I need help editing this code I keep getting an error import java.util.Scanner; public class Factorial { public static void main(String[] args) { Scanner scan=new Scanner(System.in); System.out.print("Enter a number to find its factorial value : "); //read an integer value from keboard int num=Integer.parseInt(scan.nextLine()); //Calling fact method System.out.printf("Facorial of %d is %d ", num,fact(num)); } /*Recursive method, fact * Method name:fact * Input arguments:n as integer * Output arguments: an integer value * */ public static int fact(int n) { //Base case : //Return 1 if n value is either 0 or 1 if(n==0 || n==1) return 1; else //calling method, fact with n-1 return n*fact(n-1); }// }//
I need help editing this code I keep getting an error
import java.util.Scanner;
public class Factorial
{
public static void main(String[] args)
{
Scanner scan=new Scanner(System.in);
System.out.print("Enter a number to find its factorial value : ");
//read an integer value from keboard
int num=Integer.parseInt(scan.nextLine());
//Calling fact method
System.out.printf("Facorial of %d is %d ", num,fact(num));
}
/*Recursive method, fact
* Method name:fact
* Input arguments:n as integer
* Output arguments: an integer value
* */
public static int fact(int n)
{
//Base case :
//Return 1 if n value is either 0 or 1
if(n==0 || n==1)
return 1;
else
//calling method, fact with n-1
return n*fact(n-1);
}//
}//


Step by step
Solved in 3 steps with 1 images

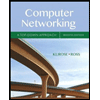
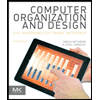
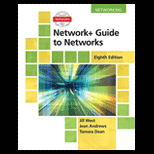
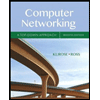
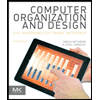
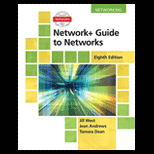
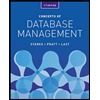
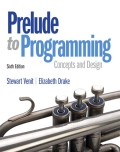
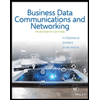