Rewrite the following code by removing Integer.parseInt
Please help with the following question:
Rewrite the following code by removing Integer.parseInt
public class javaapplication1 {
/**
* @param args
*
*/
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
//create 3 Department instances
System.out.println("Enter Department 1 name and ID: ");
Department d1=new Department(sc.nextLine(), Integer.parseInt ( sc.nextLine()));
System.out.println("Enter Department 2 name and ID: ");
Department d2=new Department(sc.nextLine(), Integer.parseInt( sc.nextLine()));
System.out.println("Enter Department 3 name and ID: ");
Department d3=new Department(sc.nextLine(), Integer.parseInt( sc.nextLine()));
//create 5 Students
System.out.println("Enter Student 1 Id, Name and Dept ID");
Student s1=new Student(Integer.parseInt(sc.nextLine()),sc.nextLine(),Integer.parseInt(sc.nextLine()));
System.out.println("Enter Student 2 Id, Name and Dept ID");
Student s2=new Student(Integer.parseInt(sc.nextLine()),sc.nextLine(),Integer.parseInt(sc.nextLine()));
System.out.println("Enter Student 3 Id, Name and Dept ID");
Student s3=new Student(Integer.parseInt(sc.nextLine()),sc.nextLine(),Integer.parseInt(sc.nextLine()));
System.out.println("Enter Student 4 Id, Name and Dept ID");
Student s4=new Student(Integer.parseInt(sc.nextLine()),sc.nextLine(),Integer.parseInt(sc.nextLine()));
System.out.println("Enter Student 5 Id, Name and Dept ID");
Student s5=new Student(Integer.parseInt(sc.nextLine()),sc.nextLine(),Integer.parseInt(sc.nextLine()));
//create University instance
University u1 = new University("Oxford",40000,2500000);
System.out.println("Student number 1 in Department 1: "+equals(s1.getDepId(),d1.getDepId()));
System.out.print("Input a String: ");
String str1=sc.nextLine(); //input string from user
String temp="";
for(int i=0;i<str1.length();i++){ //from 0 to last index
if(i%2==0) //if even position
temp+=Character.toLowerCase(str1.charAt(i)); //conavrt to lower case and add to temp
else //if odd position
temp+=Character.toUpperCase(str1.charAt(i)); //convert to upper case and add to temp
}
System.out.println(temp);
}
public static boolean equals(int n1,int n2){
return n1==n2; //return true if both are same else return false
}
}
public class University{
//declare the instance variables as private
private String name;
private int population;
private double budget;
//constructor for setting values
public University(String name, int population, double budget){
setName(name);
setPopulation(population);
setBudget(budget);
}
//setters
public void setName(String n){
name=n;
}
public void setPopulation(int p){
population=p;
}
public void setBudget(double b){
budget=b;
}
//getters
public String getName(){
return name;
}
public int getPopulation(){
return population;
}
public double getBudget(){
return budget;
}
}
public class Department{
//declaring instance variables
private String Dep_Name;
private int Dep_Id;
//constructor for setting instance variables
public Department(String name, int id){
Dep_Name=name;
Dep_Id=id;
}
//setters
public void setDepName(String name){
Dep_Name=name;
}
public void setDepId(int id){
Dep_Id=id;
}
//getters
public String getDepName(){
return Dep_Name;
}
public int getDepId(){
return Dep_Id;
}
}
public class Student{
//declaring instance variables
private int ID;
private String name;
private int Dep_Id;
//constructor for setting values to instance variables
public Student(int id, String n, int dep){
ID=id;
name=n;
Dep_Id=dep;
}
//setters
public void setID(int id){
ID=id;
}
public void setName(String n){
name=n;
}
public void setDepId(int dep){
Dep_Id=dep;
}
//getters
public int getID(){
return ID;
}
public String getName(){
return name;
}
public int getDepId(){
return Dep_Id;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

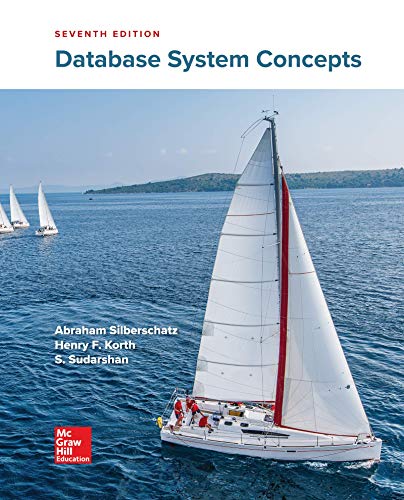
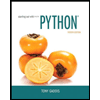
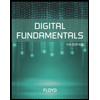
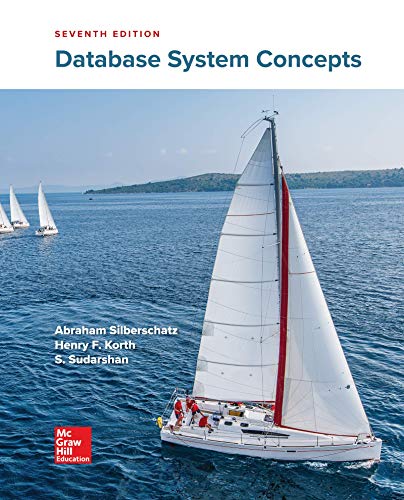
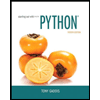
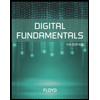
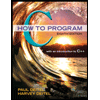
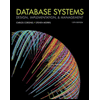
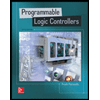