantity of cars Automobile auto = new Automobile(); System.out.print( "How many cars do you want to consider? "); n = stdIn.nextInt(); for (int i=0; i
In Java
Write a program that asks the user to enter how many automobiles are to be
described, and for each automobile, it (the driver) inputs the user’s selection of
make and color. Then the driver outputs color and make. Although you could do
this easily using a simple procedural program, do it by using a main method in
class AutomobileDriver to instantiate an object called auto from a class
Automobile. Make your classes conform to the following UML class diagram:
Let Automobile methods setMake and setColor do the prompting and inputting
for make and color, and include input verification which asks again if a selection
is illegal. Let Automobile methods printColor and printMake print color and
make on one line. In AutomobileDriver, after asking auto to call setMake and
setColor, use auto.printColor().printMake(); to chain the two printing methods.
Write code so that the program can produce the following display:
Sample session:
How many cars do you want to consider? 2
Select Buick, Chevrolet, or Pontiac (b,c,p): x
The only valid selections are 'b', 'c', or 'p'
Select Buick, Chevrolet, or Pontiac (b,c,p): p
Select blue, green, or red (b,g,r): r
red Pontiac
Select Buick, Chevrolet, or Pontiac (b,c,p): c
Select blue, green, or red (b,g,r): g
green Chevrolet
The driver class:
import java.util.Scanner;
public class AutomobileDriver
{
public static void main(String[] args)
{
Scanner stdIn = new Scanner(System.in);
int n; // quantity of cars
Automobile auto = new Automobile();
System.out.print( "How many cars do you want to consider? ");
n = stdIn.nextInt();
for (int i=0; i<n; i++)
{
auto.setMake();
auto.setColor();
auto.printColor().printMake();
System.out.println();
} // end for
} // end main
} // end class AutomobileDriver

In order to write a solution for this problem in Java, we will need to create a main method in the AutomobileDriver class. This method will be responsible for instantiating an object called auto from the Automobile class.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

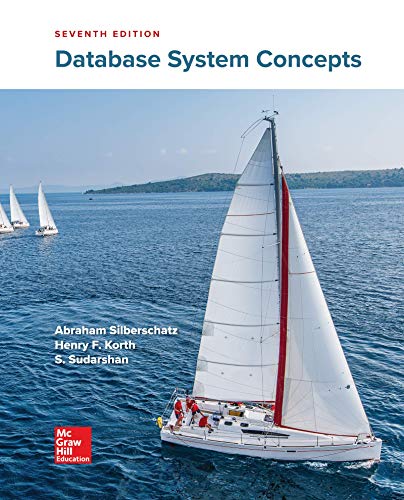
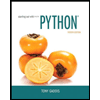
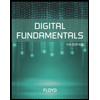
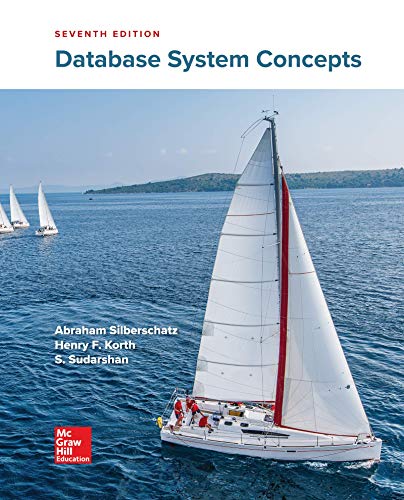
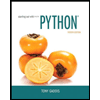
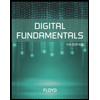
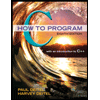
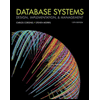
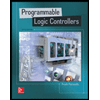