I'm having a problem with the following python code. The code is looks for "hidden treasure within a map". I get an error of heapq.heappush(open_list, (tile.f_cost, tile)) TypeError: '<' not supported between instances of 'Tile' and 'Tile', and I'm not sure why or how to fix it, any hints etc. would be great. import heapq import random class Tile: def __init__(self, x, y): self.x = x self.y = y self.is_obstacle = False self.g_cost = 0 self.h_cost = 0 self.f_cost = 0 self.parent = None class Map: def __init__(self, width, height): self.width = width self.height = height self.tiles = [[Tile(x, y) for y in range(height)] for x in range(width)] self.start_tile = self.get_tile(0, 0) self.treasure_tile = None self.generate_treasure() def generate_treasure(self): self.treasure_tile = self.get_random_tile() self.treasure_tile.is_obstacle = True def get_random_tile(self): x = random.randint(0, self.width - 1) y = random.randint(0, self.height - 1) return self.get_tile(x, y) def get_tile(self, x, y): return self.tiles[x][y] def get_adjacent_tiles(self, tile): adjacent_tiles = [] for x in range(tile.x - 1, tile.x + 2): for y in range(tile.y - 1, tile.y + 2): if x == tile.x and y == tile.y: continue if x < 0 or x >= self.width or y < 0 or y >= self.height: continue adjacent_tiles.append(self.get_tile(x, y)) return adjacent_tiles class AStar: def __init__(self, game_map, start_tile, treasure_tile): self.game_map = game_map self.start_tile = start_tile self.treasure_tile = treasure_tile def find_path(self): open_list = [] closed_list = [] start_tile = self.start_tile treasure_tile = self.treasure_tile heapq.heappush(open_list, (start_tile.f_cost, start_tile)) while len(open_list) > 0: current_tile = heapq.heappop(open_list)[1] closed_list.append(current_tile) if current_tile == treasure_tile: path = [] while current_tile != start_tile: path.append(current_tile) current_tile = current_tile.parent path.append(start_tile) path.reverse() return path adjacent_tiles = self.game_map.get_adjacent_tiles(current_tile) for tile in adjacent_tiles: if tile.is_obstacle or tile in closed_list: continue g_cost = current_tile.g_cost + 1 h_cost = abs(tile.x - treasure_tile.x) + abs(tile.y - treasure_tile.y) f_cost = g_cost + h_cost if (tile.f_cost, tile) in open_list: if tile.g_cost > g_cost: tile.g_cost = g_cost tile.h_cost = h_cost tile.f_cost = f_cost tile.parent = current_tile heapq.heapify(open_list) else: tile.g_cost = g_cost tile.h_cost = h_cost tile.f_cost = f_cost tile.parent = current_tile heapq.heappush(open_list, (tile.f_cost, tile)) return None game_board = Map(100,100) starting_place = Tile(30,15) treasure_place = Tile(1,1) play_game = AStar(game_board, starting_place, treasure_place) play_game.find_path()
I'm having a problem with the following python code. The code is looks for "hidden treasure within a map". I get an error of heapq.heappush(open_list, (tile.f_cost, tile))
TypeError: '<' not supported between instances of 'Tile' and 'Tile', and I'm not sure why or how to fix it, any hints etc. would be great.
import heapq
import random
class Tile:
def __init__(self, x, y):
self.x = x
self.y = y
self.is_obstacle = False
self.g_cost = 0
self.h_cost = 0
self.f_cost = 0
self.parent = None
class Map:
def __init__(self, width, height):
self.width = width
self.height = height
self.tiles = [[Tile(x, y) for y in range(height)] for x in range(width)]
self.start_tile = self.get_tile(0, 0)
self.treasure_tile = None
self.generate_treasure()
def generate_treasure(self):
self.treasure_tile = self.get_random_tile()
self.treasure_tile.is_obstacle = True
def get_random_tile(self):
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
return self.get_tile(x, y)
def get_tile(self, x, y):
return self.tiles[x][y]
def get_adjacent_tiles(self, tile):
adjacent_tiles = []
for x in range(tile.x - 1, tile.x + 2):
for y in range(tile.y - 1, tile.y + 2):
if x == tile.x and y == tile.y:
continue
if x < 0 or x >= self.width or y < 0 or y >= self.height:
continue
adjacent_tiles.append(self.get_tile(x, y))
return adjacent_tiles
class AStar:
def __init__(self, game_map, start_tile, treasure_tile):
self.game_map = game_map
self.start_tile = start_tile
self.treasure_tile = treasure_tile
def find_path(self):
open_list = []
closed_list = []
start_tile = self.start_tile
treasure_tile = self.treasure_tile
heapq.heappush(open_list, (start_tile.f_cost, start_tile))
while len(open_list) > 0:
current_tile = heapq.heappop(open_list)[1]
closed_list.append(current_tile)
if current_tile == treasure_tile:
path = []
while current_tile != start_tile:
path.append(current_tile)
current_tile = current_tile.parent
path.append(start_tile)
path.reverse()
return path
adjacent_tiles = self.game_map.get_adjacent_tiles(current_tile)
for tile in adjacent_tiles:
if tile.is_obstacle or tile in closed_list:
continue
g_cost = current_tile.g_cost + 1
h_cost = abs(tile.x - treasure_tile.x) + abs(tile.y - treasure_tile.y)
f_cost = g_cost + h_cost
if (tile.f_cost, tile) in open_list:
if tile.g_cost > g_cost:
tile.g_cost = g_cost
tile.h_cost = h_cost
tile.f_cost = f_cost
tile.parent = current_tile
heapq.heapify(open_list)
else:
tile.g_cost = g_cost
tile.h_cost = h_cost
tile.f_cost = f_cost
tile.parent = current_tile
heapq.heappush(open_list, (tile.f_cost, tile))
return None
game_board = Map(100,100)
starting_place = Tile(30,15)
treasure_place = Tile(1,1)
play_game = AStar(game_board, starting_place, treasure_place)
play_game.find_path()

Tile Class:
- Define a
Tile
class to represent each cell on the map. - Each
Tile
object stores its position(x, y)
, whether it's an obstacle (is_obstacle
), and the cost values for the A* algorithm (g_cost
,h_cost
, andf_cost
). - Implement comparison methods (
__lt__
and__eq__
) to allowTile
objects to be compared based on theirf_cost
values.
- Define a
Map Class:
- Create a
Map
class to represent the game map. - A 2D grid of Tile objects makes up the map.
- Initialize the map with dimensions (width and height) and generate a random position for the treasure (obstacle).
- Implement methods to access tiles, get adjacent tiles, and generate random tiles.
- Create a
AStar Class:
- Create an
AStar
class to perform the A* search. - Initialize the A* algorithm with the game map, starting tile, and treasure tile.
- Implement the
find_path
method to find the path from the starting point to the treasure using A*. - Use open and closed lists to keep track of visited tiles.
- Calculate the
f_cost
for each tile based ong_cost
(cost from the start),h_cost
(heuristic cost to the treasure), andf_cost
(total cost).
- Create an
Main Part:
- Create an instance of the game map with dimensions (10x10), a starting tile at (0, 0), and a randomly generated treasure tile.
- Print the location of the treasure on the map.
- Initialize the A* path finder with the game map and starting and treasure tiles.
- Find the path using the
find_path
method. - If a path is found, print the path from the starting point to the treasure; otherwise, indicate that no path was found.
Output:
- Display the location of the treasure (obstacle) on the map.
- If a path exists, show the path from the starting point to the treasure.
Step by step
Solved in 4 steps with 5 images

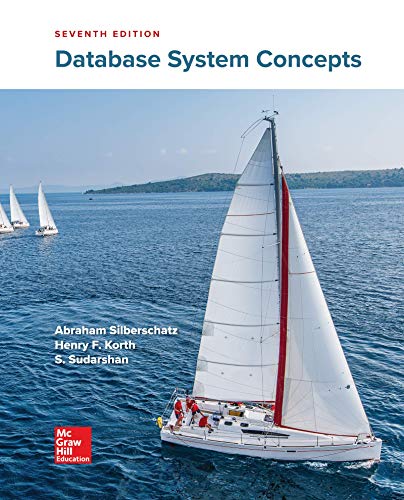
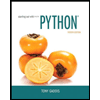
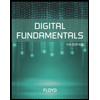
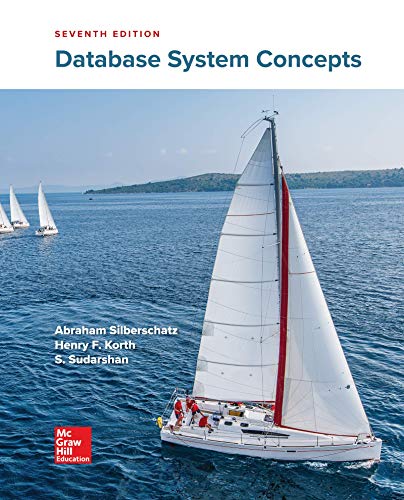
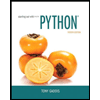
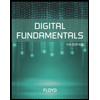
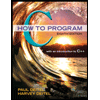
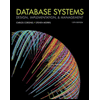
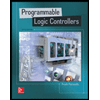