I need some code redone, I have a basic setup of the code but I would like to make a few minor changes to it, basically, in c prgramming, in the graph shown below, if a user were to input the value of .8907, the z value returned from the code would be .03 + 1.2 = 1.23. I would like to understand the new coded you provide so if you could use comments as well that would be awesome!
I need some code redone, I have a basic setup of the code but I would like to make a few minor changes to it, basically, in c prgramming, in the graph shown below, if a user were to input the value of .8907, the z value returned from the code would be .03 + 1.2 = 1.23. I would like to understand the new coded you provide so if you could use comments as well that would be awesome!
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
I need some code redone, I have a basic setup of the code but I would like to make a few minor changes to it, basically, in c prgramming, in the graph shown below, if a user were to input the value of .8907, the z value returned from the code would be .03 + 1.2 = 1.23.
I would like to understand the new coded you provide so if you could use comments as well that would be awesome!
![### C Program to Read and Process Data from a File
Below is a C program that reads data from a file named "Distribution.txt" and processes it to find the closest z-value. The program ensures the input falls within a specified range and performs calculations to find the nearest z-value from the data read.
```c
#include <stdio.h>
int main()
{
double z_array [40][10];
double z;
FILE *f = fopen ("Distribution.txt", "r");
for (int i = 0; i < 40; i++) {
fscanf (f, "%lf", &z);
for (int j = 0; j < 10; j++)
fscanf (f, "%lf", &z_array [i][j]);
}
fclose (f);
while (1) {
printf ("Values greater than one and less than .5 will not be accepted: ");
scanf ("%lf", &z);
if ((z < 0.5) || (z > 1)){
break;
}
}
int i, j;
double prev;
for (i = 0; i < 40; i++) {
for (j = 0; j < 10; j++) {
if (z_array [i][j] > z) break;
else prev = z_array [i][j];
}
if (z_array [i][j] > z) break;
}
if ((z - prev) < (z_array [i][j] - z)) {
// previous item is nearest
if (j > 0) j--;
else {
i--;
j = 9;
}
}
double zval = i * 0.1 + j * 0.01;
printf ("%0.4lf's z-value is %0.2lf\n", z, zval);
}
```
### Explanation
**1. Including the Header File:**
```c
#include <stdio.h>
```
This includes the standard input-output library used for file manipulation and console I/O.
**2. Defining the Main Function:**
```c
int main() {
```
This defines the main function which serves as the entry point of the program.
**3. Declaring Variables:**
```c
double z_array[40][10];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F986a0f43-c508-42df-9c7a-5fee8dc0775f%2Fa3bdd262-4df6-4105-962e-53e199fcc197%2Fhdbhre_processed.png&w=3840&q=75)
Transcribed Image Text:### C Program to Read and Process Data from a File
Below is a C program that reads data from a file named "Distribution.txt" and processes it to find the closest z-value. The program ensures the input falls within a specified range and performs calculations to find the nearest z-value from the data read.
```c
#include <stdio.h>
int main()
{
double z_array [40][10];
double z;
FILE *f = fopen ("Distribution.txt", "r");
for (int i = 0; i < 40; i++) {
fscanf (f, "%lf", &z);
for (int j = 0; j < 10; j++)
fscanf (f, "%lf", &z_array [i][j]);
}
fclose (f);
while (1) {
printf ("Values greater than one and less than .5 will not be accepted: ");
scanf ("%lf", &z);
if ((z < 0.5) || (z > 1)){
break;
}
}
int i, j;
double prev;
for (i = 0; i < 40; i++) {
for (j = 0; j < 10; j++) {
if (z_array [i][j] > z) break;
else prev = z_array [i][j];
}
if (z_array [i][j] > z) break;
}
if ((z - prev) < (z_array [i][j] - z)) {
// previous item is nearest
if (j > 0) j--;
else {
i--;
j = 9;
}
}
double zval = i * 0.1 + j * 0.01;
printf ("%0.4lf's z-value is %0.2lf\n", z, zval);
}
```
### Explanation
**1. Including the Header File:**
```c
#include <stdio.h>
```
This includes the standard input-output library used for file manipulation and console I/O.
**2. Defining the Main Function:**
```c
int main() {
```
This defines the main function which serves as the entry point of the program.
**3. Declaring Variables:**
```c
double z_array[40][10];

Transcribed Image Text:### z-Score Table
The table provided is a portion of the standard normal distribution table, commonly used in statistics to find the probability that a statistic is observed below, above, or between certain values. This is also known as a z-table.
#### Understanding the Table
Each cell in the table represents the cumulative probability from the mean to a specific z-score. The cumulative probability is a way of representing the area under the curve for the standard normal distribution.
- **First Column (z):** This column represents the z-score ranging from 0.0 to 1.2 in increments of 0.1.
- **Top Row (Decimal part):** This row represents the second decimal place of the z-score, ranging from 0.00 to 0.09.
#### Example Explanation
To find the cumulative probability for a z-score of 1.23:
1. Locate the value in the first column ("1.2").
2. Locate the value in the top row ("0.03").
3. The intersection of the row and column gives the cumulative probability.
In this case, if you look at the row for 1.2 and the column for 0.03, the intersecting value is **0.8907**. This means that approximately 89.07% of the distribution is below a z-score of 1.23.
#### Highlighted Example
- The row with z = **1.2** is highlighted.
- The column corresponding to the decimal part **0.03** is highlighted.
- The intersecting value (highlighted) for a z-score of **1.23** is **0.8907**.
This representation is crucial for many statistical methods including hypothesis testing, confidence intervals, and more, providing a standardized method for comparing different datasets and observations.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
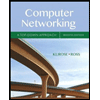
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
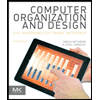
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
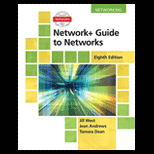
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
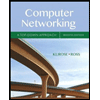
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
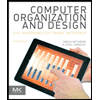
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
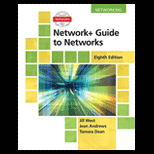
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
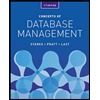
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
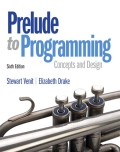
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
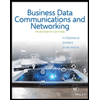
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY