Example: Object arg = "Kean"; System.out.println(arg); arg = 1234; System.out.println(arg); arg = true; System.out.println(arg); Output Kean 1234 true The method called as "RecursiveMethod" takes two Object type arguments. This method should return either True or False if the two arguments belong to same data type and also have the same value. Sample Run: public static void main(String[] args) { System.out.println (Recursion Problem (12,12)); System.out.println (Recursion Problem (245, "245")); System.out.println(Recursion Problem (true,false)); System.out.println (RecursionProblem("Kean", "Kean")); System.out.println (Recursion Problem ("KEAN", "Kean")); System.out.println(RecursionProblem("K", 'K')); System.out.println (Recursion Problem (340.6,340.6)); } Output: true false false true false false true
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
![## Example Code and Explanation
### Code Example:
```java
Object arg = "Kean";
System.out.println(arg);
arg = 1234;
System.out.println(arg);
arg = true;
System.out.println(arg);
```
### Output:
```
Kean
1234
true
```
### Explanation:
- The `Object` type can store different data types, demonstrating polymorphism in Java.
- This example initializes `arg` with a `String`, then an `int`, and finally a `boolean`, printing each value.
---
## Method Explanation
### Method: RecursiveMethod
- Takes two `Object` type arguments.
- Returns `True` if both arguments are of the same data type and contain the same value; otherwise, it returns `False`.
---
## Sample Run
### Sample Code:
```java
public static void main(String[] args)
{
System.out.println(RecursionProblem(12, 12));
System.out.println(RecursionProblem(245, "245"));
System.out.println(RecursionProblem(true, false));
System.out.println(RecursionProblem("Kean", "Kean"));
System.out.println(RecursionProblem("KEAN", "Kean"));
System.out.println(RecursionProblem("K", 'K'));
System.out.println(RecursionProblem(340.6, 340.6));
}
```
### Output:
```
true
false
false
true
false
false
true
```
### Explanation:
- The `RecursionProblem` method evaluates the equality and data type of its two arguments.
- `RecursionProblem(12, 12)` returns `true` because both are integers with the same value.
- `RecursionProblem(245, "245")` returns `false` because they are of different data types (`int` vs. `String`).
- `RecursionProblem(true, false)` returns `false` because the boolean values differ.
- `RecursionProblem("Kean", "Kean")` returns `true` since both Strings are identical.
- `RecursionProblem("KEAN", "Kean")` returns `false` due to case sensitivity.
- `RecursionProblem("K", 'K')` returns `false` because one is a `String` and the other is a `char`.
- `RecursionProblem(340.6,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb64c1c6f-d4d7-4d8b-8ebe-5eadb49feddc%2F88d705aa-85a1-4e0b-aebc-d7edf264d9ed%2Fnzwy8le_processed.png&w=3840&q=75)


For the given problem, below is the Java code.
Step by step
Solved in 4 steps with 2 images

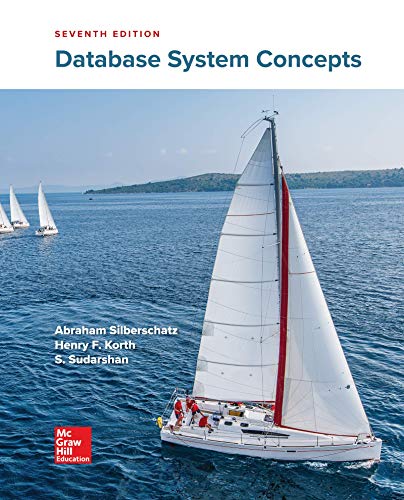
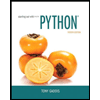
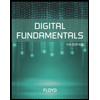
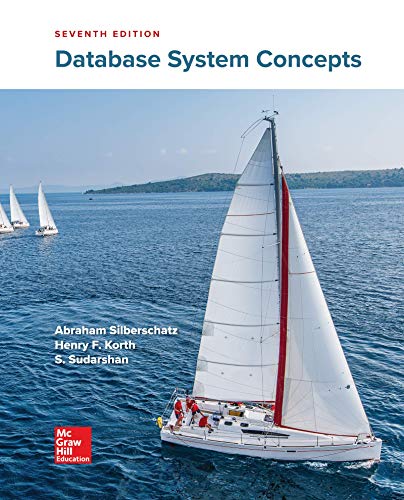
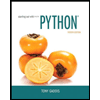
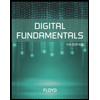
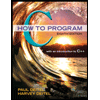
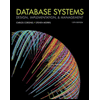
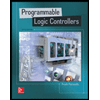