Correct and complete the following program. Hint: You may need to add a destructor. The output should be: ------------------------- BMW X5 1999 Ford Mustang 1969 Car: [Ford Mustang 1969] is deleted! Car: [BMW X5 1999] is deleted! ------------------------ #include using namespace std; class Car { string brand; string model; int year; string getModel() { return model; } string getBrand() { return brand; } int getYear() { return year; } } // Constructor definition outside the class Car::Car(string x, int y, int x) { brand = x; model = y; year = z; } int main() { // Create Car objects and call the constructor with different values Car carObj1(); Car carObj2("Ford", "Mustang"); // Print values cout << carObj1.brand << " " << carObj1.model << " " << carObj1.year << "\n"; cout << carObj2.brand << " " << carObj2.model << " " << carObj2.year << "\n"; return 0; }
Correct and complete the following program.
Hint: You may need to add a destructor.
The output should be:
-------------------------
BMW X5 1999
Ford Mustang 1969
Car: [Ford Mustang 1969] is deleted!
Car: [BMW X5 1999] is deleted!
------------------------
#include <iostream>
using namespace std;
class Car {
string brand;
string model;
int year;
string getModel() {
return model;
}
string getBrand() {
return brand;
}
int getYear() {
return year;
}
}
// Constructor definition outside the class
Car::Car(string x, int y, int x) {
brand = x;
model = y;
year = z;
}
int main() {
// Create Car objects and call the constructor with different values
Car carObj1();
Car carObj2("Ford", "Mustang");
// Print values
cout << carObj1.brand << " " << carObj1.model << " " << carObj1.year << "\n";
cout << carObj2.brand << " " << carObj2.model << " " << carObj2.year << "\n";
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

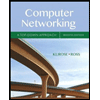
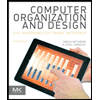
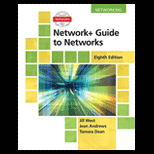
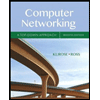
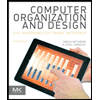
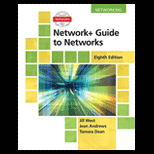
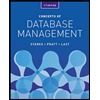
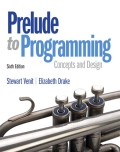
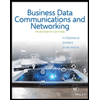