I need help annotating this code. def OR(a, b): if a == 1: return 1 elif b == 1: return 1 else: return 0 def NOR(a, b): if a == 0 and b == 0: return 1 elif a == 0 and b == 1: return 0 elif a == 1 and b == 0: return 0 elif a == 1 and b == 1: return 0 def AND(a, b): if a == 1 and b == 1: return 1 else: return 0 def logic_circuit(A,B,C): Q = OR(NOR(A,B),AND(B,C)) return(Q) A = int(input("Enter binary input For A: ")) B = int(input("Enter binary input For B: ")) C = int(input("Enter binary input For C: ")) Q = logic_circuit(A, B, C) print("Output: ", Q)
I need help annotating this code.
def OR(a, b):
if a == 1:
return 1
elif b == 1:
return 1
else:
return 0
def NOR(a, b):
if a == 0 and b == 0:
return 1
elif a == 0 and b == 1:
return 0
elif a == 1 and b == 0:
return 0
elif a == 1 and b == 1:
return 0
def AND(a, b):
if a == 1 and b == 1:
return 1
else:
return 0
def logic_circuit(A,B,C):
Q = OR(NOR(A,B),AND(B,C))
return(Q)
A = int(input("Enter binary input For A: "))
B = int(input("Enter binary input For B: "))
C = int(input("Enter binary input For C: "))
Q = logic_circuit(A, B, C)
print("Output: ", Q)

The annotations in Python are provided in 4 different manners:
- Annotations for simple parameters
- def foobar(a: expression, b: expression = 5):
- Annotations for excess parameters
- def foobar(*args: expression, *kwargs: expression):
- Annotations for nested parameters
- def foobar((a: expression, b: expression), (c: expression, d: expression)):
- Annotations for return type
- def foobar(a: expression)->expression:
# User defined function for logic OR
# The function takes two parameters and returns a single int
def OR(a: int, b: int)->int:
# If a is equal to 1 return 1
if a == 1 :
return 1
# If b is equal to 1 return 1
elif b == 1 :
return 1
# If a and b is equal to 0 return 0
else :
return 0
# User defined function for logic NOR
# The function takes two parameters and returns a single int
def NOR(a: int, b: int)->int:
# If a is equal to 0 and b is also equal to 0 return 1
if a == 0 and b == 0 :
return 1
# If a is equal to 0 and b is equal to 1 return 0
elif a == 0 and b == 1 :
return 0
# If a is equal to 1 and b is also equal to 0 return 0
elif a == 1 and b == 0 :
return 0
# If a is equal to 1 and b is also equal to 1 return 0
elif a == 1 and b == 1 :
return 0
# User defined function for logic AND
# The function takes two parameters and returns a single int
def AND(a: int, b: int)->int:
# Return 1 if both a and b are equal to 1
if a == 1 and b == 1 :
return 1
# Return 0 otherwise
else :
return 0
# User defined function for defining a logical circuit
# The function takes three parameters and returns a single int
def logic_circuit(A: int, B: int, C: int)->int:
# Calling above defined functions with
# parameters passed to them
# The function calls simulate a logic circuit
# The result is stored in a variable Q
# The value in Q is returned
Q = OR(NOR(A, B), AND(B, C))
return Q
# User inputs for bits A, B and C
A = int(input("Enter binary input For A: "))
B = int(input("Enter binary input For B: "))
C = int(input("Enter binary input For C: "))
# Calling function logic_circuit with A, B and C
# passed as parameters
# The result is stored in a variable Q
# The value of Q is returned
Q = logic_circuit(A, B, C)
print("Output: ", Q)
NOTE:
The definition for NOR(a, b) can be changed as follows:
# User defined function for logic NOR
# The function takes two parameters and returns a single int
def NOR(a: int, b: int)->int:
# If a is equal to 0 and b is also equal to 0 return 1
if a == 0 and b == 0 :
return 1
# Return 0 for all other value combination of a and b
else:
return 0
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

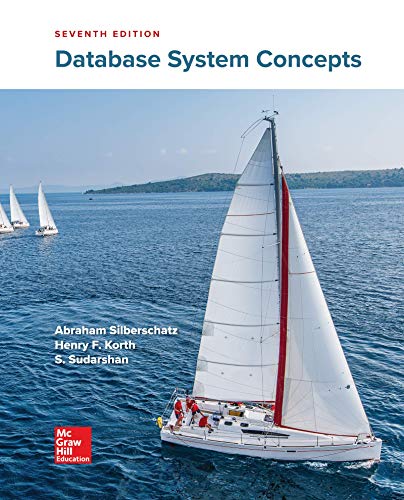
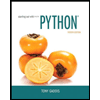
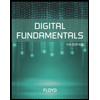
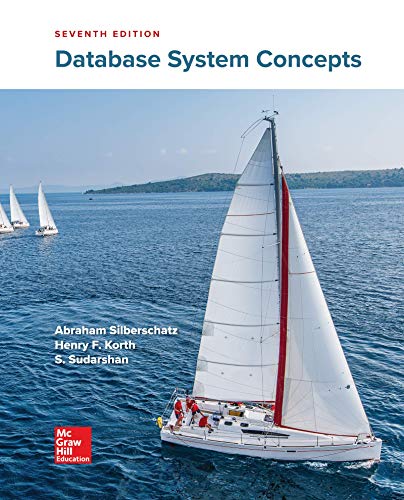
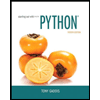
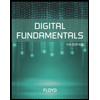
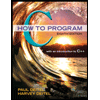
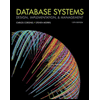
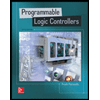