I need help with my MATLAB code. There is an error in the following code. The error says my orbitaldynamics function must return a column vector. Can you help me fix it? mu_earth = 398600.4418; % Earth's gravitational parameter (km^3/s^2) R_earth = 6378.137; % Earth's radius (km) C_d = 0.3; % Drag coefficient (assumed) A = 0.023; % Cross-sectional area of ISS (km^2) m = 420000; % Mass of ISS (kg) % Initial conditions: position and velocity (ISS state vector) % ISS initial state vector (km and km/s) - sample data state_ISS =[-2.1195e+03, 3.9866e+03, 5.0692e+03, -5.3489, -5.1772, 1.8324]; % Time span for 10 revolutions T_orbit = 2 * pi * sqrt((norm(state_ISS(1:3))^3) / mu_earth); time_span = [0, 10 * T_orbit]; % Step 3: Numerical integration using ODE solver options = odeset('RelTol', 1e-12, 'AbsTol', 1e-12); [t, state] = ode45(@orbitalDynamics, time_span, state_ISS, options); % Step 4: Plot the results figure; plot3(state(:, 1), state(:, 2), state(:, 3)); xlabel('X (km)'); ylabel('Y (km)'); zlabel('Z (km)'); title('ISS Orbit with Perturbations'); %% Functions function dstate_dt = orbitalDynamics(t, state) % Extract position and velocity from state vector r = state(1:3); % position vector (km) v = state(4:6); % velocity vector (km/s) % Compute Earth's gravitational acceleration r_norm = norm(r); mu_earth = 398600.4418; % Earth's gravitational parameter (km^3/s^2) a_earth = -mu_earth / r_norm^3 * r; r_sun = 1.0e+08 * [-1.3216, -0.6303, -0.2732]; r_moon = 1.0e+05 * [1.3924, 2.9851, 1.6094]; % Compute third-body perturbations (Sun, Moon) a_third_bodies = computeThirdBodyAcceleration(t, r, r_sun, r_moon); % Compute drag acceleration w = [0, 0, 7.272e-5]; v_rel = v - cross(w, r); rho = 8.19e-12; A = 0.023; m = 420000; C_d = 0.3; a_drag = -(C_d/2)*rho*(A/m)*dot(v_rel,v_rel)*(v_rel/norm(v_rel)); % Compute SRP acceleration S_unit = r_sun/norm(r_sun); a_srp = computeSRP(A, m, r, r_sun, C_d, S_unit); % Total acceleration a_total = a_earth + a_third_bodies + a_drag + a_srp; % Define the derivative of the state vector dstate_dt = [v, a_total]; end % Additional functions for third-body and SRP calculations function acc_third_bodies = computeThirdBodyAcceleration(t, r, r_sun, r_moon) % Compute third-body effects using SPICE/MICE functions % For simplicity, assume Sun and Moon as third-bodies mu_sun = 132712440018; % km^3/s^2 mu_moon = 4902.8; % km^3/s^2 % Accelerations due to Sun and Moon acc_sun = -mu_sun * ((r_sun - r) / norm(r_sun - r)^3 + r_sun / norm(r_sun)^3); acc_moon = -mu_moon * ((r_moon - r) / norm(r_moon - r)^3 + r_moon / norm(r_moon)^3); acc_third_bodies = acc_sun + acc_moon; end function a_srp = computeSRP(A, m, r, r_sun, C_d, S_unit) % Compute Solar Radiation Pressure (SRP) acceleration E = 1361; c = 299792.458; A_earth = 510072000; C_tilda = (1/4) + (1/9)*C_d; a_srp = -(A/m)*(E/c)*(A_earth^2/norm(r-r_sun)^2)*C_tilda*S_unit; end
I need help with my MATLAB code. There is an error in the following code. The error says my orbitaldynamics function must return a column vector. Can you help me fix it? mu_earth = 398600.4418; % Earth's gravitational parameter (km^3/s^2) R_earth = 6378.137; % Earth's radius (km) C_d = 0.3; % Drag coefficient (assumed) A = 0.023; % Cross-sectional area of ISS (km^2) m = 420000; % Mass of ISS (kg) % Initial conditions: position and velocity (ISS state vector) % ISS initial state vector (km and km/s) - sample data state_ISS =[-2.1195e+03, 3.9866e+03, 5.0692e+03, -5.3489, -5.1772, 1.8324]; % Time span for 10 revolutions T_orbit = 2 * pi * sqrt((norm(state_ISS(1:3))^3) / mu_earth); time_span = [0, 10 * T_orbit]; % Step 3: Numerical integration using ODE solver options = odeset('RelTol', 1e-12, 'AbsTol', 1e-12); [t, state] = ode45(@orbitalDynamics, time_span, state_ISS, options); % Step 4: Plot the results figure; plot3(state(:, 1), state(:, 2), state(:, 3)); xlabel('X (km)'); ylabel('Y (km)'); zlabel('Z (km)'); title('ISS Orbit with Perturbations'); %% Functions function dstate_dt = orbitalDynamics(t, state) % Extract position and velocity from state vector r = state(1:3); % position vector (km) v = state(4:6); % velocity vector (km/s) % Compute Earth's gravitational acceleration r_norm = norm(r); mu_earth = 398600.4418; % Earth's gravitational parameter (km^3/s^2) a_earth = -mu_earth / r_norm^3 * r; r_sun = 1.0e+08 * [-1.3216, -0.6303, -0.2732]; r_moon = 1.0e+05 * [1.3924, 2.9851, 1.6094]; % Compute third-body perturbations (Sun, Moon) a_third_bodies = computeThirdBodyAcceleration(t, r, r_sun, r_moon); % Compute drag acceleration w = [0, 0, 7.272e-5]; v_rel = v - cross(w, r); rho = 8.19e-12; A = 0.023; m = 420000; C_d = 0.3; a_drag = -(C_d/2)*rho*(A/m)*dot(v_rel,v_rel)*(v_rel/norm(v_rel)); % Compute SRP acceleration S_unit = r_sun/norm(r_sun); a_srp = computeSRP(A, m, r, r_sun, C_d, S_unit); % Total acceleration a_total = a_earth + a_third_bodies + a_drag + a_srp; % Define the derivative of the state vector dstate_dt = [v, a_total]; end % Additional functions for third-body and SRP calculations function acc_third_bodies = computeThirdBodyAcceleration(t, r, r_sun, r_moon) % Compute third-body effects using SPICE/MICE functions % For simplicity, assume Sun and Moon as third-bodies mu_sun = 132712440018; % km^3/s^2 mu_moon = 4902.8; % km^3/s^2 % Accelerations due to Sun and Moon acc_sun = -mu_sun * ((r_sun - r) / norm(r_sun - r)^3 + r_sun / norm(r_sun)^3); acc_moon = -mu_moon * ((r_moon - r) / norm(r_moon - r)^3 + r_moon / norm(r_moon)^3); acc_third_bodies = acc_sun + acc_moon; end function a_srp = computeSRP(A, m, r, r_sun, C_d, S_unit) % Compute Solar Radiation Pressure (SRP) acceleration E = 1361; c = 299792.458; A_earth = 510072000; C_tilda = (1/4) + (1/9)*C_d; a_srp = -(A/m)*(E/c)*(A_earth^2/norm(r-r_sun)^2)*C_tilda*S_unit; end
Elements Of Electromagnetics
7th Edition
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Sadiku, Matthew N. O.
ChapterMA: Math Assessment
Section: Chapter Questions
Problem 1.1MA
Related questions
Question
I need help with my MATLAB code. There is an error in the following code. The error says my orbitaldynamics function must return a column
mu_earth = 398600.4418; % Earth's gravitational parameter (km^3/s^2)
R_earth = 6378.137; % Earth's radius (km)
C_d = 0.3; % Drag coefficient (assumed)
A = 0.023; % Cross-sectional area of ISS (km^2)
m = 420000; % Mass of ISS (kg)
% Initial conditions: position and velocity (ISS state vector)
% ISS initial state vector (km and km/s) - sample data
state_ISS =[-2.1195e+03, 3.9866e+03, 5.0692e+03, -5.3489, -5.1772, 1.8324];
% Time span for 10 revolutions
T_orbit = 2 * pi * sqrt((norm(state_ISS(1:3))^3) / mu_earth);
time_span = [0, 10 * T_orbit];
% Step 3: Numerical integration using ODE solver
options = odeset('RelTol', 1e-12, 'AbsTol', 1e-12);
[t, state] = ode45(@orbitalDynamics, time_span, state_ISS, options);
% Step 4: Plot the results
figure;
plot3(state(:, 1), state(:, 2), state(:, 3));
xlabel('X (km)');
ylabel('Y (km)');
zlabel('Z (km)');
title('ISS Orbit with Perturbations');
%% Functions
function dstate_dt = orbitalDynamics(t, state)
% Extract position and velocity from state vector
r = state(1:3); % position vector (km)
v = state(4:6); % velocity vector (km/s)
% Compute Earth's gravitational acceleration
r_norm = norm(r);
mu_earth = 398600.4418; % Earth's gravitational parameter (km^3/s^2)
a_earth = -mu_earth / r_norm^3 * r;
r_sun = 1.0e+08 * [-1.3216, -0.6303, -0.2732];
r_moon = 1.0e+05 * [1.3924, 2.9851, 1.6094];
% Compute third-body perturbations (Sun, Moon)
a_third_bodies = computeThirdBodyAcceleration(t, r, r_sun, r_moon);
% Compute drag acceleration
w = [0, 0, 7.272e-5];
v_rel = v - cross(w, r);
rho = 8.19e-12;
A = 0.023;
m = 420000;
C_d = 0.3;
a_drag = -(C_d/2)*rho*(A/m)*dot(v_rel,v_rel)*(v_rel/norm(v_rel));
% Compute SRP acceleration
S_unit = r_sun/norm(r_sun);
a_srp = computeSRP(A, m, r, r_sun, C_d, S_unit);
% Total acceleration
a_total = a_earth + a_third_bodies + a_drag + a_srp;
% Define the derivative of the state vector
dstate_dt = [v, a_total];
end
% Additional functions for third-body and SRP calculations
function acc_third_bodies = computeThirdBodyAcceleration(t, r, r_sun, r_moon)
% Compute third-body effects using SPICE/MICE functions
% For simplicity, assume Sun and Moon as third-bodies
mu_sun = 132712440018; % km^3/s^2
mu_moon = 4902.8; % km^3/s^2
% Accelerations due to Sun and Moon
acc_sun = -mu_sun * ((r_sun - r) / norm(r_sun - r)^3 + r_sun / norm(r_sun)^3);
acc_moon = -mu_moon * ((r_moon - r) / norm(r_moon - r)^3 + r_moon / norm(r_moon)^3);
acc_third_bodies = acc_sun + acc_moon;
end
function a_srp = computeSRP(A, m, r, r_sun, C_d, S_unit)
% Compute Solar Radiation Pressure (SRP) acceleration
E = 1361;
c = 299792.458;
A_earth = 510072000;
C_tilda = (1/4) + (1/9)*C_d;
a_srp = -(A/m)*(E/c)*(A_earth^2/norm(r-r_sun)^2)*C_tilda*S_unit;
end
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
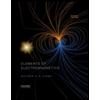
Elements Of Electromagnetics
Mechanical Engineering
ISBN:
9780190698614
Author:
Sadiku, Matthew N. O.
Publisher:
Oxford University Press
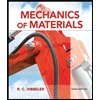
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:
9780134319650
Author:
Russell C. Hibbeler
Publisher:
PEARSON
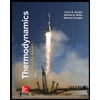
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:
9781259822674
Author:
Yunus A. Cengel Dr., Michael A. Boles
Publisher:
McGraw-Hill Education
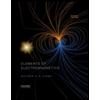
Elements Of Electromagnetics
Mechanical Engineering
ISBN:
9780190698614
Author:
Sadiku, Matthew N. O.
Publisher:
Oxford University Press
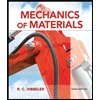
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:
9780134319650
Author:
Russell C. Hibbeler
Publisher:
PEARSON
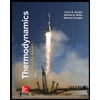
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:
9781259822674
Author:
Yunus A. Cengel Dr., Michael A. Boles
Publisher:
McGraw-Hill Education
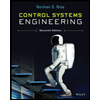
Control Systems Engineering
Mechanical Engineering
ISBN:
9781118170519
Author:
Norman S. Nise
Publisher:
WILEY
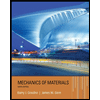
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:
9781337093347
Author:
Barry J. Goodno, James M. Gere
Publisher:
Cengage Learning
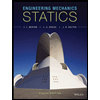
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:
9781118807330
Author:
James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:
WILEY