Implement a class CountingArrayList that extends ArrayList and that counts the number of calls to get and set. import java.util.ArrayList; import java.util.Collections; public class CountingArrayListTester { public static String smallest(ArrayList values) { String smallestSoFar = values.get(0); for (int i = 1; i < values.size(); i++) { String value = values.get(i); if (value.compareTo(smallestSoFar) < 0) { smallestSoFar = value; } } return smallestSoFar; } public static void main(String[] args) { CountingArrayList words = new CountingArrayList(); words.add("Mary"); words.add("had"); words.add("a"); words.add("little"); words.add("lamb"); words.add("its"); words.add("fleece"); words.add("was"); words.add("white"); words.add("as"); words.add("snow"); String smallest = smallest(words); System.out.println(words.count("get")); System.out.println("Expected: 11"); System.out.println(words.count("set")); System.out.println("Expected: 0\n"); CountingArrayListTester2 tester2 = new CountingArrayListTester2(); tester2.main(null); } } import java.util.ArrayList; public class CountingArrayListTester2 { public static void reverse(ArrayList words) { for (int i = 0; i < words.size() / 2; i++) { String oldValue = words.set(words.size() - i - 1, words.get(i)); words.set(i, oldValue); } } public static void main(String[] args) { CountingArrayList words = new CountingArrayList(); words.add("Mary"); words.add("had"); words.add("a"); words.add("little"); words.add("lamb"); words.add("its"); words.add("fleece"); words.add("was"); words.add("white"); words.add("as"); words.add("snow"); reverse(words); System.out.println(words.count("get")); System.out.println("Expected: 5"); System.out.println(words.count("set")); System.out.println("Expected: 10"); System.out.println(words); System.out.println("Expected: [snow, as, white, was, " + "fleece, its, lamb, little, a, had, Mary]"); } }
Implement a class CountingArrayList that extends ArrayList<String> and that counts the number of calls to get and set.
import java.util.ArrayList;
import java.util.Collections;
public class CountingArrayListTester
{
public static String smallest(ArrayList<String> values)
{
String smallestSoFar = values.get(0);
for (int i = 1; i < values.size(); i++)
{
String value = values.get(i);
if (value.compareTo(smallestSoFar) < 0)
{
smallestSoFar = value;
}
}
return smallestSoFar;
}
public static void main(String[] args)
{
CountingArrayList words = new CountingArrayList();
words.add("Mary");
words.add("had");
words.add("a");
words.add("little");
words.add("lamb");
words.add("its");
words.add("fleece");
words.add("was");
words.add("white");
words.add("as");
words.add("snow");
String smallest = smallest(words);
System.out.println(words.count("get"));
System.out.println("Expected: 11");
System.out.println(words.count("set"));
System.out.println("Expected: 0\n");
CountingArrayListTester2 tester2 = new CountingArrayListTester2();
tester2.main(null);
}
}
import java.util.ArrayList;
public class CountingArrayListTester2
{
public static void reverse(ArrayList<String> words)
{
for (int i = 0; i < words.size() / 2; i++)
{
String oldValue = words.set(words.size() - i - 1,
words.get(i));
words.set(i, oldValue);
}
}
public static void main(String[] args)
{
CountingArrayList words = new CountingArrayList();
words.add("Mary");
words.add("had");
words.add("a");
words.add("little");
words.add("lamb");
words.add("its");
words.add("fleece");
words.add("was");
words.add("white");
words.add("as");
words.add("snow");
reverse(words);
System.out.println(words.count("get"));
System.out.println("Expected: 5");
System.out.println(words.count("set"));
System.out.println("Expected: 10");
System.out.println(words);
System.out.println("Expected: [snow, as, white, was, "
+ "fleece, its, lamb, little, a, had, Mary]");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

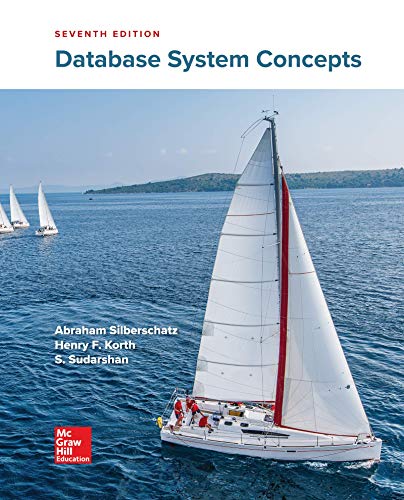
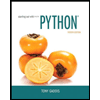
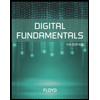
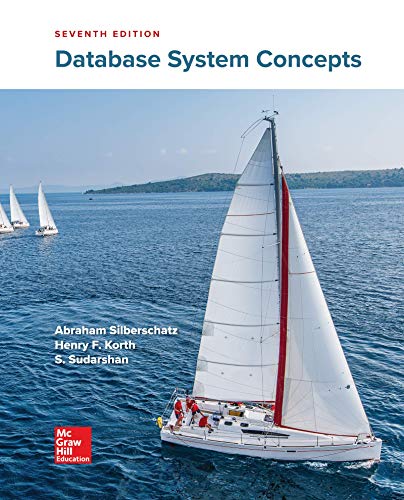
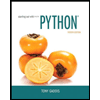
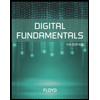
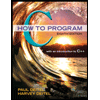
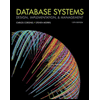
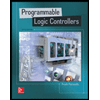