Implement in C Programming 6.4.1: Function definition: Volume of a pyramid with modular functions. Define a function CalcPyramidVolume with double data type parameters baseLength, baseWidth, and pyramidHeight, that returns as a double the volume of a pyramid with a rectangular base. CalcPyramidVolume() calls the given CalcBaseArea() function in the calculation. Relevant geometry equations: Volume = base area x height x 1/3 Base area = base length x base width. (Watch out for integer division). #include double CalcBaseArea(double baseLength, double baseWidth) { return baseLength * baseWidth; } /* Your solution goes here */ int main(void) { double userLength; double userWidth; double userHeight; scanf("%lf", &userLength); scanf("%lf", &userWidth); scanf("%lf", &userHeight); printf("Volume: %lf\n", CalcPyramidVolume(userLength, userWidth, userHeight)); return 0; }
Implement in C Programming
6.4.1: Function definition: Volume of a pyramid with modular functions.
Define a function CalcPyramidVolume with double data type parameters baseLength, baseWidth, and pyramidHeight, that returns as a double the volume of a pyramid with a rectangular base. CalcPyramidVolume() calls the given CalcBaseArea() function in the calculation.
Relevant geometry equations:
Volume = base area x height x 1/3
Base area = base length x base width.
(Watch out for integer division).
#include <stdio.h>
double CalcBaseArea(double baseLength, double baseWidth) {
return baseLength * baseWidth;
}
/* Your solution goes here */
int main(void) {
double userLength;
double userWidth;
double userHeight;
scanf("%lf", &userLength);
scanf("%lf", &userWidth);
scanf("%lf", &userHeight);
printf("Volume: %lf\n", CalcPyramidVolume(userLength, userWidth, userHeight));
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

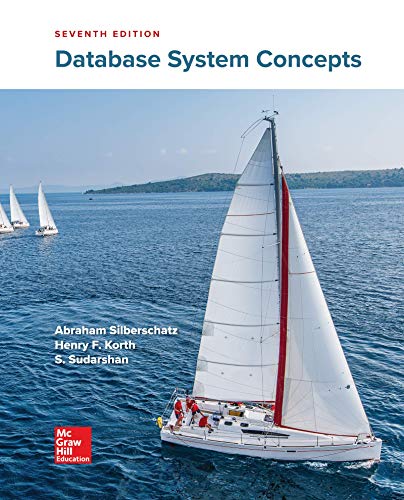
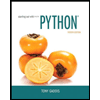
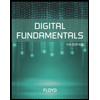
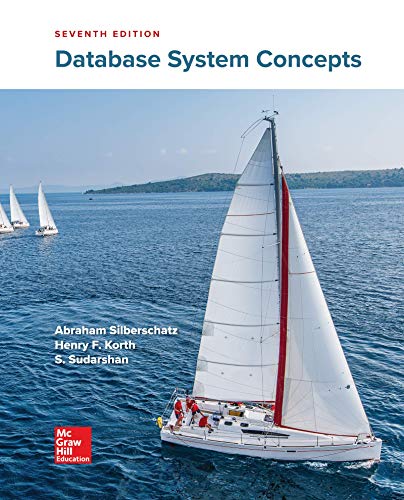
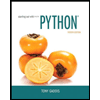
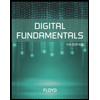
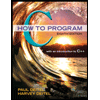
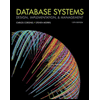
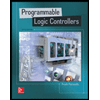