in C# i need to Add an operator*() method to the Fraction class created in Programming Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2. my errors are in the pictures my code is public class Fraction { private int numerator; private int denominator; public Fraction(int num, int denom) { numerator = num; denominator = denom; } // Define the * operator for Fraction objects public static Fraction operator *(Fraction a, Fraction b) { int num = a.numerator * b.numerator; int denom = a.denominator * b.denominator; return new Fraction(num, denom).Reduce(); } // Reduce the fraction to its lowest terms public Fraction Reduce() { int gcd = GCD(numerator, denominator); numerator /= gcd; denominator /= gcd; return this; } // Calculate the greatest common divisor private int GCD(int a, int b) { if (b == 0) return a; return GCD(b, a % b); } } class FractionDemo2 { static void Main() { // Create two Fractions Fraction a = new Fraction(2, 3); Fraction b = new Fraction(3, 4); // Multiply the two Fractions Fraction result = a * b; // Display the result Console.WriteLine("{0} * {1} = {2}", a, b, result); } }
in C# i need to Add an operator*() method to the Fraction class created in Programming Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2. my errors are in the pictures my code is public class Fraction { private int numerator; private int denominator; public Fraction(int num, int denom) { numerator = num; denominator = denom; } // Define the * operator for Fraction objects public static Fraction operator *(Fraction a, Fraction b) { int num = a.numerator * b.numerator; int denom = a.denominator * b.denominator; return new Fraction(num, denom).Reduce(); } // Reduce the fraction to its lowest terms public Fraction Reduce() { int gcd = GCD(numerator, denominator); numerator /= gcd; denominator /= gcd; return this; } // Calculate the greatest common divisor private int GCD(int a, int b) { if (b == 0) return a; return GCD(b, a % b); } } class FractionDemo2 { static void Main() { // Create two Fractions Fraction a = new Fraction(2, 3); Fraction b = new Fraction(3, 4); // Multiply the two Fractions Fraction result = a * b; // Display the result Console.WriteLine("{0} * {1} = {2}", a, b, result); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
in C# i need to
Add an operator*() method to the Fraction class created in Programming Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2.
my errors are in the pictures
my code is
public class Fraction
{
private int numerator;
private int denominator;
public Fraction(int num, int denom)
{
numerator = num;
denominator = denom;
}
// Define the * operator for Fraction objects
public static Fraction operator *(Fraction a, Fraction b)
{
int num = a.numerator * b.numerator;
int denom = a.denominator * b.denominator;
return new Fraction(num, denom).Reduce();
}
// Reduce the fraction to its lowest terms
public Fraction Reduce()
{
int gcd = GCD(numerator, denominator);
numerator /= gcd;
denominator /= gcd;
return this;
}
// Calculate the greatest common divisor
private int GCD(int a, int b)
{
if (b == 0)
return a;
return GCD(b, a % b);
}
}
class FractionDemo2
{
static void Main()
{
// Create two Fractions
Fraction a = new Fraction(2, 3);
Fraction b = new Fraction(3, 4);
// Multiply the two Fractions
Fraction result = a * b;
// Display the result
Console.WriteLine("{0} * {1} = {2}", a, b, result);
}
}
![=1
39
26
70
24
34
75 Fraction's operator () multiplication test 3
76
77
32
24
25
14
15
22
27
29
30
public class FractionClassMultiply Test
€
42
[Test]
Ⓒreferences
public void FractionfultiplyTest2()
[
45
Fraction f1 = new Fraction (2, 0, 1);
Fraction f2 = new Fraction (4, 1, 8);
Fraction res
res - f2. f1;
Assert.Ar Equal(B, ze.Wholeum);
Assert.Ar Equal (1, res.Numerator);
Assert.Ar Equal (4, res.Denominator)
1
Unit TestIncomplete
Build Status
Build Failed
Build Output
Compilation failed; & error(s), 0 warnings
FractionDemo2.cs(54,9): error (50103: The name Console does not exist in the current context
NtTestlalee16.ca(12, 19): error C51729: The type Fraction does not contain a constructor that takes '3' arguments
FractionCwmo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlalee16.ca(13, 19); error C51729: The type Fraction does not contain a constructor that takes 3 arguments
Fraction@wmo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlalee15.cs(18, 28): error C51061: Type "Fraction does not contain a definition for 'Wholellum' and no extension method "Wholelium of type 'Fraction could be found. Are you missing an assembly reference?
to previous error)
Fractions2.es(1,14): (Location of the symbol related
NtTestlalee15.ca(19, 28); error C51061: Type Fraction does not contain a definition for "Numerator and no extension method Numerator of type 'Fraction could be found. Are you missing an assembly reference?
Fraction@wmo2.cs(1,14): (Location of the symbol related to previous error)
NtTestlalee15.cs(20, 28); error C51061: Type Fraction does not contain a definition for "Denominator and no extension method Denominator of type 'Fraction could be found. Are you missing an assembly reference?
FractionCwmo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
public class FractionClassMultiply Test
[Test]
Ⓒreferences
public void Fractionfultiply Test2()
{
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
20 NtTestd35c50a5.ca(13,19): error C51729: The type "Fraction does not contain a constructor that takes '3' arguments
Fraction f1 = new Fraction (1, 0, 1);
Fraction f2 = new Fraction (4, 0, 1);
Fraction res
res - f2f1;
Assert.Ar Equal(4, s.Wholeum);
Assert.Ar Equal(0, res.Numerator);
}
Unit Testincomplete
Fraction's operator() multiplication test
Build Status
Build Failed
Build Output
Compilation failed: 5 error(s), 0 warnings
O referenc
FractionDemo2.cs(54,9): error C50103: The name Console does not exist in the current context
NtTest635c50a5.cs(12, 19): error CS1729: The type 'Fraction does not contain a constructor that takes '3' arguments
DIMENSION
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTestd35c50a5.ca(18,28): error c51061; Type Fraction does not contain a definition for 'WholeNum' and no extension method 'Wholellum' of type "Fraction could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTesta35c50a5.ca(19,30): error c51061: Type 'Fraction does not contain a definition for 'FracString' and no extension method "FracString of type 'Fraction could be found. Are you missing an assembly reference?
FractionCwmo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
public class Fraction ClassMultiply Test
{
public void FractionfultiplyTest2()
Fraction f1 = new Fraction (0, 1, 1);
Fraction f2= new Fraction(0, 1, 1);
Fraction res;
res f2f1;
Assert. Arequal (1, s.WholeNum);
Assert.Aretqual("1", res.Fraestring());
}
Assert. Arequal(1, res.Denominator);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda2a136f-d248-470a-adf7-afa3bf81602c%2Fd03e1f49-f69e-4fc5-9b9f-6927d8647dd3%2Fyy9s81n_processed.png&w=3840&q=75)
Transcribed Image Text:=1
39
26
70
24
34
75 Fraction's operator () multiplication test 3
76
77
32
24
25
14
15
22
27
29
30
public class FractionClassMultiply Test
€
42
[Test]
Ⓒreferences
public void FractionfultiplyTest2()
[
45
Fraction f1 = new Fraction (2, 0, 1);
Fraction f2 = new Fraction (4, 1, 8);
Fraction res
res - f2. f1;
Assert.Ar Equal(B, ze.Wholeum);
Assert.Ar Equal (1, res.Numerator);
Assert.Ar Equal (4, res.Denominator)
1
Unit TestIncomplete
Build Status
Build Failed
Build Output
Compilation failed; & error(s), 0 warnings
FractionDemo2.cs(54,9): error (50103: The name Console does not exist in the current context
NtTestlalee16.ca(12, 19): error C51729: The type Fraction does not contain a constructor that takes '3' arguments
FractionCwmo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlalee16.ca(13, 19); error C51729: The type Fraction does not contain a constructor that takes 3 arguments
Fraction@wmo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlalee15.cs(18, 28): error C51061: Type "Fraction does not contain a definition for 'Wholellum' and no extension method "Wholelium of type 'Fraction could be found. Are you missing an assembly reference?
to previous error)
Fractions2.es(1,14): (Location of the symbol related
NtTestlalee15.ca(19, 28); error C51061: Type Fraction does not contain a definition for "Numerator and no extension method Numerator of type 'Fraction could be found. Are you missing an assembly reference?
Fraction@wmo2.cs(1,14): (Location of the symbol related to previous error)
NtTestlalee15.cs(20, 28); error C51061: Type Fraction does not contain a definition for "Denominator and no extension method Denominator of type 'Fraction could be found. Are you missing an assembly reference?
FractionCwmo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
public class FractionClassMultiply Test
[Test]
Ⓒreferences
public void Fractionfultiply Test2()
{
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
20 NtTestd35c50a5.ca(13,19): error C51729: The type "Fraction does not contain a constructor that takes '3' arguments
Fraction f1 = new Fraction (1, 0, 1);
Fraction f2 = new Fraction (4, 0, 1);
Fraction res
res - f2f1;
Assert.Ar Equal(4, s.Wholeum);
Assert.Ar Equal(0, res.Numerator);
}
Unit Testincomplete
Fraction's operator() multiplication test
Build Status
Build Failed
Build Output
Compilation failed: 5 error(s), 0 warnings
O referenc
FractionDemo2.cs(54,9): error C50103: The name Console does not exist in the current context
NtTest635c50a5.cs(12, 19): error CS1729: The type 'Fraction does not contain a constructor that takes '3' arguments
DIMENSION
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTestd35c50a5.ca(18,28): error c51061; Type Fraction does not contain a definition for 'WholeNum' and no extension method 'Wholellum' of type "Fraction could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTesta35c50a5.ca(19,30): error c51061: Type 'Fraction does not contain a definition for 'FracString' and no extension method "FracString of type 'Fraction could be found. Are you missing an assembly reference?
FractionCwmo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
public class Fraction ClassMultiply Test
{
public void FractionfultiplyTest2()
Fraction f1 = new Fraction (0, 1, 1);
Fraction f2= new Fraction(0, 1, 1);
Fraction res;
res f2f1;
Assert. Arequal (1, s.WholeNum);
Assert.Aretqual("1", res.Fraestring());
}
Assert. Arequal(1, res.Denominator);
![The Fraction class operator *() method defined for Fraction class
O references
e out of 4 checks passed. Review the results below for more details.
Checks
Unit Test
Incomplete
Fraction's operator+* () multiplication test 1
Build Status
Build Failed
Build Output,
Compilation failed: 6 error(s), @ warnings
FractionDemo2.cs (54,9): error CS0103: The name 'Console' does not exist in the current context
NtTestlef5453f.cs(12,19): error CS1729: The type 'Fraction does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlef5453f.cs(13, 19): error CS1729: The type 'Fraction' does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlef5453f.cs (18, 29): error CS1061: Type Fraction does not contain a definition for 'WholeNum' and no extension method 'WholeNum' of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTestlef5453f.cs (19, 28): error CS1061: Type Fraction does not contain a definition for 'Numerator and no extension method Numerator of type 'Fraction could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTestlef5453f.cs(20, 28): error CS1061: Type Fraction does not contain a definition for 'Denominator' and no extension method 'Denominator of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
3 references
public class Fraction ClassMultiply Test
{
[Test]
0
O references
public void FractionMultiplyTest()
{
}
Fraction f1= new Fraction (5, 3, 9);
Fraction f2= new Fraction(16, 2, 7);
Fraction res;
res = f1 + f2;
Assert. AreEqual(86, res.WholeNum);
Assert. AreEqual(6, res.Numerator);
Assert. AreEqual(7, res. Denominator);
}
Unit TestIncomplete
Fraction's operator* () multiplication test 2
Build Status
Build Failed
Build Output
Compilation failed: 6 error(s), 0 warnings
O references
FractionDemo2.cs (54,9): error CS0103: The name Console' does not exist in the current context
NtTest4713646f.cs(13,19): error CS1729: The type 'Fraction does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTest4713646f.cs(14, 19): error CS1729: The type 'Fraction' does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTest4713646f.cs(19, 28): error CS1061: Type Fraction does not contain a definition for 'WholeNum' and no extension method 'WholeNum' of type 'Fraction could be found. Are you missing an assembly reference?
FractionDemo2.cs (1,14): (Location of the symbol related to previous error)
NtTest4713646f.cs(20, 28): error CS1061: Type Fraction does not contain a definition for 'Numerator and no extension method Numerator of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTest4713646f.cs(21, 28): error CS1061: Type Fraction does not contain a definition for 'Denominator' and no extension method 'Denominator of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda2a136f-d248-470a-adf7-afa3bf81602c%2Fd03e1f49-f69e-4fc5-9b9f-6927d8647dd3%2Fny9p5fo_processed.png&w=3840&q=75)
Transcribed Image Text:The Fraction class operator *() method defined for Fraction class
O references
e out of 4 checks passed. Review the results below for more details.
Checks
Unit Test
Incomplete
Fraction's operator+* () multiplication test 1
Build Status
Build Failed
Build Output,
Compilation failed: 6 error(s), @ warnings
FractionDemo2.cs (54,9): error CS0103: The name 'Console' does not exist in the current context
NtTestlef5453f.cs(12,19): error CS1729: The type 'Fraction does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlef5453f.cs(13, 19): error CS1729: The type 'Fraction' does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTestlef5453f.cs (18, 29): error CS1061: Type Fraction does not contain a definition for 'WholeNum' and no extension method 'WholeNum' of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTestlef5453f.cs (19, 28): error CS1061: Type Fraction does not contain a definition for 'Numerator and no extension method Numerator of type 'Fraction could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTestlef5453f.cs(20, 28): error CS1061: Type Fraction does not contain a definition for 'Denominator' and no extension method 'Denominator of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
3 references
public class Fraction ClassMultiply Test
{
[Test]
0
O references
public void FractionMultiplyTest()
{
}
Fraction f1= new Fraction (5, 3, 9);
Fraction f2= new Fraction(16, 2, 7);
Fraction res;
res = f1 + f2;
Assert. AreEqual(86, res.WholeNum);
Assert. AreEqual(6, res.Numerator);
Assert. AreEqual(7, res. Denominator);
}
Unit TestIncomplete
Fraction's operator* () multiplication test 2
Build Status
Build Failed
Build Output
Compilation failed: 6 error(s), 0 warnings
O references
FractionDemo2.cs (54,9): error CS0103: The name Console' does not exist in the current context
NtTest4713646f.cs(13,19): error CS1729: The type 'Fraction does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTest4713646f.cs(14, 19): error CS1729: The type 'Fraction' does not contain a constructor that takes '3' arguments
FractionDemo2.cs(6,12): (Location of the symbol related to previous error)
NtTest4713646f.cs(19, 28): error CS1061: Type Fraction does not contain a definition for 'WholeNum' and no extension method 'WholeNum' of type 'Fraction could be found. Are you missing an assembly reference?
FractionDemo2.cs (1,14): (Location of the symbol related to previous error)
NtTest4713646f.cs(20, 28): error CS1061: Type Fraction does not contain a definition for 'Numerator and no extension method Numerator of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
NtTest4713646f.cs(21, 28): error CS1061: Type Fraction does not contain a definition for 'Denominator' and no extension method 'Denominator of type 'Fraction' could be found. Are you missing an assembly reference?
FractionDemo2.cs(1,14): (Location of the symbol related to previous error)
Test Contents
[TestFixture]
Expert Solution

Step 1
The correct code is given below with output screenshot
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
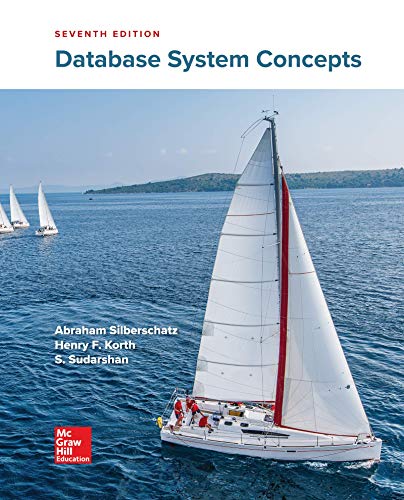
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
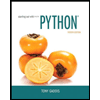
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
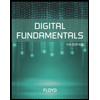
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
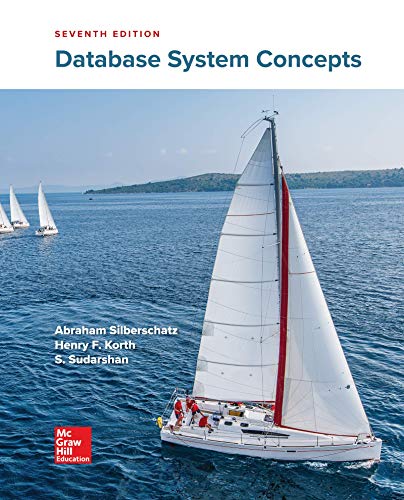
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
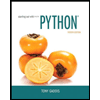
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
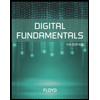
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
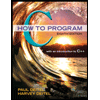
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
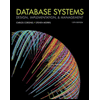
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
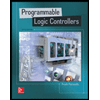
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education