In data structure, I want to make code about printing this java tree codes in preorder Traversal and postorder Traversal. My Node code and Tree Code is here import java.util.ArrayList;
In data structure, I want to make code about printing this java tree codes in preorder Traversal and postorder Traversal. My Node code and Tree Code is here import java.util.ArrayList;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
In data structure, I want to make code about printing this java tree codes in preorder Traversal and postorder Traversal.
My Node code and Tree Code is here
import java.util.ArrayList;
public class MyNode {
private Object element;
private MyNode parent;
private ArrayList children;
public MyNode() {
this.element = null;
this.parent = null;
this.children = null;
}
public MyNode(Object e) {
this.element = e;
this.parent = null;
this.children = new ArrayList();
this.children.add(null);
this.children.add(null);
}
public Object element() {
return this.element;
}
public MyNode parent() {
return this.parent;
}
public ArrayList children() {
return this.children;
}
public int degree() {
return this.children.size();
}
public void setElement(Object e) {
this.element = e;
}
public void setParent(MyNode p) {
this.parent = p;
}
public void setChildren(ArrayList c) {
this.children = c;
}
}
import java.util.ArrayList;
public class MyTree {
private MyNode root;
private int totalSize;
public MyTree() {
this.root = null;
this.totalSize = 0;
}
public MyTree(Object e) {
this.root = new MyBinNode(e);
this.totalSize = 1;
}
public int size() {
return this.totalSize;
}
public MyNode root() {
return this.root;
}
public ArrayList children(MyNode v) {
return v.children();
}
public boolean isExternal(MyNode v) {
return v.children().isEmpty();
}
public MyNode addRoot(Object e) {
MyNode temp = this.root;
this.root = new MyBinNode(e);
this.totalSize = 1;
return temp;
}
public MyNode addNode(Object e) {
MyNode newNode = new MyBinNode(e);
newNode.setParent(this.root);
this.root.children().add(newNode);
this.totalSize++;
return newNode;
}
public MyNode addChild(MyNode v, Object e) {
MyNode newNode = new MyBinNode(e);
newNode.setParent(v);
v.children().add(newNode);
this.totalSize++;
return newNode;
}
public MyNode addChild(MyNode v, int i, Object e) {
MyNode newNode = new MyBinNode(e);
newNode.setParent(v);
v.children().add(i, newNode);
this.totalSize++;
return newNode;
}
public MyNode setChild(MyNode v, int i, Object e) {
MyNode newNode = new MyBinNode(e);
newNode.setParent(v);
v.children().set(i, newNode);
return newNode;
}
public MyNode removeChild(MyNode v, int i) {
this.totalSize--;
return (MyNode)v.children().remove(i);
}
}
![[숙제] 앞에서 구현한 MyTree 클래스를 이용하여 다음의
문제를 Java로 구현하시오
1) 아래 그림과 같은 구조의 Tree를 생성하고 Preorder Traversal을 이용하여 오
른쪽 그림과 같이 출력하는 Java 프로그램을 작성하시오
※ 줄간 Indentation 주의
Make Money Fast!
Make Money Fast
1. Motivation
화면
출력
1.1 Greed
2. Methods
1.2 Avidity
1. Motivations
References
2. Methods
2.1 Stock F'raud
1.2 Avidity
2.1 Stock
Fraud
2.2 Ponzi
Scheme
2.3 Bank
Robbery
1.1 Greed
2) 아래 그림과 같은 구조의 Tree를 생성하고 Postorder Traversal을 이용하여
오른쪽 그림과 같이 출력하는 Java 프로그램을 작성하시오
※각 Node element는 파일명과
크기를 모두 포함
cs16/
화면
출력
todo,txt
homeworks/ = 5KB
homeworks/
programs/
(1K)
programs/ = 55KB
cs16/ = 61KB
hic.doc
(3K)
hinc.doc
(2K)
DDR.java
(10K)
Stocks. java
(25K)
Robot.java
(20K)
5](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2080659c-822e-4785-8970-9b3188b7725e%2Fdba8104f-bd40-4806-ba91-b44aed7aee62%2Fnt9tuit_processed.png&w=3840&q=75)
Transcribed Image Text:[숙제] 앞에서 구현한 MyTree 클래스를 이용하여 다음의
문제를 Java로 구현하시오
1) 아래 그림과 같은 구조의 Tree를 생성하고 Preorder Traversal을 이용하여 오
른쪽 그림과 같이 출력하는 Java 프로그램을 작성하시오
※ 줄간 Indentation 주의
Make Money Fast!
Make Money Fast
1. Motivation
화면
출력
1.1 Greed
2. Methods
1.2 Avidity
1. Motivations
References
2. Methods
2.1 Stock F'raud
1.2 Avidity
2.1 Stock
Fraud
2.2 Ponzi
Scheme
2.3 Bank
Robbery
1.1 Greed
2) 아래 그림과 같은 구조의 Tree를 생성하고 Postorder Traversal을 이용하여
오른쪽 그림과 같이 출력하는 Java 프로그램을 작성하시오
※각 Node element는 파일명과
크기를 모두 포함
cs16/
화면
출력
todo,txt
homeworks/ = 5KB
homeworks/
programs/
(1K)
programs/ = 55KB
cs16/ = 61KB
hic.doc
(3K)
hinc.doc
(2K)
DDR.java
(10K)
Stocks. java
(25K)
Robot.java
(20K)
5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
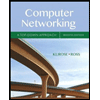
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
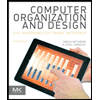
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
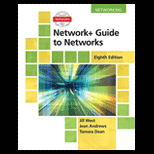
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
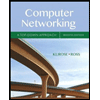
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
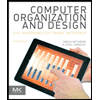
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
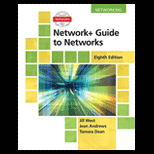
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
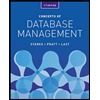
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
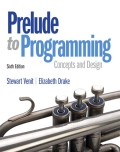
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
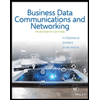
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY