Implement a stack as an array of int[100] and an int variable top top is initialized to -1 (which means the stack is empty) Define three int functions: push (), pop(), and isEmpty() push(int v): if top>=99 print error message and return 0, otherwise increment top, put v into stack[top], return 0 pop(): if top<0 print error message and return 0, otherwise return stack[top] and decrement top isEmpty(): if top<0 then return 0, otherwise return 1 To test your program, run the following sequence of function calls, printing the values of the рops: pop (); push(1); push(2); push(3); pop(); push (4); push(5); pop(); pop(); push(6); pop(); pop();
Implement a stack as an array of int[100] and an int variable top top is initialized to -1 (which means the stack is empty) Define three int functions: push (), pop(), and isEmpty() push(int v): if top>=99 print error message and return 0, otherwise increment top, put v into stack[top], return 0 pop(): if top<0 print error message and return 0, otherwise return stack[top] and decrement top isEmpty(): if top<0 then return 0, otherwise return 1 To test your program, run the following sequence of function calls, printing the values of the рops: pop (); push(1); push(2); push(3); pop(); push (4); push(5); pop(); pop(); push(6); pop(); pop();
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How do I code the following stack in C program?
![### Implementing a Stack in C
Stacks are a fundamental data structure used in various applications in computer science. In this example, we will demonstrate how to implement a stack using an array in the C programming language.
A stack operates on a Last-In-First-Out (LIFO) principle, where the most recently added item is the first one to be removed.
#### Step-by-Step Implementation
1. **Initialization:**
- We will use an array of integers, `int[100]`, to store the stack elements.
- An integer variable `top` will keep track of the index of the topmost element. It is initialized to `-1`, indicating that the stack is empty.
2. **Defining Functions:**
- Three primary functions will be implemented: `push()`, `pop()`, and `isEmpty()`.
#### Function Definitions
1. **push(int v):**
- This function adds an integer `v` to the top of the stack.
- **Error Handling:** If the stack is full (i.e., `top >= 99`), it prints an error message and returns `0`.
- Otherwise, it increments the `top`, adds `v` to `stack[top]`, and returns `0`.
2. **pop():**
- This function removes and returns the topmost element from the stack.
- **Error Handling:** If the stack is empty (i.e., `top < 0`), it prints an error message and returns `0`.
- Otherwise, it returns the value of `stack[top]` and decrements the `top`.
3. **isEmpty():**
- This function checks if the stack is empty.
- It returns `0` if the stack is empty (`top < 0`) and `1` otherwise.
#### Implementation Code
```c
#include <stdio.h>
int stack[100];
int top = -1; // The stack is initially empty
int push(int v) {
if (top >= 99) {
printf("Error: Stack Overflow\n");
return 0;
} else {
top++;
stack[top] = v;
return 0;
}
}
int pop() {
if (top < 0) {
printf("Error: Stack Underflow\n");
return 0;
} else {
return stack[top--];
}
}
int](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8cb58778-70ba-4f7e-90ce-7267bd79a013%2Faffe827b-5f54-4a77-a366-d7f633df75b1%2Fehsqasj_processed.png&w=3840&q=75)
Transcribed Image Text:### Implementing a Stack in C
Stacks are a fundamental data structure used in various applications in computer science. In this example, we will demonstrate how to implement a stack using an array in the C programming language.
A stack operates on a Last-In-First-Out (LIFO) principle, where the most recently added item is the first one to be removed.
#### Step-by-Step Implementation
1. **Initialization:**
- We will use an array of integers, `int[100]`, to store the stack elements.
- An integer variable `top` will keep track of the index of the topmost element. It is initialized to `-1`, indicating that the stack is empty.
2. **Defining Functions:**
- Three primary functions will be implemented: `push()`, `pop()`, and `isEmpty()`.
#### Function Definitions
1. **push(int v):**
- This function adds an integer `v` to the top of the stack.
- **Error Handling:** If the stack is full (i.e., `top >= 99`), it prints an error message and returns `0`.
- Otherwise, it increments the `top`, adds `v` to `stack[top]`, and returns `0`.
2. **pop():**
- This function removes and returns the topmost element from the stack.
- **Error Handling:** If the stack is empty (i.e., `top < 0`), it prints an error message and returns `0`.
- Otherwise, it returns the value of `stack[top]` and decrements the `top`.
3. **isEmpty():**
- This function checks if the stack is empty.
- It returns `0` if the stack is empty (`top < 0`) and `1` otherwise.
#### Implementation Code
```c
#include <stdio.h>
int stack[100];
int top = -1; // The stack is initially empty
int push(int v) {
if (top >= 99) {
printf("Error: Stack Overflow\n");
return 0;
} else {
top++;
stack[top] = v;
return 0;
}
}
int pop() {
if (top < 0) {
printf("Error: Stack Underflow\n");
return 0;
} else {
return stack[top--];
}
}
int
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
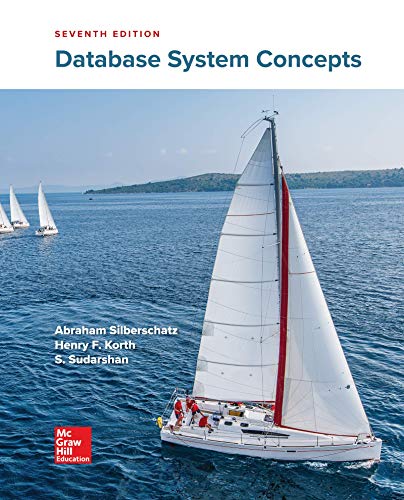
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
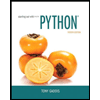
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
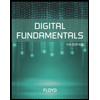
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
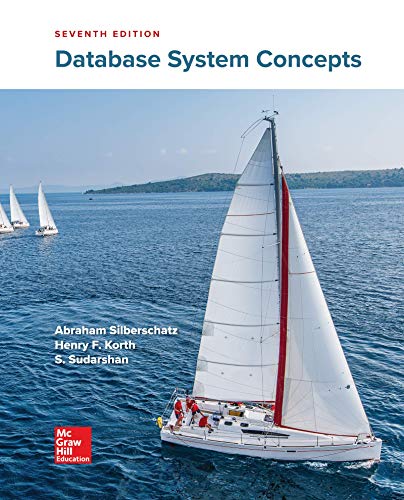
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
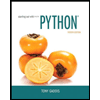
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
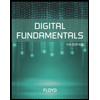
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
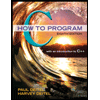
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
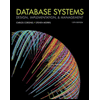
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
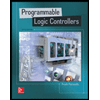
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education