The reciprocal of a number is 1 divided by that number. The starter program below is expected to compute the reciprocal of all the numbers in a list. Fix the starter program to safely catch and handle incorrect divisions accordingly. If an exception occurs, catch the exception and print the exception. Your output should match the expected output below. Expected output The reciprocal of 1 is 1.0 The reciprocal of 2.5 is 0.4 The reciprocal of -6 is -0.17 division by zero The reciprocal of 0.4 is 2.5 The reciprocal of -0.17 is -5.88 unsupported operand type(s) for /: 'int' and 'str' The reciprocal of 3.14 is 0.32 type complex doesn't define __round__ method The reciprocal of 3.141592653589793 is 0.32 Here is the code from the starter file we are required to use: import math numbers = [1, 5 / 2, -6, 0, 0.4, -0.17, 'pi', 3.14, 2-6j, math.pi] for n in numbers: reciprocal = 1 / n print(f"The reciprocal of {n} is {round(reciprocal, 2)}")
Exception handling
The reciprocal of a number is 1 divided by that number. The starter program below is expected to compute the reciprocal of all the numbers in a list. Fix the starter program to safely catch and handle incorrect divisions accordingly. If an exception occurs, catch the exception and print the exception. Your output should match the expected output below.
Expected output
The reciprocal of 1 is 1.0 The reciprocal of 2.5 is 0.4 The reciprocal of -6 is -0.17 division by zero The reciprocal of 0.4 is 2.5 The reciprocal of -0.17 is -5.88 unsupported operand type(s) for /: 'int' and 'str' The reciprocal of 3.14 is 0.32 type complex doesn't define __round__ method The reciprocal of 3.141592653589793 is 0.32 |
Here is the code from the starter file we are required to use:
import math
numbers = [1, 5 / 2, -6, 0, 0.4, -0.17, 'pi', 3.14, 2-6j, math.pi]
for n in numbers:
reciprocal = 1 / n
print(f"The reciprocal of {n} is {round(reciprocal, 2)}")

Step by step
Solved in 2 steps with 2 images

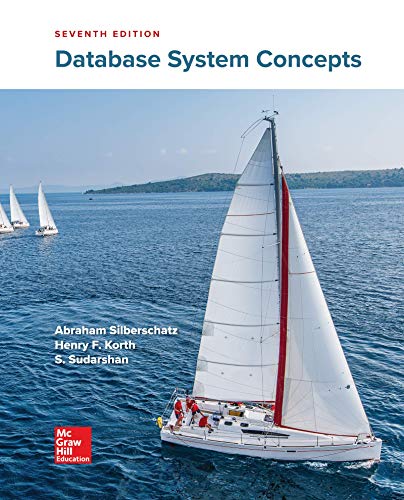
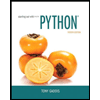
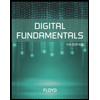
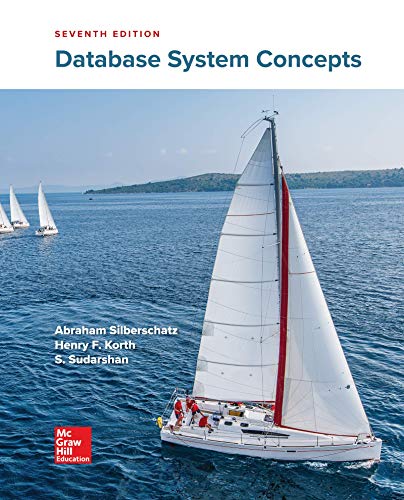
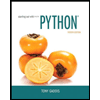
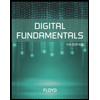
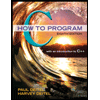
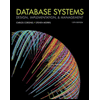
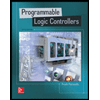