import java.io.File; import java.util.ArrayList; import java.util.Scanner; public class Assignment08 { public static void main(String[] args) throws Exception { File inputData = new File("input05.txt"); Scanner input = new Scanner(inputData); int totalNumber = input.nextInt(); Friend.friends = new Friend[totalNumber]; input.nextLine();
import java.io.File; import java.util.ArrayList; import java.util.Scanner; public class Assignment08 { public static void main(String[] args) throws Exception { File inputData = new File("input05.txt"); Scanner input = new Scanner(inputData); int totalNumber = input.nextInt(); Friend.friends = new Friend[totalNumber]; input.nextLine();
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
How do I match the output?
Code:
import java.io.File;
import java.util.ArrayList;
import java.util.Scanner;
public class Assignment08
{
public static void main(String[] args) throws Exception
{
File inputData = new File("input05.txt");
Scanner input = new Scanner(inputData);
int totalNumber = input.nextInt();
Friend.friends = new Friend[totalNumber];
input.nextLine();
for (int i = 0; i < totalNumber; i++)
{
String name = input.nextLine();
String phone = input.nextLine();
String age = input.nextLine();
int ageNum = Integer.parseInt(age);
Friend.friends[i] = new Friend(name, phone, ageNum);
}
printContacts(Friend.friends);
Friend.showTotalFriendAge();
ManageDuplicate(Friend.friends);
printContacts(Friend.friends);
Friend.showTotalFriendAge();
}
public static void printContacts(Friend[] contacts)
{
System.out.println("Contacts (" + contacts.length + ")");
System.out.println("===================");
for (Friend friend : contacts)
{
System.out.println(friend);
}
System.out.println();
}
public static void ManageDuplicate(Friend[] contacts)
{
ArrayList<Friend> duplicates = new ArrayList<>();
for (int i = 0; i < contacts.length - 1; i++)
{
for (int j = i + 1; j < contacts.length; j++)
{
if (contacts[i].equals(contacts[j]))
{
duplicates.add(contacts[i]);
}
}
}
if (!duplicates.isEmpty())
{
System.out.println("Duplicate contact detected:");
for (int i = 0; i < duplicates.size(); i++)
{
System.out.println("(" + i + ")" + duplicates.get(i).getName());
}
System.out.println();
// Modify duplicate entries and set age to 0
for (Friend duplicate : duplicates)
{
duplicate.setAge(0);
}
}
}
}
public class Friend
{
private String name;
private String phone;
private int age;
public static Friend[] friends; //array
public String toString()
{
return String.format("Name: %-20sPhone: %-15sAge: %d", name, phone, age);
}
public Friend(String name)
{
this.name = name;
}
public Friend(String name, String phone)
{
this.name = name;
this.phone = phone;
}
public Friend(String name, String phone, int age)
{
this.age = age;
this.name = name;
this.phone = phone;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public boolean equals(Friend dest)
{
if(this.name.equals(dest.name) && this.phone.equals(dest.phone) && this.age == dest.age)
{
return true;
}
else
{
return false;
}
}
public static void showTotalFriendAge()
{
int totalAge = 0;
System.out.print("Total Friends Age:(");
for(int i = 0; i < friends.length; i++)
{
if(i < friends.length - 1)
{
System.out.print(friends[i].age + ",");
}
else
{
System.out.print(friends[i].age + "");
}
totalAge += friends[i].age;
}
System.out.print(") Total: " + totalAge);
}
public static int getNumFriends()
{
return 0;
}
}

Transcribed Image Text:Contacts (12)
====
Name: Albert Zoe
Name: Albert Zoe
Name: Cat Xiver
Name: Dan Woow
Name: Esther Uno
Name: Frankie Uion
Name: Albert Zoe
Name: Gab Xvy
Name: Henrik Que
Name: Albert Zoe
Name: Henrik Que
Name: Henrik Que
Total Friends Age: (10,44,20,2,16,10,10,12,11,10,11,12) Total: 168
Duplicate contact detected: (0)Albert Zoe and (6) Albert Zoe
Duplicate contact detected: (0)Albert Zoe and (9)Albert Zoe
Duplicate contact detected: (8)Henrik Que and (10) Henrik Que
Contacts (12)
========
======
Phone: 8581234567
Phone: 8581234567
Phone: 6193456789
Phone: 8584567891
Phone: 8584567890
Phone: 4294567123
Phone: 8581234567
Phone: 6184567234
Phone: 7604567345
Phone: 8581234567
Phone: 7604567345
Phone: 7604567345
Age: 10
Age: 44
Age: 20
Age: 2
Age: 16
Age: 10
Age: 10
Age: 12
Age: 11
Age: 10
Age: 11
Age: 12
Name: Albert Zoe
Name: Albert Zoe
Name: Cat Xiver
Name: Dan Woow
Name: Esther Uno
Name: Frankie Uion
Name: Albert Zoe (1)
Name: Gab Xvy
Name: Henrik Que
Name: Albert Zoe(2)
Name: Henrik Que(3)
Name: Henrik Que
Total Friends Age: (44,20,2,16,10,12,10,11,12,0,0,0) Total: 137
Phone: 8581234567
Phone: 8581234567
Phone: 6193456789
Phone: 8584567891
Phone: 8584567890
Phone: 4294567123
Phone: 8581234567(1)
Phone: 6184567234
Phone: 7604567345
Phone:
Age: 11
8581234567(2) Age: 0
Phone: 7604567345(3) Age: 0
Phone: 7604567345
Age: 12
Age: 10
Age: 44
Age: 20
Age: 2
Age: 16
Age: 10
Age: 0
Age: 12
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
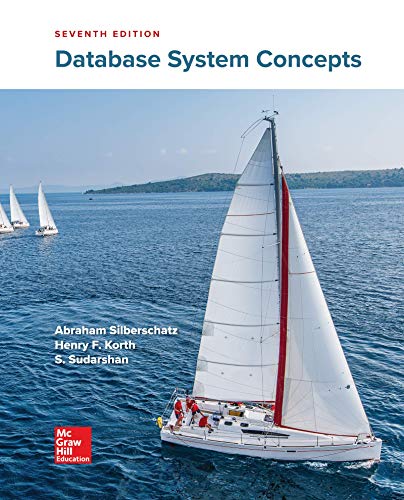
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
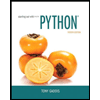
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
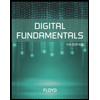
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
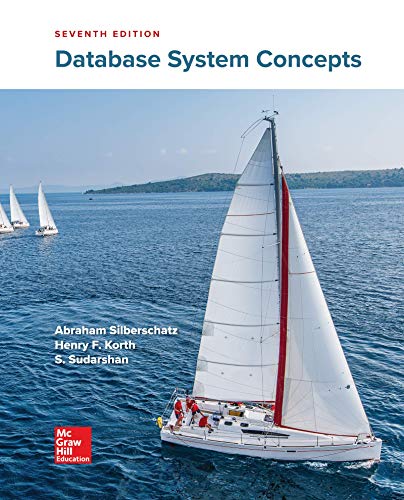
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
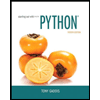
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
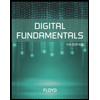
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
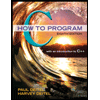
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
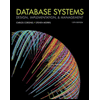
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
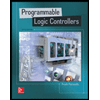
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education