python: def ohno_twozero(placeholder, statement): """ Question 1 - Regex You are typing a personal statement but accidentally used the number 0 instead of the letter o (the two are very close on the keyboard). After realizing this, seeing the mistake once does not bother you, but you absolutely cannot tolerate seeing it twice in a row. Therefore, you will need to replace any WORD where '00' shows up at any point with the given placeholder. Do not replace any NUMBER where 00 shows up. (e.g. '100' should stay as '100' and not be replaced with the placeholder). You may assume that when '00' shows up in a word, there will be at least one letter preceding it. Return the output string after making these changes. THIS MUST BE DONE IN ONE LINE. Args: placeholder (str) statement (str) Returns: str >>> ohno_twozero('epic', "I am n0w l00king at 500 t0tal h0urs 0n Super Smash Br0s.") "I am n0w epic at 500 t0tal h0urs 0n Super Smash Br0s." >>> ohno_twozero('bruh', "And I w0uld have g0tten away with it t00 if it wasn't f0r all 200 0f y0u meddling kids!") "And I w0uld have g0tten away with it bruh if it wasn't f0r all 200 0f y0u meddling kids!" """ # pprint(ohno_twozero('epic', "I am n0w l00king at 500 t0tal h0urs 0n Super Smash Br0s.")) # pprint(ohno_twozero('bruh', "And I w0uld have g0tten away with it t00 if it wasn't f0r all 200 0f y0u meddling kids!"))
python:
def ohno_twozero(placeholder, statement):
"""
Question 1 - Regex
You are typing a personal statement but accidentally used the number 0 instead of the letter o (the two are very close on the keyboard).
After realizing this, seeing the mistake once does not bother you, but you absolutely cannot tolerate seeing it twice in a row.
Therefore, you will need to replace any WORD where '00' shows up at any point with the given placeholder.
Do not replace any NUMBER where 00 shows up. (e.g. '100' should stay as '100' and not be replaced with the placeholder).
You may assume that when '00' shows up in a word, there will be at least one letter preceding it.
Return the output string after making these changes.
THIS MUST BE DONE IN ONE LINE.
Args:
placeholder (str)
statement (str)
Returns:
str
>>> ohno_twozero('epic', "I am n0w l00king at 500 t0tal h0urs 0n Super Smash Br0s.")
"I am n0w epic at 500 t0tal h0urs 0n Super Smash Br0s."
>>> ohno_twozero('bruh', "And I w0uld have g0tten away with it t00 if it wasn't f0r all 200 0f y0u meddling kids!")
"And I w0uld have g0tten away with it bruh if it wasn't f0r all 200 0f y0u meddling kids!"
"""
# pprint(ohno_twozero('epic', "I am n0w l00king at 500 t0tal h0urs 0n Super Smash Br0s."))
# pprint(ohno_twozero('bruh', "And I w0uld have g0tten away with it t00 if it wasn't f0r all 200 0f y0u meddling kids!"))

The Python program for the given problem is as follows:
# Import the pprint module for calling pprint() function
import pprint
# Import the re module for using the regex function
import re
# Declare the function ohno_twozero(placeholder, statement) with two parameters placeholder and statement
def ohno_twozero(placeholder, statement):
return re.sub(r'\b\w*0{2}\w*\b(?<!\d)', placeholder, statement)
# Call and display ohno_twozero('epic', "I am n0w l00king at 500 t0tal h0urs 0n Super Smash Br0s.") function
pprint.pprint(ohno_twozero('epic', "I am n0w l00king at 500 t0tal h0urs 0n Super Smash Br0s."))
# Call and display ohno_twozero('bruh', "And I w0uld have g0tten away with it t00k if it wasn't f0r all 200 0f y0u meddling kids!") function
pprint.pprint(ohno_twozero('bruh', "And I w0uld have g0tten away with it t00k if it wasn't f0r all 200 0f y0u meddling kids!"))
Step by step
Solved in 2 steps with 2 images

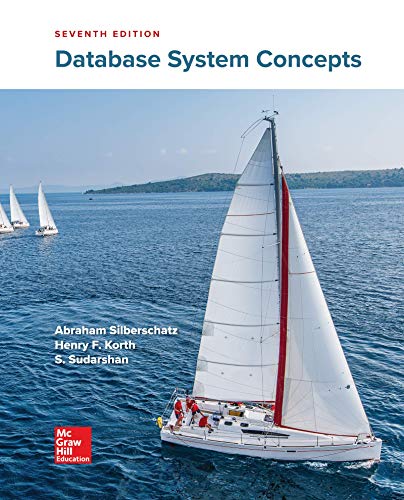
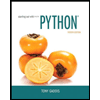
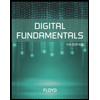
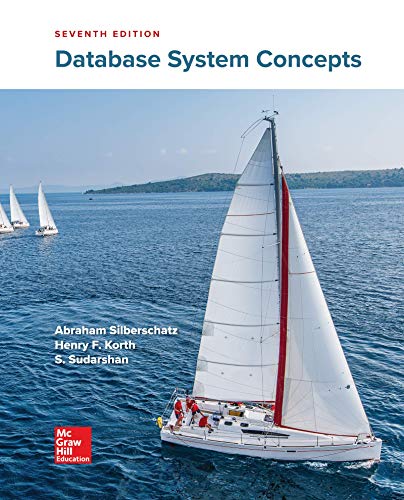
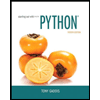
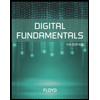
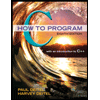
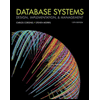
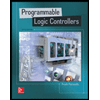