I've attached the question. Below, you will find my python code answer. It doesn't output correctly, as you can see in the image with errors. Code: class Node: def __init__(self, data): self.data = data self.next = None self.prev = None # Empty Doubly Linked List class DoublyLinkedList: def __init__(self): self.head = None def append(self, new_data): if self.head == None: self.head = new_data self.tail = new_data else: self.tail.next = new_data new_data.prev = self.tail self.tail = new_data def printList(self): if(self.head == None): print('Empty!') else: node = self.head while(node is not None): print(node.data), node = node.next def remove(self, current_node): successor_node = current_node.next predecessor_node = current_node.prev if successor_node is not None: successor_node.prev = predecessor_node if predecessor_node is not None: predecessor_node.next = successor_node if current_node is self.head: self.head = successor_node if current_node is self.tail: self.tail = predecessor_node def node_search(self,nodeA): node = self.head while(node is not None): if(node.data==nodeA.data): return True node=node.next return False def chunck_removal(self,nodeA): node = self.head temp=None while(node is not None): if(node.data==nodeA.data): temp.next = None break temp=node node=node.next if __name__ == '__main__': d=DoublyLinkedList() while(True): n=int(input()) if(n==-1): break else: d.append(Node(n)) removeNodeDate=int(input()) if(d.node_search(Node(removeNodeDate))): d.chunck_removal(Node(removeNodeDate)) d.printList() else: print('Node not found')
I've attached the question. Below, you will find my python code answer. It doesn't output correctly, as you can see in the image with errors.
Code:
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
# Empty Doubly Linked List
class DoublyLinkedList:
def __init__(self):
self.head = None
def append(self, new_data):
if self.head == None:
self.head = new_data
self.tail = new_data
else:
self.tail.next = new_data
new_data.prev = self.tail
self.tail = new_data
def printList(self):
if(self.head == None):
print('Empty!')
else:
node = self.head
while(node is not None):
print(node.data),
node = node.next
def remove(self, current_node):
successor_node = current_node.next
predecessor_node = current_node.prev
if successor_node is not None:
successor_node.prev = predecessor_node
if predecessor_node is not None:
predecessor_node.next = successor_node
if current_node is self.head:
self.head = successor_node
if current_node is self.tail:
self.tail = predecessor_node
def node_search(self,nodeA):
node = self.head
while(node is not None):
if(node.data==nodeA.data):
return True
node=node.next
return False
def chunck_removal(self,nodeA):
node = self.head
temp=None
while(node is not None):
if(node.data==nodeA.data):
temp.next = None
break
temp=node
node=node.next
if __name__ == '__main__':
d=DoublyLinkedList()
while(True):
n=int(input())
if(n==-1):
break
else:
d.append(Node(n))
removeNodeDate=int(input())
if(d.node_search(Node(removeNodeDate))):
d.chunck_removal(Node(removeNodeDate))
d.printList()
else:
print('Node not found')



Step by step
Solved in 6 steps with 4 images

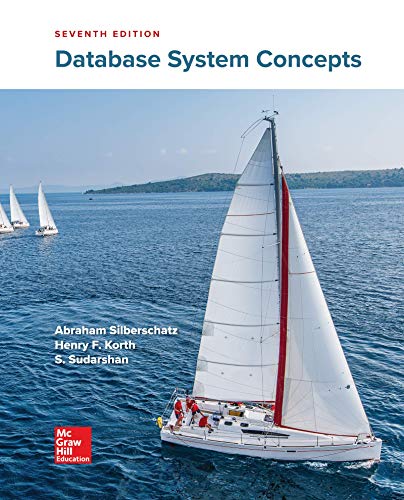
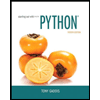
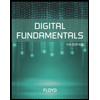
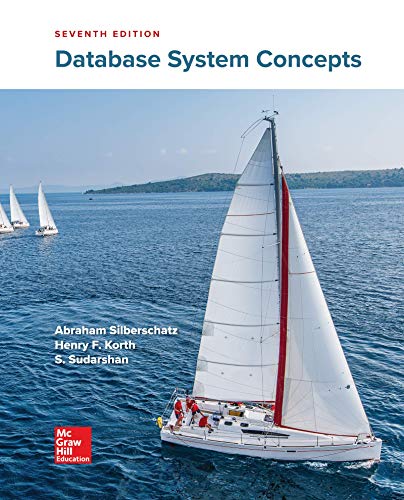
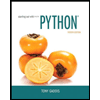
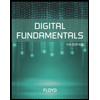
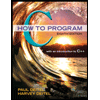
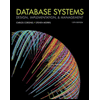
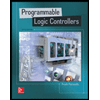