Create a function called get_age() to input the user's age. If the user enters a value that is outside the specified range, throw a ValueError exception. Create a function called fat_burning_heart_rate() to calculate and return the heart rate. Try getting the user age input in main() along with the exception handling. Prompt the user to continue the program with another age until the user indicates to quit.
Create a function called get_age() to input the user's age. If the user enters
a value that is outside the specified range, throw a ValueError exception.
Create a function called fat_burning_heart_rate() to calculate and return the heart rate.
Try getting the user age input in main() along with the exception handling.
Prompt the user to continue the program with another age until the user indicates to quit.
I have all of the main code written but I can't seem to figure out how to get it all within a main function that will restart when the user types yes and print bye bye for no.
This is the kind of output I want:
This program calculates the fat-burning heart rate based on age.
Enter an age between 18-75: 35
Fat burning heart rate for a 35 year-old: 129.5 bpm
Would you like to try another age? (yes/no)yes
Enter an age between 18-75: 17
Invalid age.
Could not calculate heart rate info.
Would you like to try another age? (yes/no)yes
Enter an age between 18-75: 18
Fat burning heart rate for a 18 year-old: 141.39999999999998 bpm
Would you like to try another age? (yes/no)yes
Enter an age between 18-75: 75
Fat burning heart rate for a 75 year-old: 101.5 bpm
Would you like to try another age? (yes/no)no
bye-bye
This is the code I have so far:
def get_age():
age = int(input("Enter an age between 18-75: "))
if age < 18 or age > 75:
raise ValueError('Invalid age.')
return age
def fat_burning_heart_rate(age):
heart_rate = (220 - age) * .7
return heart_rate
if __name__ == "__main__":
try:
age = get_age()
heart_rate = fat_burning_heart_rate(age)
print("Fat burning heart rate for ", age, 'year-old: ',heart_rate, 'bpm')
except ValueError as e:
print(e)
print("Could not calculate heart rate info.")
user_input = input("Would you like to try another age? (yes/no): ")
if user_input == 'no':
print("bye-bye")
if user_input == 'yes':
get_age()

Step by step
Solved in 3 steps with 1 images

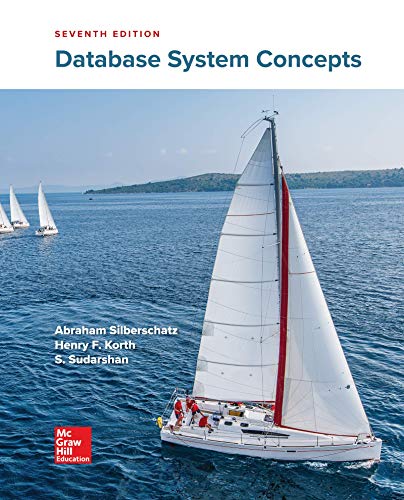
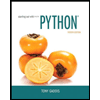
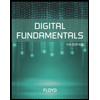
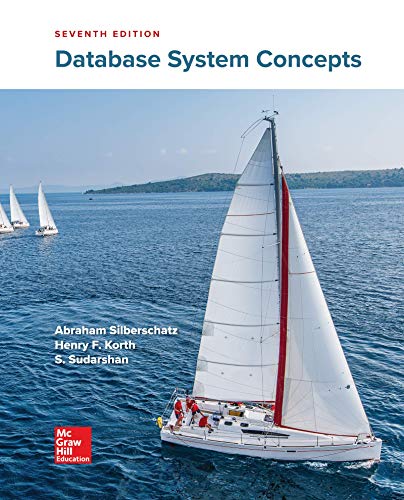
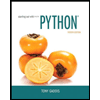
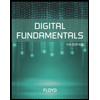
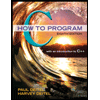
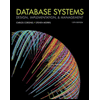
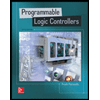