Computer Science Question , Create a GUI for the code below import java.util.ArrayList; import java.util.List; public class Budget { private String name; private double balance; private List expenses; public Budget(String name, double balance) { this.name = name; this.balance = balance; this.expenses = new ArrayList<>(); } public void addExpense(Expense expense) { if (expense.getAmount() <= this.balance) { this.expenses.add(expense); this.balance -= expense.getAmount(); } else { System.out.println("Error: not enough funds to add expense"); } } public double getBalance() { return this.balance; } public List getExpenses() { return this.expenses; } } Step 2/2 Expense.java: public class Expense { private String name; private double amount; public Expense(String name, double amount) { this.name = name; this.amount = amount; } public double getAmount() { return this.amount; } public String toString() { return this.name + ": " + this.amount; } } Here's an example usage of the Budget and Expense classes: public class Main { public static void main(String[] args) { Budget budget = new Budget("Monthly Budget", 1000.0); Expense expense1 = new Expense("Rent", 800.0); Expense expense2 = new Expense("Groceries", 100.0); Expense expense3 = new Expense("Gas", 50.0); budget.addExpense(expense1); budget.addExpense(expense2); budget.addExpense(expense3); System.out.println("Expenses:"); for (Expense expense : budget.getExpenses()) { System.out.println(expense); } System.out.println("Balance: " + budget.getBalance()); } } Output: Expenses: Rent: 800.0 Groceries: 100.0 Gas: 50.0 Balance: 50.0
Computer Science
Question , Create a GUI for the code below
import java.util.ArrayList;
import java.util.List;
public class Budget {
private String name;
private double balance;
private List<Expense> expenses;
public Budget(String name, double balance) {
this.name = name;
this.balance = balance;
this.expenses = new ArrayList<>();
}
public void addExpense(Expense expense) {
if (expense.getAmount() <= this.balance) {
this.expenses.add(expense);
this.balance -= expense.getAmount();
} else {
System.out.println("Error: not enough funds to add expense");
}
}
public double getBalance() {
return this.balance;
}
public List<Expense> getExpenses() {
return this.expenses;
}
}
Step 2/2
Expense.java:
public class Expense {
private String name;
private double amount;
public Expense(String name, double amount) {
this.name = name;
this.amount = amount;
}
public double getAmount() {
return this.amount;
}
public String toString() {
return this.name + ": " + this.amount;
}
}
Here's an example usage of the Budget and Expense classes:
public class Main {
public static void main(String[] args) {
Budget budget = new Budget("Monthly Budget", 1000.0);
Expense expense1 = new Expense("Rent", 800.0);
Expense expense2 = new Expense("Groceries", 100.0);
Expense expense3 = new Expense("Gas", 50.0);
budget.addExpense(expense1);
budget.addExpense(expense2);
budget.addExpense(expense3);
System.out.println("Expenses:");
for (Expense expense : budget.getExpenses()) {
System.out.println(expense);
}
System.out.println("Balance: " + budget.getBalance());
}
}
Output:
Expenses:
Rent: 800.0
Groceries: 100.0
Gas: 50.0
Balance: 50.0

Step by step
Solved in 3 steps with 1 images

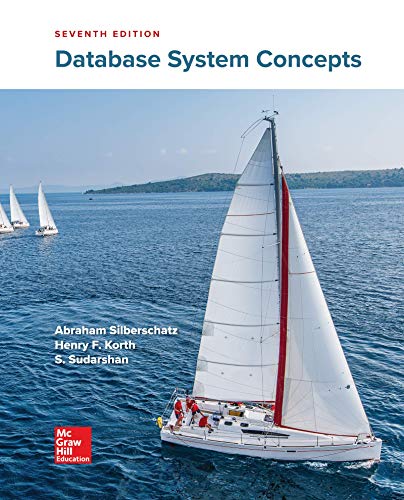
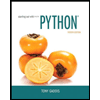
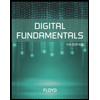
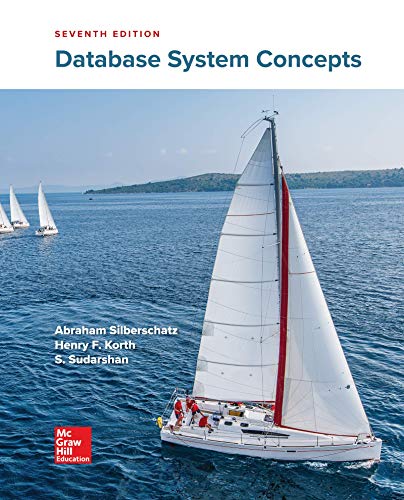
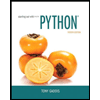
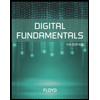
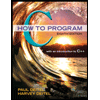
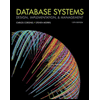
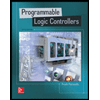