First, create the custom exception class called OutOfStockException by creating a new class that extends the Exception class The class has a single constructor that takes a single argument, a string called message. The message argument is passed to the parent class (Exception) via the super keyword. Create the Store class and import the java.util.HashMap and java.util.Map classes The Store class is defined with a private Map called products, which is used to store the product names and their corresponding quantities. Create a default constructor that initializes the products map with three items: "apple", "banana", and "orange", with respective quantities of 10, 5, and 0. Define thepurchase method , which takes two arguments: a product name and a quantity to be purchased. This method throws an OutOfStockException if either the specified product is not available in the store or if the requested quantity is greater than the quantity available in stock. The method first checks if the product exists in the store's products map by using the containsKey method of the Map class. If the product is not in the store, the method throws an instance of the OutOfStockException class with a message indicating that the ("Product " +product+" not available in store") The second check in the purchase method uses the get method of the Map class to retrieve the quantity of the specified product in the store. If the requested quantity is greater than the quantity in stock, the method throws an OutOfStockException with a message indicating that the ("Product " +product+" out of stock") The template code provided to you defines a Main class that contains a main method. This method creates an instance of the Store class and calls the purchase method on it to try to purchase 11 units of the "apple" product.The purchase method call is enclosed in a try-catch block. This is because the purchase method is declared to throw an OutOfStockException, which means it can throw an exception in certain situations(e.g. when the product is out of stock or not available in the store). --------------------------------------------------------------------------------------------------------------------------------------------------------- public class Main { //Do not modify this code public static void main(String[] args) { Store store = new Store(); try { store.purchase("apple",11); System.out.println("Purchase successful!"); } catch (OutOfStockException e) { System.out.println(e.getMessage()); } } } --------------------------------------------------------------------------------------------------------------------------------------------------------- public class OutOfStockException extends Exception { // Custom exception class for when a product is out of stock public OutOfStockException() { // Call the superclass constructor to pass the error message to the parent class } } --------------------------------------------------------------------------------------------------------------------------------------------------------- import java.util.HashMap; import java.util.Map; public class Store { //create the map public Store() { // Initialize the products map with default values } public void purchase(String product, int quantity) throws OutOfStockException { // Check if the product is available in the store if (){ // If not, throw an OutOfStockException with a message indicating the product is not available } // Check if there is enough stock for the desired quantity if (){ // If not, throw an OutOfStockException with a message indicating the product is out of stock } } }
-
First, create the custom exception class called OutOfStockException by creating a new class that extends the Exception class
-
The class has a single constructor that takes a single argument, a string called message.
-
The message argument is passed to the parent class (Exception) via the super keyword.
-
Create the Store class and import the java.util.HashMap and java.util.Map classes
-
The Store class is defined with a private Map called products, which is used to store the product names and their corresponding quantities.
-
Create a default constructor that initializes the products map with three items: "apple", "banana", and "orange", with respective quantities of 10, 5, and 0.
-
Define thepurchase method , which takes two arguments: a product name and a quantity to be purchased. This method throws an OutOfStockException if either the specified product is not available in the store or if the requested quantity is greater than the quantity available in stock.
-
The method first checks if the product exists in the store's products map by using the containsKey method of the Map class.
-
If the product is not in the store, the method throws an instance of the OutOfStockException class with a message indicating that the ("Product " +product+" not available in store")
-
The second check in the purchase method uses the get method of the Map class to retrieve the quantity of the specified product in the store. If the requested quantity is greater than the quantity in stock, the method throws an OutOfStockException with a message indicating that the ("Product " +product+" out of stock")
-
The template code provided to you defines a Main class that contains a main method. This method creates an instance of the Store class and calls the purchase method on it to try to purchase 11 units of the "apple" product.The purchase method call is enclosed in a try-catch block. This is because the purchase method is declared to throw an OutOfStockException, which means it can throw an exception in certain situations(e.g. when the product is out of stock or not available in the store).
---------------------------------------------------------------------------------------------------------------------------------------------------------

Step by step
Solved in 4 steps with 5 images

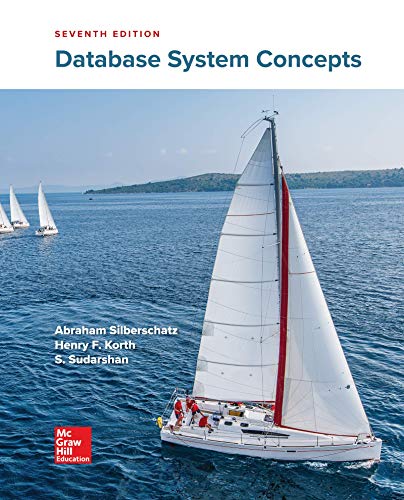
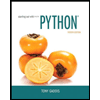
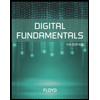
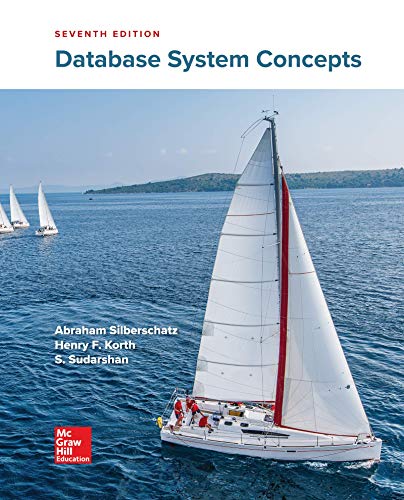
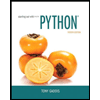
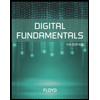
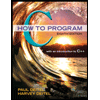
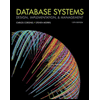
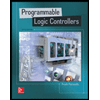