I need help with my BreakCheck java method that can be used in Linter program: Here's my code: BreakCheck class: import java.util.*; public class BreakCheck implements Check { public Optional lint(String line, int lineNumber) { // Match "break" outside and inside of comments or strings if (line.matches(".*\\bbreak\\b.*") && !line.matches("(?s).*\\s*//.*\\bbreak\\b.*")) { return Optional.of(new Error(2, lineNumber, "Line contains 'break' keyword outside of a comment")); } return Optional.empty(); } } My LinterMain Class: import java.util.*; import java.io.*; public class LinterMain { public static final String FILE_NAME = "TestFile.java"; public static void main(String[] args) throws FileNotFoundException{ List checks = new ArrayList<>(); checks.add(new LongLineCheck()); checks.add(new BlankPrintlnCheck()); checks.add(new BreakCheck()); Linter linter = new Linter(checks); List errors = linter.lint(FILE_NAME); for (Error e : errors) { System.out.println(e); } } } My Error Class: import java.util.*; public class Error { private final int code; private final int lineNumber; private final String message; public Error(int code, int lineNumber, String message) { this.code = code; this.lineNumber = lineNumber; this.message = message; } public int getLineNumber() { return lineNumber; } public int getCode() { return code; } public String getMessage() { return message; } public String toString() { return String.format("(Line: %d) has error code %d\n%s", lineNumber, code, message); } } Check Class: import java.util.*; // A common interface for linters that can check a single line of code // Every Check will check for its own type of error on a single line of code. public interface Check { // Checks for this Check's error condition on this line with this line number. // If an error exists on this line, returns an Optional with an Error present // indicating an error occurred. If no errors are present, returns an empty Optional. public Optional lint(String line, int lineNumber); } and This is a test class for testing the method: public class TestFile { public static void main(String[] args) { System.out.println("This is a really really really long line that should fail the long line check"); while (true) { System.out.println(""); /break; } } } Should return an error (with custom message) if the given line contains the break keyword outside of a single line comment (comments that start with //). i.e, we don't care about the word break inside comments, and only in the actual java code. Your check should only look for break specifically in all-lowercase (so occurrences of "Break" or "BReaK" outside of a single line comment should not be flagged). Note that this check is overly-simplistic in that it might flag some false uses of break such as System.out.println("break");. You do not need to handle this case specially; you should flag any use of the word break outside of a single line comment. Currently, /break is being ignored as error when it should not be ignored because it has / in front. This is the only error I encountered.
import java.util.*;
public class BreakCheck implements Check {
public Optional<Error> lint(String line, int lineNumber) {
// Match "break" outside and inside of comments or strings
if (line.matches(".*\\bbreak\\b.*") && !line.matches("(?s).*\\s*//.*\\bbreak\\b.*")) {
return Optional.of(new Error(2, lineNumber, "Line contains 'break' keyword outside of a comment"));
}
return Optional.empty();
}
}
My LinterMain Class:
import java.util.*;
import java.io.*;
public class LinterMain {
public static final String FILE_NAME = "TestFile.java";
public static void main(String[] args) throws FileNotFoundException{
List<Check> checks = new ArrayList<>();
checks.add(new LongLineCheck());
checks.add(new BlankPrintlnCheck());
checks.add(new BreakCheck());
Linter linter = new Linter(checks);
List<Error> errors = linter.lint(FILE_NAME);
for (Error e : errors) {
System.out.println(e);
}
}
}
My Error Class:
import java.util.*;
public class Error {
private final int code;
private final int lineNumber;
private final String message;
public Error(int code, int lineNumber, String message) {
this.code = code;
this.lineNumber = lineNumber;
this.message = message;
}
public int getLineNumber() {
return lineNumber;
}
public int getCode() {
return code;
}
public String getMessage() {
return message;
}
public String toString() {
return String.format("(Line: %d) has error code %d\n%s", lineNumber, code, message);
}
}
Check Class:
import java.util.*;
// A common interface for linters that can check a single line of code
// Every Check will check for its own type of error on a single line of code.
public interface Check {
// Checks for this Check's error condition on this line with this line number.
// If an error exists on this line, returns an Optional with an Error present
// indicating an error occurred. If no errors are present, returns an empty Optional.
public Optional<Error> lint(String line, int lineNumber);
}
and This is a test class for testing the method:
public class TestFile {
public static void main(String[] args) {
System.out.println("This is a really really really long line that should fail the long line check");
while (true) {
System.out.println("");
/break;
}
}
}
Should return an error (with custom message) if the given line contains the break keyword outside of a single line comment (comments that start with //). i.e, we don't care about the word break inside comments, and only in the actual java code. Your check should only look for break specifically in all-lowercase (so occurrences of "Break" or "BReaK" outside of a single line comment should not be flagged). Note that this check is overly-simplistic in that it might flag some false uses of break such as System.out.println("break");. You do not need to handle this case specially; you should flag any use of the word break outside of a single line comment.
Currently, /break is being ignored as error when it should not be ignored because it has / in front. This is the only error I encountered.


Step by step
Solved in 3 steps

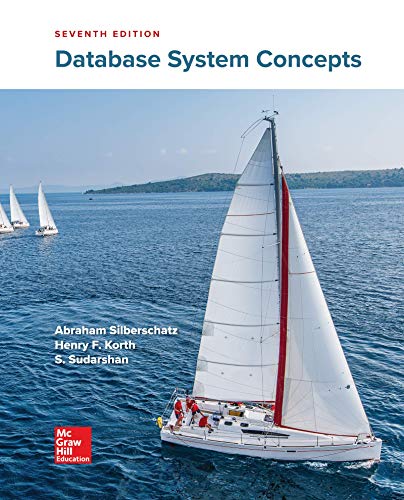
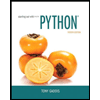
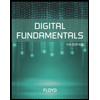
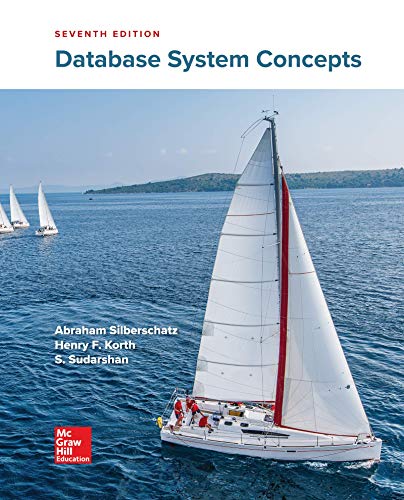
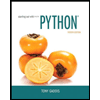
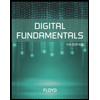
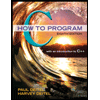
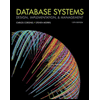
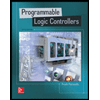