What can I fix about this code to program it to display ONLY duplicates instead of with non-duplicates? Prompt for reference: Write a console app that inserts 30 random letters into a List. Perform the following queries on the List and display your results: [Hint: Strings can be indexed like arrays to access a character at a specific index.] Use LINQ to sort the List in ascending order. Use LINQ to sort the List in descending order. Display list with ONLY duplicates using System; using System.Collections.Generic; using System.Linq; using System.Text;
What can I fix about this code to program it to display ONLY duplicates instead of with non-duplicates?
Prompt for reference:
Write a console app that inserts 30 random letters into a List<char>. Perform the following queries on the List and display your results: [Hint: Strings can be indexed like arrays to access a character at a specific index.]
Use LINQ to sort the List in ascending order.
Use LINQ to sort the List in descending order.
Display list with ONLY duplicates
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace List
{
class Program
{
static void Main(string[] args)
{
//Create a Random object
Random rand = new Random();
//Create a List of type to store 30 random values
List<char> list = new List<char>(30);
//Generate a random number and insert a character into the list
for (int i = 0; i < 30; i++)
{
int r = rand.Next(30) + 1;
//insert letters that starts from 'a'
list.Add((char)(r + 'a'));
}
Console.WriteLine("Before Sorting");
//Print before sorting
foreach (var item in list)
Console.WriteLine("{0}", item);
//Call OrderBy for each element in ascending order
List<char> sortedList = list.OrderBy(n => n).ToList();
Console.WriteLine("After ascending order sorting");
//Print ascnding sorted list
foreach (var item in sortedList )
Console.WriteLine("{0}", item);
//Call orderByDescending for each element
List<char> decendingList = list.OrderByDescending(n => n).ToList();
Console.WriteLine("After Descending order sorting");
//Print decending sorted list
foreach (var item in decendingList )
Console.WriteLine("{0}", item);
List<char> uniqueSortedList = sortedList.Distinct().ToList();
}
}
}

Step by step
Solved in 4 steps with 3 images

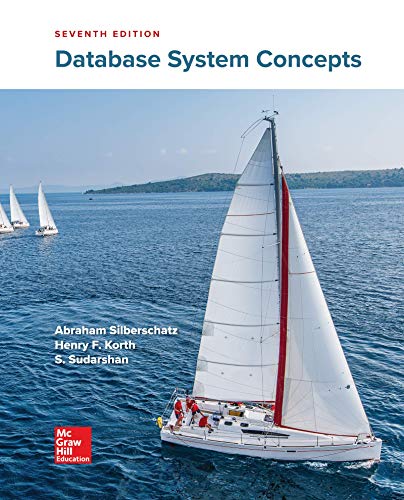
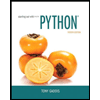
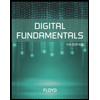
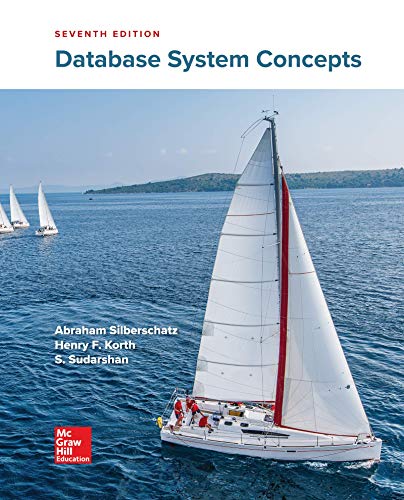
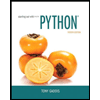
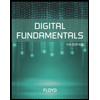
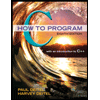
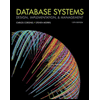
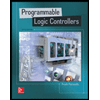