import java.util.concurrent.Semaphore; public class ReadWriteLock { private int count = 0; public void readLock() throws InterruptedException { readSemaphore.acquire(); if(count == 0){ writeSemaphore.acquire(); } count=count+1; readSemaphore.release(); } public void readUnLock() throws InterruptedException { readSemaphore.acquire(); count=count-1; if(count == 0){ writeSemaphore.release(); } readSemaphore.release(); } public void writeLock() throws InterruptedException { writeSemaphore.acquire(); } public void writeUnlock(){ writeSemaphore.release(); } } Test code, not rigorous enough, just have a look import org.junit.Test; public class ReadWriteLockTest { String test = “In Thread 1”; @Test public void testRead() throws Exception { ReadWriteLock readWriteLock = new ReadWriteLock(); Thread thread1 = new Thread(); try { readWriteLock.readLock(); System.out.println(test); Thread.sleep(6000); readWriteLock.readUnLock(); } catch (Exception e) { e.printStackTrace(); } Thread thread2 = new Thread(); try { readWriteLock.readLock(); test=”In Thread 2”; System.out.println(test); Thread.sleep(4000); readWriteLock.readUnLock(); } catch (Exception e) { e.printStackTrace(); } Thread t3 = new Thread(); try { readWriteLock.writeLock(); test =”In Thread 3”; System.out.println(test); readWriteLock.writeUnlock(); } catch (Exception e) { e.printStackTrace(); } thread1.start(); thread2.start(); thread3.start(); thread3.join(); } @Test public void testWrite() throws Exception { ReadWriteLock readWriteLock = new ReadWriteLock(); Thread thread1 = new Thread(); try { readWriteLock.readLock(); System.out.println(test); readWriteLock.readUnLock(); } catch (Exception e) { e.printStackTrace(); } Thread thread2 = new Thread(); try { readWriteLock.readLock(); System.out.println(test); readWriteLock.readUnLock(); } catch (InterruptedException e) { e.printStackTrace(); } Thread thread3 = new Thread(); try { readWriteLock.writeLock(); test = “In Write Thread 3; System.out.println(test); Thread.sleep(2000); readWriteLock.writeUnlock(); } catch (InterruptedException e) { e.printStackTrace(); } thread3.start(); thread1.start(); thread2.start(); thread2.join(); } } -Can you write the report describing this code?
import java.util.concurrent.Semaphore;
public class ReadWriteLock {
private int count = 0;
public void readLock() throws InterruptedException {
readSemaphore.acquire();
if(count == 0){
writeSemaphore.acquire();
}
count=count+1;
readSemaphore.release();
}
public void readUnLock() throws InterruptedException {
readSemaphore.acquire();
count=count-1;
if(count == 0){
writeSemaphore.release();
}
readSemaphore.release();
}
public void writeLock() throws InterruptedException {
writeSemaphore.acquire();
}
public void writeUnlock(){
writeSemaphore.release();
}
}
Test code, not rigorous enough, just have a look
import org.junit.Test;
public class ReadWriteLockTest {
String test = “In Thread 1”;
@Test
public void testRead() throws Exception {
ReadWriteLock readWriteLock = new ReadWriteLock();
Thread thread1 = new Thread();
try {
readWriteLock.readLock();
System.out.println(test);
Thread.sleep(6000);
readWriteLock.readUnLock();
} catch (Exception e) {
e.printStackTrace();
}
Thread thread2 = new Thread();
try {
readWriteLock.readLock();
test=”In Thread 2”;
System.out.println(test);
Thread.sleep(4000);
readWriteLock.readUnLock();
} catch (Exception e) {
e.printStackTrace();
}
Thread t3 = new Thread();
try {
readWriteLock.writeLock();
test =”In Thread 3”;
System.out.println(test);
readWriteLock.writeUnlock();
} catch (Exception e) {
e.printStackTrace();
}
thread1.start();
thread2.start();
thread3.start();
thread3.join();
}
@Test
public void testWrite() throws Exception {
ReadWriteLock readWriteLock = new ReadWriteLock();
Thread thread1 = new Thread();
try {
readWriteLock.readLock();
System.out.println(test);
readWriteLock.readUnLock();
} catch (Exception e) {
e.printStackTrace();
}
Thread thread2 = new Thread();
try {
readWriteLock.readLock();
System.out.println(test);
readWriteLock.readUnLock();
} catch (InterruptedException e) {
e.printStackTrace();
}
Thread thread3 = new Thread();
try {
readWriteLock.writeLock();
test = “In Write Thread 3;
System.out.println(test);
Thread.sleep(2000);
readWriteLock.writeUnlock();
} catch (InterruptedException e) {
e.printStackTrace();
}
thread3.start();
thread1.start();
thread2.start();
thread2.join();
}
}
-Can you write the report describing this code?

Step by step
Solved in 2 steps

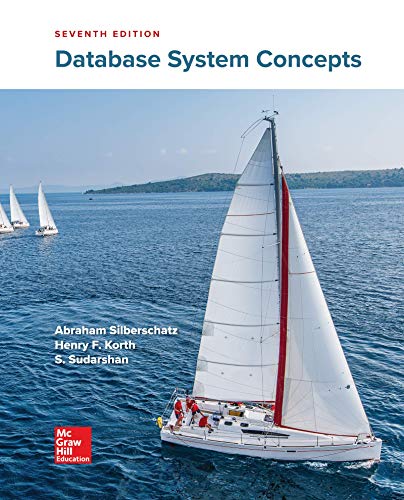
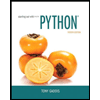
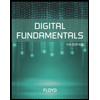
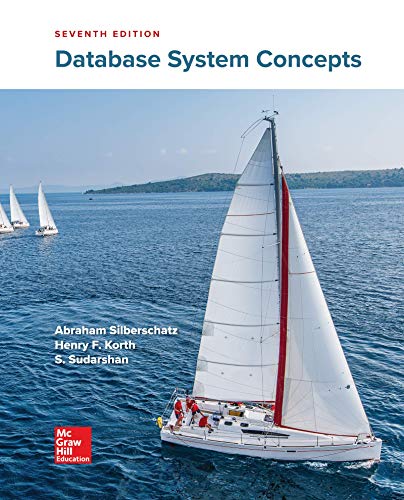
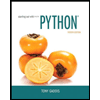
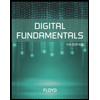
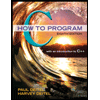
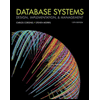
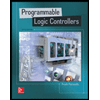