I need help with my RPN calculator. It keeps outputting null for every expression. Any help is appreciated. I added my code below. RPNStringTokenizer.java import java.util.ArrayList; public interface RPNStringTokenizer { public static ArrayList tokenize (String expression) { // take a string. If it is valid RPN stuff - integers or operators // - then put them in a list for processing. // anything bad (not int or one of the operators we like) and return a null. // YOU WRITE THIS! return null; } } YourArrayListStack.java import java.util.ArrayList; public class YourArrayListStack implements YourStack { // YOU MUST USE THIS IMPLEMENTATION - just code the methods ArrayList theStack = new ArrayList<>(); @Override public void push(Integer i) { theStack.add(i); } @Override public Integer pop() { if(theStack.isEmpty()) { return null; } else { return theStack.remove(theStack.size()-1); } } @Override public Integer size() { return theStack.size(); } } YourLinkedListStack.java public class YourLinkedListStack implements YourStack { // YOU MUST USE THIS IMPLEMENTATION - just code the methods private YourStackNode head = null; private Integer size = 0; @Override public void push(Integer i) { YourStackNode newNode=new YourStackNode(); newNode.item = i; newNode.next= head; head = newNode; size++; } @Override public Integer pop() { if(head==null) { return null; } else { int item = head.item; head = head.next; size--; return item; } } @Override public Integer size() { return size; } } YourStackNode.java public class YourStackNode { // DO NOT CHANGE THIS! Integer item; YourStackNode next; public Integer getItem() { return item; } public YourStackNode getNext() { return next; } public void setItem (Integer i) { item = i; } public void setNext (YourStackNode node) { next = node; } } YourStack.java public interface YourStack { public void push (Integer i); public Integer pop(); public Integer size(); } RPNTester.java import java.util.ArrayList; public class RPNTester { public static void main(String[] args) { // don't change this method! YourRPNCalculator calc = new YourRPNCalculator(new YourArrayListStack()); System.out.println("Testing ArrayList version"); testRPNCalculator(calc); calc = new YourRPNCalculator(new YourLinkedListStack()); System.out.println("Testing LinkedList version"); testRPNCalculator(calc); } private static void testRPNCalculator(SimpleRPNCalculator calc) { ArrayList testExpressions = new ArrayList<>(); testExpressions.add("1 1 +"); // 2 testExpressions.add("1 3 -"); // 2 testExpressions.add("1 1 + 2 *"); // 4 testExpressions.add("1 1 2 + *"); // 3 testExpressions.add("1 1 + 2 2 * -"); // 2 testExpressions.add("11 bv +"); // bad token testExpressions.add("2 3 + -"); // underflow on an operator testExpressions.add("2 3 + 4 5 -"); // leftover tokens // YOU SHOULD ADD MORE TEST CASES! for (String s : testExpressions) { System.out.println(calc.calculate(s)); } } } YourRPNCalculator.java import java.util.ArrayList; public class YourRPNCalculator implements SimpleRPNCalculator { // don't change these... YourStack theStack = null; public YourRPNCalculator(YourStack stack) { theStack = stack; } @Override public String calculate(String inputString) { // this is probably helpful, but you can remove... ArrayList tokens = RPNStringTokenizer.tokenize(inputString); // here's the calculator logic! if (tokens==null) { return null; } for(String token : tokens) { if(token.matches("\\d+")) { int num = Integer.parseInt(token); theStack.push(num); } else { //operator if (theStack.size()<2) { return null; } int op2 = theStack.pop(); int op1 = theStack.pop(); int result; switch(token) { case "+": result = op1 + op2 ; break; case "-": result = op1 - op2; break; case "*": result = op1 * op2; break; default: return null; } theStack.push(result); } } if (theStack.size()!=1) { return"Bad expression"; } return String.valueOf(theStack.pop()); } }
I need help with my RPN calculator. It keeps outputting null for every expression. Any help is appreciated. I added my code below.
RPNStringTokenizer.java
import java.util.ArrayList;
public interface RPNStringTokenizer {
public static ArrayList<String> tokenize (String expression) {
// take a string. If it is valid RPN stuff - integers or operators
// - then put them in a list for processing.
// anything bad (not int or one of the operators we like) and return a null.
// YOU WRITE THIS!
return null;
}
}
YourArrayListStack.java
import java.util.ArrayList;
public class YourArrayListStack implements YourStack {
// YOU MUST USE THIS IMPLEMENTATION - just code the methods
ArrayList<Integer> theStack = new ArrayList<>();
@Override
public void push(Integer i) {
theStack.add(i);
}
@Override
public Integer pop() {
if(theStack.isEmpty()) {
return null;
}
else {
return theStack.remove(theStack.size()-1);
}
}
@Override
public Integer size() {
return theStack.size();
}
}
YourLinkedListStack.java
public class YourLinkedListStack implements YourStack {
// YOU MUST USE THIS IMPLEMENTATION - just code the methods
private YourStackNode head = null;
private Integer size = 0;
@Override
public void push(Integer i) {
YourStackNode newNode=new YourStackNode();
newNode.item = i;
newNode.next= head;
head = newNode;
size++;
}
@Override
public Integer pop() {
if(head==null) {
return null;
}
else {
int item = head.item;
head = head.next;
size--;
return item;
}
}
@Override
public Integer size() {
return size;
}
}
YourStackNode.java
public class YourStackNode {
// DO NOT CHANGE THIS!
Integer item;
YourStackNode next;
public Integer getItem() {
return item;
}
public YourStackNode getNext() {
return next;
}
public void setItem (Integer i) {
item = i;
}
public void setNext (YourStackNode node) {
next = node;
}
}
YourStack.java
public interface YourStack {
public void push (Integer i);
public Integer pop();
public Integer size();
}
RPNTester.java
import java.util.ArrayList;
public class RPNTester {
public static void main(String[] args) {
// don't change this method!
YourRPNCalculator calc = new YourRPNCalculator(new YourArrayListStack());
System.out.println("Testing ArrayList version");
testRPNCalculator(calc);
calc = new YourRPNCalculator(new YourLinkedListStack());
System.out.println("Testing LinkedList version");
testRPNCalculator(calc);
}
private static void testRPNCalculator(SimpleRPNCalculator calc) {
ArrayList<String> testExpressions = new ArrayList<>();
testExpressions.add("1 1 +"); // 2
testExpressions.add("1 3 -"); // 2
testExpressions.add("1 1 + 2 *"); // 4
testExpressions.add("1 1 2 + *"); // 3
testExpressions.add("1 1 + 2 2 * -"); // 2
testExpressions.add("11 bv +"); // bad token
testExpressions.add("2 3 + -"); // underflow on an operator
testExpressions.add("2 3 + 4 5 -"); // leftover tokens
// YOU SHOULD ADD MORE TEST CASES!
for (String s : testExpressions) {
System.out.println(calc.calculate(s));
}
}
}
YourRPNCalculator.java
import java.util.ArrayList;
public class YourRPNCalculator implements SimpleRPNCalculator {
// don't change these...
YourStack theStack = null;
public YourRPNCalculator(YourStack stack) {
theStack = stack;
}
@Override
public String calculate(String inputString) {
// this is probably helpful, but you can remove...
ArrayList<String> tokens = RPNStringTokenizer.tokenize(inputString);
// here's the calculator logic!
if (tokens==null) {
return null;
}
for(String token : tokens) {
if(token.matches("\\d+")) {
int num = Integer.parseInt(token);
theStack.push(num);
}
else {
//operator
if (theStack.size()<2) {
return null;
}
int op2 = theStack.pop();
int op1 = theStack.pop();
int result;
switch(token) {
case "+":
result = op1 + op2 ;
break;
case "-":
result = op1 - op2;
break;
case "*":
result = op1 * op2;
break;
default:
return null;
}
theStack.push(result);
}
}
if (theStack.size()!=1) {
return"Bad expression";
}
return String.valueOf(theStack.pop());
}
}

Step by step
Solved in 3 steps

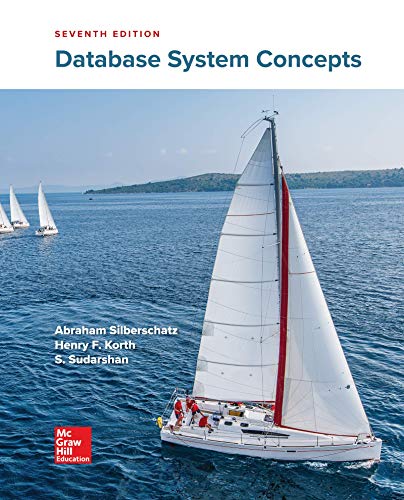
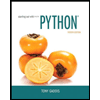
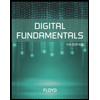
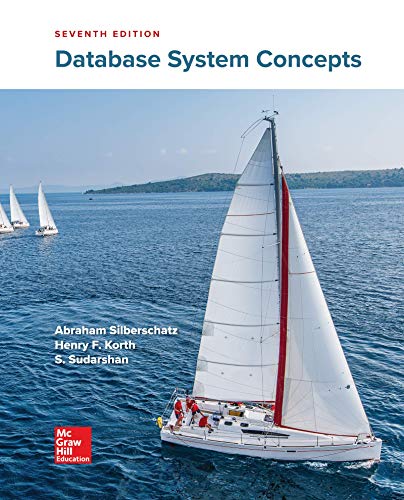
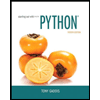
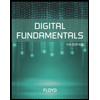
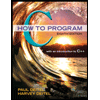
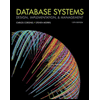
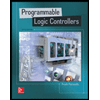