I need help with this C assignment, I've been stuck on!! I have attached the assignment. It declares that the files merge.c, mergesort.h, and wrt.c CANNOT be changed Please follow the assignment to further complete all else thats required! Merge.c [Don't make changes] #include "mergesort.h" void merge(int a[], int b[], int c[], int m, int n) { int i = 0, j = 0, k = 0; /* Loop through both arrays and compare their elements to eachother */ while (i < m && j < n) if (a[i] < b[j]) c[k++] = a[i++]; else c[k++] = b[j++]; /* If there are any remaining elements in array b[]. copy them to array c[]. */ while (i < m) /* pick up any remainder */ c[k++] = a[i++]; /* If there are any remaining elements in array a[], copy them to array c[]. */ while (j < n) c[k++] = b[j++]; } Mergesort.h[Don't make changes] #include #include #include // This function merges two sorted arrys a and b into a sorted array c. // paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in b void merge(int a[], int b[], int c[], int m, int n); //This function sorts an array of integers using the merge sort algorithm //key: the array of integers to be sorted //n: the number of elements in the array void mergesort(int key[], int n); //This function writes an array of integers //key: the array of integers to be written //sz: the number of elements in the array void wrt(int key [], int sz); Wrt.c [Don't make changes] #include "mergesort.h" /* Print out the elements of an array of integers. key: the array to be printed. sz: the number of elements in the array. */ void wrt(int key[], int sz) { int i; for (i=0; i < sz; ++i) printf("%4d%s", key[i], ((i < sz - 1)? "" : "\n")); } [KINDLY, PLEASE Check the makefile, sort158a.c and merge.c if it needs change, please do so, make sure that the file will execute the "make" command and NO ERRORS DISPLAY] Ex. sort158a.c #include "mergesort.h" int main(void) { int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4, -5, 37, 7, 4, 2, 9, 1, -1 }; sz = sizeof(key) / sizeof(int); /* the size of key[] */ wrt(key, sz); /* Print the contents of the orginal arry */ mergesort(key, sz); /* Sort the array using mergesort */ printf("After mergesort:"\n); /*prints "After mergesort:"*/ wrt(key, sz); // Print the contents of the sorted array return 0; } MERGE.C #include "mergesort.h" void merge(int a[], int b[], int c[], int m, int n) { int i = 0, j = 0, k = 0; /* Loop through both arrays and compare their elements to eachother */ while (i < m && j < n) if (a[i] < b[j]) c[k++] = a[i++]; else c[k++] = b[j++]; /* If there are any remaining elements in array b[]. copy them to array c[]. */ while (i < m) /* pick up any remainder */ c[k++] = a[i++]; /* If there are any remaining elements in array a[], copy them to array c[]. */ while (i < m) /* pick up any remainder */ c[k++] = a[i++]; while (j < n) c[k++] = b[j++]; } MAKEFILE sort158: main.o merge.o mergesort.o wrt.o gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm main.o: main.c mergesort.h gcc -c main.c merge.o: merge.c mergesort.h gcc -c merge.c mergesort.o: mergesort.c mergesort.h gcc -c mergesort.c wrt.o: wrt.c gcc -c wrt.c clean: rm *.o sort158
I need help with this C assignment, I've been stuck on!!
I have attached the assignment.
It declares that the files merge.c, mergesort.h, and wrt.c CANNOT be changed
Please follow the assignment to further complete all else thats required!
Merge.c [Don't make changes]
#include "mergesort.h"
void merge(int a[], int b[], int c[], int m, int n)
{
int i = 0, j = 0, k = 0;
/* Loop through both arrays and compare their elements to eachother */
while (i < m && j < n)
if (a[i] < b[j])
c[k++] = a[i++];
else
c[k++] = b[j++];
/* If there are any remaining elements in array b[]. copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
/* If there are any remaining elements in array a[], copy them to array c[]. */
while (j < n)
c[k++] = b[j++];
}
Mergesort.h[Don't make changes]
#include <assert.h>
#include <stdio.h>
#include <stdlib.h>
// This function merges two sorted arrys a and b into a sorted array c.
// paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in b
void merge(int a[], int b[], int c[], int m, int n);
//This function sorts an array of integers using the merge sort
//key: the array of integers to be sorted
//n: the number of elements in the array
void mergesort(int key[], int n);
//This function writes an array of integers
//key: the array of integers to be written
//sz: the number of elements in the array
void wrt(int key [], int sz);
Wrt.c [Don't make changes]
#include "mergesort.h"
/* Print out the elements of an array of integers.
key: the array to be printed.
sz: the number of elements in the array. */
void wrt(int key[], int sz)
{
int i;
for (i=0; i < sz; ++i)
printf("%4d%s", key[i], ((i < sz - 1)? "" : "\n"));
}
[KINDLY, PLEASE Check the makefile, sort158a.c and merge.c if it needs change, please do so, make sure that the file will execute the "make" command and NO ERRORS DISPLAY]
Ex.
sort158a.c
#include "mergesort.h"
int main(void)
{
int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4,
-5, 37, 7, 4, 2, 9, 1, -1 };
sz = sizeof(key) / sizeof(int); /* the size of key[] */
wrt(key, sz); /* Print the contents of the orginal arry */
mergesort(key, sz); /* Sort the array using mergesort */
printf("After mergesort:"\n); /*prints "After mergesort:"*/
wrt(key, sz); // Print the contents of the sorted array
return 0;
}
MERGE.C
#include "mergesort.h"
void merge(int a[], int b[], int c[], int m, int n)
{
int i = 0, j = 0, k = 0;
/* Loop through both arrays and compare their elements to eachother */
while (i < m && j < n)
if (a[i] < b[j])
c[k++] = a[i++];
else
c[k++] = b[j++];
/* If there are any remaining elements in array b[]. copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
/* If there are any remaining elements in array a[], copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
while (j < n)
c[k++] = b[j++];
}
MAKEFILE
sort158: main.o merge.o mergesort.o wrt.o
gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm
main.o: main.c mergesort.h
gcc -c main.c
merge.o: merge.c mergesort.h
gcc -c merge.c
mergesort.o: mergesort.c mergesort.h
gcc -c mergesort.c
wrt.o: wrt.c
gcc -c wrt.c
clean:
rm *.o sort158

Step by step
Solved in 5 steps with 2 images

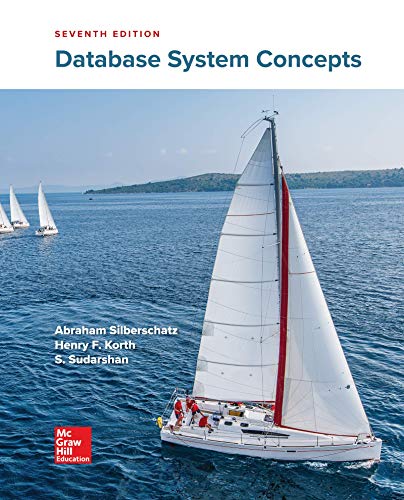
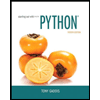
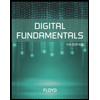
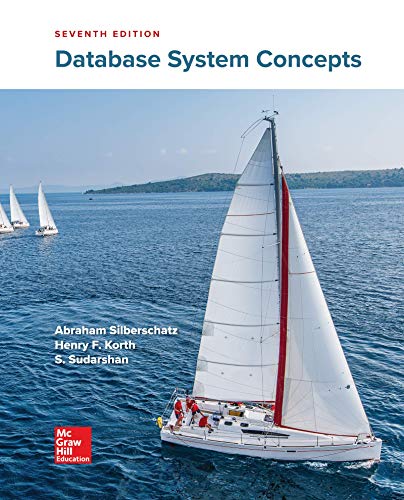
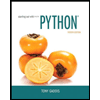
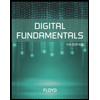
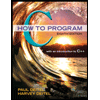
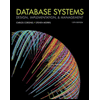
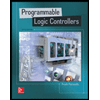