To your code for Lab0, convert 3 of your functions to Dunders. Pick Dunders of your choice and note whether the Lab0 function you are replacing is binary or unary. An example of a unary Dunder is the __len__ and __add__ is binary. Click this link to get a Dunder list and more info about using Dunders: https://www.geeksforgeeks.org/customize-your-python-class-with-magic-or-dunder-methods/?ref=rpLinks to an external site. Then demonstrate your Dunders are used. mycode.py import re from urllib.request import urlopen from bs4 import BeautifulSoup from collections import defaultdict class WebCraping: def __init__(self): self.soup = None self.rows = [] self.dict = defaultdict(list) def openHtmlSite(self, htmlsite): html = urlopen(htmlsite) self.soup = BeautifulSoup(html, 'html.parser') # Children def children(self): [print(child.name) for child in self.soup.recursiveChildGenerator() if child.name is not None] #findAll def findAll(self, tags): dict = {} for tag in self.soup.find_all(tags): print("{0}: {1}".format(tag.name, tag.text)) r = re.compile(r'\s') s = r.split(tag.text) dict[s[0]] = s[1] for k, v in dict.items(): print('key= ', k, '\tvalue= ', v) # Append tag def appendTag(self, tag, nustr): newtag = self.soup.new_tag(tag) newtag.string = nustr ultag = self.soup.ul ultag.append(newtag) print(ultag.prettify()) # Inserting a new tag at position def insertAt(self, tag, nustr, index): newtag = self.soup.new_tag(tag) newtag.string = nustr ultag = self.soup.ul ultag.insert(index, newtag) print(ultag.prettify()) # Select list element def selectIndex(self, index): sel = "li:nth-of-type(" + str(index) + ")" print(self.soup.select(sel)) # Select paragraph def selectParagraph(self): spanElem = self.soup.select('p') print(spanElem) for i in range(0, len(spanElem)): print(str((spanElem)[i])) # Selects all span in given site def selectSpan(self): spanElem = self.soup.select('span') print(spanElem) for i in range(0, len(spanElem)): print(str((spanElem)[i])) # Find data we need: Country Name, data in 1980 and 2018 def find_data(self): self.dict = defaultdict() table = self.soup.find('table', class_='wikitable sortable') self.rows = table.find_all('tr') # Collect data for i in self.rows: # Find all info of td - table data in the site column = i.find_all('td') # Collect data and format them (country - strip(), number data - replace \xa0 and \n) if column: country = column[0].text.strip() year1 = column[1].text.replace('\xa0', '') year2 = column[14].text.replace('\n', '') # Store data in the default dict self.dict[country] = (year1, year2) # Print data def print_data(self): # Print collected data print("Production-based emissions: annual carbon dioxide emissions in metric tons per capita in 1980 and 2018") print("{:>46} {:>9} {:>10}".format("COUNTRY NAME", "1980", "2018")) for k, v in self.dict.items(): y1, y2 = v print(f'{k:>46}' + "{:>10} {:>10}".format(y1, y2)) # Print defaultdict def print_dict(self): print(self.dict) if __name__ == "__main__": openHtmlSite = WebCraping() openHtmlSite.openHtmlSite('https://en.wikipedia.org/wiki/List_of_countries_by_carbon_dioxide_emissions_per_capita') openHtmlSite.find_data() openHtmlSite.print_data() openHtmlSite.print_dict()
To your code for Lab0, convert 3 of your functions to Dunders. Pick Dunders of your choice and note whether the Lab0 function you are replacing is binary or unary. An example of a unary Dunder is the __len__ and __add__ is binary. Click this link to get a Dunder list and more info about using Dunders: https://www.geeksforgeeks.org/customize-your-python-class-with-magic-or-dunder-methods/?ref=rpLinks to an external site. Then demonstrate your Dunders are used. mycode.py import re from urllib.request import urlopen from bs4 import BeautifulSoup from collections import defaultdict class WebCraping: def __init__(self): self.soup = None self.rows = [] self.dict = defaultdict(list) def openHtmlSite(self, htmlsite): html = urlopen(htmlsite) self.soup = BeautifulSoup(html, 'html.parser') # Children def children(self): [print(child.name) for child in self.soup.recursiveChildGenerator() if child.name is not None] #findAll def findAll(self, tags): dict = {} for tag in self.soup.find_all(tags): print("{0}: {1}".format(tag.name, tag.text)) r = re.compile(r'\s') s = r.split(tag.text) dict[s[0]] = s[1] for k, v in dict.items(): print('key= ', k, '\tvalue= ', v) # Append tag def appendTag(self, tag, nustr): newtag = self.soup.new_tag(tag) newtag.string = nustr ultag = self.soup.ul ultag.append(newtag) print(ultag.prettify()) # Inserting a new tag at position def insertAt(self, tag, nustr, index): newtag = self.soup.new_tag(tag) newtag.string = nustr ultag = self.soup.ul ultag.insert(index, newtag) print(ultag.prettify()) # Select list element def selectIndex(self, index): sel = "li:nth-of-type(" + str(index) + ")" print(self.soup.select(sel)) # Select paragraph def selectParagraph(self): spanElem = self.soup.select('p') print(spanElem) for i in range(0, len(spanElem)): print(str((spanElem)[i])) # Selects all span in given site def selectSpan(self): spanElem = self.soup.select('span') print(spanElem) for i in range(0, len(spanElem)): print(str((spanElem)[i])) # Find data we need: Country Name, data in 1980 and 2018 def find_data(self): self.dict = defaultdict() table = self.soup.find('table', class_='wikitable sortable') self.rows = table.find_all('tr') # Collect data for i in self.rows: # Find all info of td - table data in the site column = i.find_all('td') # Collect data and format them (country - strip(), number data - replace \xa0 and \n) if column: country = column[0].text.strip() year1 = column[1].text.replace('\xa0', '') year2 = column[14].text.replace('\n', '') # Store data in the default dict self.dict[country] = (year1, year2) # Print data def print_data(self): # Print collected data print("Production-based emissions: annual carbon dioxide emissions in metric tons per capita in 1980 and 2018") print("{:>46} {:>9} {:>10}".format("COUNTRY NAME", "1980", "2018")) for k, v in self.dict.items(): y1, y2 = v print(f'{k:>46}' + "{:>10} {:>10}".format(y1, y2)) # Print defaultdict def print_dict(self): print(self.dict) if __name__ == "__main__": openHtmlSite = WebCraping() openHtmlSite.openHtmlSite('https://en.wikipedia.org/wiki/List_of_countries_by_carbon_dioxide_emissions_per_capita') openHtmlSite.find_data() openHtmlSite.print_data() openHtmlSite.print_dict()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
To your code for Lab0, convert 3 of your functions to Dunders. Pick Dunders of your choice and note whether the Lab0 function you are replacing is binary or unary. An example of a unary Dunder is the __len__ and __add__ is binary. Click this link to get a Dunder list and more info about using Dunders:
https://www.geeksforgeeks.org/customize-your-python-class-with-magic-or-dunder-methods/?ref=rpLinks to an external site.
Then demonstrate your Dunders are used.
mycode.py
import re
from urllib.request import urlopen
from bs4 import BeautifulSoup
from collections import defaultdict
class WebCraping:
def __init__(self):
self.soup = None
self.rows = []
self.dict = defaultdict(list)
def openHtmlSite(self, htmlsite):
html = urlopen(htmlsite)
self.soup = BeautifulSoup(html, 'html.parser')
# Children
def children(self):
[print(child.name) for child in self.soup.recursiveChildGenerator() if child.name is not None]
#findAll
def findAll(self, tags):
dict = {}
for tag in self.soup.find_all(tags):
print("{0}: {1}".format(tag.name, tag.text))
r = re.compile(r'\s')
s = r.split(tag.text)
dict[s[0]] = s[1]
for k, v in dict.items():
print('key= ', k, '\tvalue= ', v)
# Append tag
def appendTag(self, tag, nustr):
newtag = self.soup.new_tag(tag)
newtag.string = nustr
ultag = self.soup.ul
ultag.append(newtag)
print(ultag.prettify())
# Inserting a new tag at position
def insertAt(self, tag, nustr, index):
newtag = self.soup.new_tag(tag)
newtag.string = nustr
ultag = self.soup.ul
ultag.insert(index, newtag)
print(ultag.prettify())
# Select list element
def selectIndex(self, index):
sel = "li:nth-of-type(" + str(index) + ")"
print(self.soup.select(sel))
# Select paragraph
def selectParagraph(self):
spanElem = self.soup.select('p')
print(spanElem)
for i in range(0, len(spanElem)):
print(str((spanElem)[i]))
# Selects all span in given site
def selectSpan(self):
spanElem = self.soup.select('span')
print(spanElem)
for i in range(0, len(spanElem)):
print(str((spanElem)[i]))
# Find data we need: Country Name, data in 1980 and 2018
def find_data(self):
self.dict = defaultdict()
table = self.soup.find('table', class_='wikitable sortable')
self.rows = table.find_all('tr')
# Collect data
for i in self.rows:
# Find all info of td - table data in the site
column = i.find_all('td')
# Collect data and format them (country - strip(), number data - replace \xa0 and \n)
if column:
country = column[0].text.strip()
year1 = column[1].text.replace('\xa0', '')
year2 = column[14].text.replace('\n', '')
# Store data in the default dict
self.dict[country] = (year1, year2)
# Print data
def print_data(self):
# Print collected data
print("Production-based emissions: annual carbon dioxide emissions in metric tons per capita in 1980 and 2018")
print("{:>46} {:>9} {:>10}".format("COUNTRY NAME", "1980", "2018"))
for k, v in self.dict.items():
y1, y2 = v
print(f'{k:>46}' + "{:>10} {:>10}".format(y1, y2))
# Print defaultdict
def print_dict(self):
print(self.dict)
if __name__ == "__main__":
openHtmlSite = WebCraping()
openHtmlSite.openHtmlSite('https://en.wikipedia.org/wiki/List_of_countries_by_carbon_dioxide_emissions_per_capita')
openHtmlSite.find_data()
openHtmlSite.print_data()
openHtmlSite.print_dict()
from urllib.request import urlopen
from bs4 import BeautifulSoup
from collections import defaultdict
class WebCraping:
def __init__(self):
self.soup = None
self.rows = []
self.dict = defaultdict(list)
def openHtmlSite(self, htmlsite):
html = urlopen(htmlsite)
self.soup = BeautifulSoup(html, 'html.parser')
# Children
def children(self):
[print(child.name) for child in self.soup.recursiveChildGenerator() if child.name is not None]
#findAll
def findAll(self, tags):
dict = {}
for tag in self.soup.find_all(tags):
print("{0}: {1}".format(tag.name, tag.text))
r = re.compile(r'\s')
s = r.split(tag.text)
dict[s[0]] = s[1]
for k, v in dict.items():
print('key= ', k, '\tvalue= ', v)
# Append tag
def appendTag(self, tag, nustr):
newtag = self.soup.new_tag(tag)
newtag.string = nustr
ultag = self.soup.ul
ultag.append(newtag)
print(ultag.prettify())
# Inserting a new tag at position
def insertAt(self, tag, nustr, index):
newtag = self.soup.new_tag(tag)
newtag.string = nustr
ultag = self.soup.ul
ultag.insert(index, newtag)
print(ultag.prettify())
# Select list element
def selectIndex(self, index):
sel = "li:nth-of-type(" + str(index) + ")"
print(self.soup.select(sel))
# Select paragraph
def selectParagraph(self):
spanElem = self.soup.select('p')
print(spanElem)
for i in range(0, len(spanElem)):
print(str((spanElem)[i]))
# Selects all span in given site
def selectSpan(self):
spanElem = self.soup.select('span')
print(spanElem)
for i in range(0, len(spanElem)):
print(str((spanElem)[i]))
# Find data we need: Country Name, data in 1980 and 2018
def find_data(self):
self.dict = defaultdict()
table = self.soup.find('table', class_='wikitable sortable')
self.rows = table.find_all('tr')
# Collect data
for i in self.rows:
# Find all info of td - table data in the site
column = i.find_all('td')
# Collect data and format them (country - strip(), number data - replace \xa0 and \n)
if column:
country = column[0].text.strip()
year1 = column[1].text.replace('\xa0', '')
year2 = column[14].text.replace('\n', '')
# Store data in the default dict
self.dict[country] = (year1, year2)
# Print data
def print_data(self):
# Print collected data
print("Production-based emissions: annual carbon dioxide emissions in metric tons per capita in 1980 and 2018")
print("{:>46} {:>9} {:>10}".format("COUNTRY NAME", "1980", "2018"))
for k, v in self.dict.items():
y1, y2 = v
print(f'{k:>46}' + "{:>10} {:>10}".format(y1, y2))
# Print defaultdict
def print_dict(self):
print(self.dict)
if __name__ == "__main__":
openHtmlSite = WebCraping()
openHtmlSite.openHtmlSite('https://en.wikipedia.org/wiki/List_of_countries_by_carbon_dioxide_emissions_per_capita')
openHtmlSite.find_data()
openHtmlSite.print_data()
openHtmlSite.print_dict()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
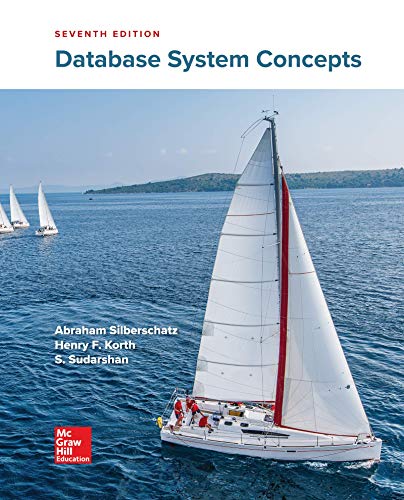
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
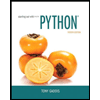
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
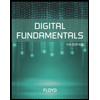
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
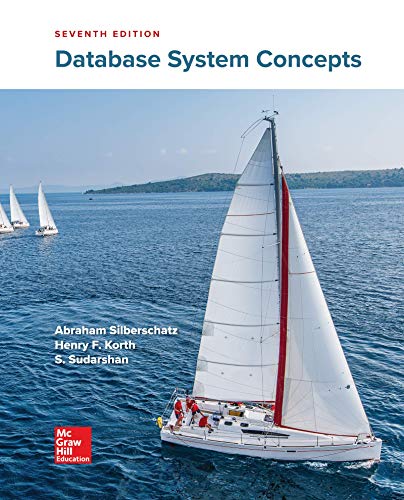
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
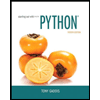
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
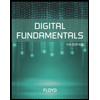
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
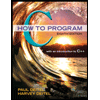
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
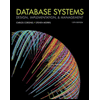
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
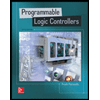
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education