I need help: Please help me correct it the mistake I keep receiving this error: TestFrame1.java:29: error: count has private access in Frame System.out.println("Total number of frames created: " + frame.count); 1 error. Below is the code: import java.util.Scanner; public class TestFrame1 { // Scanner class instance private static Scanner scanner; // Main() public static void main(String[] args) { scanner = new Scanner(System.in); //make frame Frame frame = makeFrame(); //calc cost double cost = calcCost(frame); // Display frame attributes and cost System.out.println(frame); System.out.println(String.format("Total cost of frame: $%.2f", cost)); // Prompt user to buy another desk System.out.println("Do you want to buy another desk? (y/n)"); String input = scanner.next(); // If yes, then repeat above steps if (input.toLowerCase().equals("y")) { frame = makeFrame(); double cost2 = calcCost(frame); cost += cost2; System.out.println("Total number of frames created: " + frame.count); System.out.println(String.format("Total cost of pictures: $%.2f", cost)); System.out.println(String.format("Average picture cost: $%.2f",cost / 2)); } // End program System.out.println("See you later Alligator!"); } // Method to create a frame private static Frame makeFrame() { scanner = new Scanner(System.in); // Prompt user to enter length, width, type, matte System.out.print("Please enter the length: "); float length = scanner.nextFloat(); System.out.print("Please enter the width: "); float width = scanner.nextFloat(); System.out.print("Please enter the type: P, W or M "); char type = scanner.next().charAt(0); //convert type to uppercase Character.toUpperCase(type); // Validate type if (type != 'P' && type != 'W' && type != 'M') { type = 'W'; } System.out.print("Please enter the number of matte: "); int matte = scanner.nextInt(); // Create a Frame class object with all the values Frame frame = new Frame(length, width, type, matte); return frame; } // Method to calculate price of a picture public static double calcCost(Frame frame) { // Initialize price double cost = 0; // Store and check type of frame, update price accordingly char type = frame.getType(); if (type == 'P') { cost += 10.00; } else if (type == 'W') { cost += 22; } else {cost += 31;} // Store area of frame double area = frame.getArea(); // If area is greater than 100, update price if (area > 100) { cost += 12.50; } // If matte is greater than 5, add 5 if (frame.getMatte() > 5) { cost += 5 * 7.25; } //else add cost of matte else { cost += frame.getMatte() * 7.25; } // Return cost return cost; } }
I need help: Please help me correct it the mistake
I keep receiving this error:
TestFrame1.java:29: error: count has private access in Frame
System.out.println("Total number of frames created: " + frame.count);
1 error.
Below is the code:
import java.util.Scanner;
public class TestFrame1 {
// Scanner class instance
private static Scanner scanner;
// Main()
public static void main(String[] args) {
scanner = new Scanner(System.in);
//make frame
Frame frame = makeFrame();
//calc cost
double cost = calcCost(frame);
// Display frame attributes and cost
System.out.println(frame);
System.out.println(String.format("Total cost of frame: $%.2f", cost));
// Prompt user to buy another desk
System.out.println("Do you want to buy another desk? (y/n)");
String input = scanner.next();
// If yes, then repeat above steps
if (input.toLowerCase().equals("y")) {
frame = makeFrame();
double cost2 = calcCost(frame);
cost += cost2;
System.out.println("Total number of frames created: " + frame.count);
System.out.println(String.format("Total cost of pictures: $%.2f", cost));
System.out.println(String.format("Average picture cost: $%.2f",cost / 2));
}
// End program
System.out.println("See you later Alligator!");
}
// Method to create a frame
private static Frame makeFrame() {
scanner = new Scanner(System.in);
// Prompt user to enter length, width, type, matte
System.out.print("Please enter the length: ");
float length = scanner.nextFloat();
System.out.print("Please enter the width: ");
float width = scanner.nextFloat();
System.out.print("Please enter the type: P, W or M ");
char type = scanner.next().charAt(0);
//convert type to uppercase
Character.toUpperCase(type);
// Validate type
if (type != 'P' && type != 'W' && type != 'M') {
type = 'W';
}
System.out.print("Please enter the number of matte: ");
int matte = scanner.nextInt();
// Create a Frame class object with all the values
Frame frame = new Frame(length, width, type, matte);
return frame;
}
// Method to calculate price of a picture
public static double calcCost(Frame frame) {
// Initialize price
double cost = 0;
// Store and check type of frame, update price accordingly
char type = frame.getType();
if (type == 'P') {
cost += 10.00;
}
else if (type == 'W') {
cost += 22;
}
else {cost += 31;}
// Store area of frame
double area = frame.getArea();
// If area is greater than 100, update price
if (area > 100) {
cost += 12.50;
}
// If matte is greater than 5, add 5
if (frame.getMatte() > 5) {
cost += 5 * 7.25;
}
//else add cost of matte
else {
cost += frame.getMatte() * 7.25;
}
// Return cost
return cost;
}
}

Step by step
Solved in 4 steps with 2 images

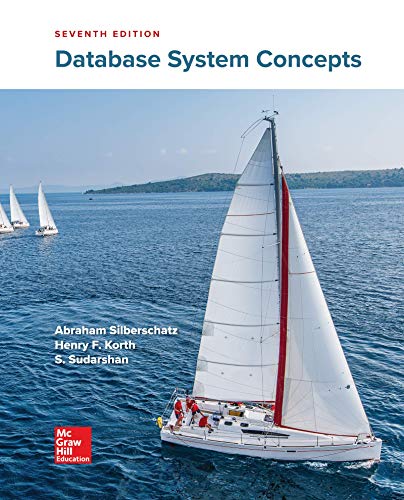
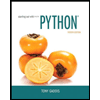
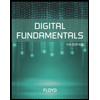
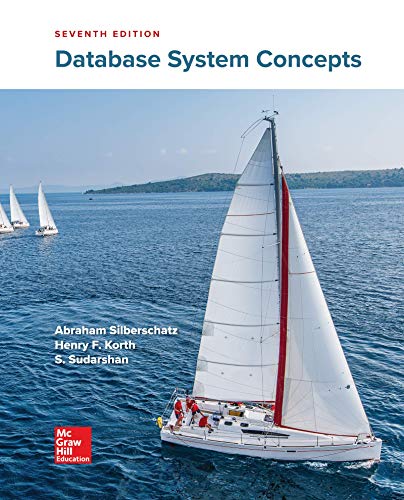
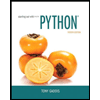
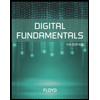
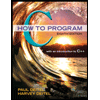
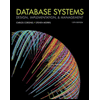
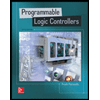