-Add a method to the tractor class to write all of a tractors attributes to a text file. Name it saveData(String filename) It should throw Exceptions. -Add a method to the tractor class to read all of a tractors attributes from a text file. Name it loadData(String filename) It should throw Exceptions. -Test these methods by calling them from your test drivers main() method public class Tractor { private String ID; private double rentalRate; private int rentalDays; Tractor() { this.ID = "00"; this.rentalDays = 0; this.rentalRate = 0; } } Tractor(String ID, double rentalRate, int rentalDays) { this.setID(ID); this.setRentalDays(rentalDays); this.setRentalRate(rentalRate); } /** * @return the iD */ public String getID() { return ID; } /** * @return the rentalDays */ public int getRentalDays() { return rentalDays; } /** * @return the rentalRate */ public double getRentalRate() { return rentalRate; } /** * @param iD the iD to set */ public void setID(String iD) { this.ID = iD; } /** * @param rentalDays the rentalDays to set */ public void setRentalDays(int rentalDays) { if (rentalDays < 0) { return; } this.rentalDays = rentalDays; } /** * @param rentalRate the rentalRate to set */ public void setRentalRate(double rentalRate) { if (rentalRate < 0) { return; } this.rentalRate = rentalRate; } public double RentalProfit() { return rentalRate * rentalDays; } @Override public String toString() { return "Tractor VIN: " + ID + "\nRental Days: " + rentalDays + "\nRental Rate: " + rentalRate+"\n"; } }
-Add a method to the tractor class to write all of a
tractors attributes to a text file. Name it saveData(String
filename) It should throw Exceptions.
-Add a method to the tractor class to read all of a
tractors attributes from a text file. Name it
loadData(String filename) It should throw Exceptions.
-Test these methods by calling them from your test
drivers main() method
public class Tractor {
private String ID;
private double rentalRate;
private int rentalDays;
Tractor() {
this.ID = "00";
this.rentalDays = 0;
this.rentalRate = 0;
}
}
Tractor(String ID, double rentalRate, int rentalDays) {
this.setID(ID);
this.setRentalDays(rentalDays);
this.setRentalRate(rentalRate);
}
/**
* @return the iD
*/
public String getID() {
return ID;
}
/**
* @return the rentalDays
*/
public int getRentalDays() {
return rentalDays;
}
/**
* @return the rentalRate
*/
public double getRentalRate() {
return rentalRate;
}
/**
* @param iD the iD to set
*/
public void setID(String iD) {
this.ID = iD;
}
/**
* @param rentalDays the rentalDays to set
*/
public void setRentalDays(int rentalDays) {
if (rentalDays < 0) {
return;
}
this.rentalDays = rentalDays;
}
/**
* @param rentalRate the rentalRate to set
*/
public void setRentalRate(double rentalRate) {
if (rentalRate < 0) {
return;
}
this.rentalRate = rentalRate;
}
public double RentalProfit() {
return rentalRate * rentalDays;
}
@Override
public String toString() {
return "Tractor VIN: " + ID + "\nRental Days: " + rentalDays + "\nRental Rate: " + rentalRate+"\n";
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

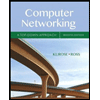
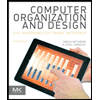
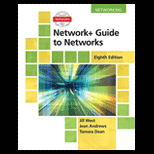
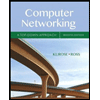
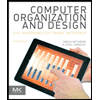
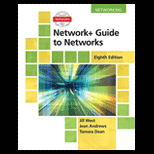
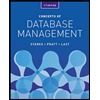
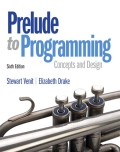
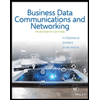