I need assistance fixing a program written in C++. It is supposed to open a file called "students.txt," which contains the name of 5 students, who each have 6 grade scores. It extracts the information from the file to create averages for each student. Each student is assigned a letter grade based on their averages. The student's name, letter grade, and average score is supposed to be written onto a new file called "studentsresults.txt" and displayed on the output monitor with cout. However when I run the program, I get weird values in both the created file and the output monitor, as seen in the image below. I wanted to know what is the issue and how to fix it. Here's the program: /* write a program to open a data file called "student.txt" which contains name of at least 5 students and 6 grades each. calculate average grade and letter grade of each and print them into file.(to another file)*/ #include #include using namespace std; int main() { ifstream in_stream; ofstream out_stream; char student_name[5][20]; int test_grades[6]; int i; int j; double average_grade = 0.0; char letter_grade; double total = 0.0; in_stream.open("students.txt"); out_stream.open("studentresults.txt"); for (i = 0; i < 5; i++) { in_stream >> student_name[i]; cout << i << " " << student_name[i] << endl; for (j = 0; j < 6; j++) { in_stream >> test_grades[j]; cout << i << " " << j << " " << test_grades[j] << endl; average_grade = test_grades[j] + average_grade; } average_grade = average_grade / 6; if (average_grade >= 90) { letter_grade = 'A'; } else if (average_grade >= 80) { letter_grade = 'B'; } else if (average_grade >= 70) { letter_grade = 'C'; } else if (average_grade >= 60) { letter_grade = 'D'; } else { letter_grade = 'F'; } cout << "Student: " << student_name[i] << "\tLetter Grade : " << letter_grade << "\tAverage grade:"; cout << average_grade << endl; out_stream << "Student: " << student_name << "\tLetter Grade : " << letter_grade << "\tAverage: " << average_grade << endl; } in_stream.close(); out_stream.close(); return 0; }
I need assistance fixing a program written in C++. It is supposed to open a file called "students.txt," which contains the name of 5 students, who each have 6 grade scores. It extracts the information from the file to create averages for each student. Each student is assigned a letter grade based on their averages.
The student's name, letter grade, and average score is supposed to be written onto a new file called "studentsresults.txt" and displayed on the output monitor with cout.
However when I run the program, I get weird values in both the created file and the output monitor, as seen in the image below. I wanted to know what is the issue and how to fix it.
Here's the program:
/*
write a program to open a data file called "student.txt" which contains name of at least
5 students and 6 grades each. calculate average grade and letter grade of each and print
them into file.(to another file)*/
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ifstream in_stream;
ofstream out_stream;
char student_name[5][20];
int test_grades[6];
int i;
int j;
double average_grade = 0.0;
char letter_grade;
double total = 0.0;
in_stream.open("students.txt");
out_stream.open("studentresults.txt");
for (i = 0; i < 5; i++)
{
in_stream >> student_name[i];
cout << i << " " << student_name[i] << endl;
for (j = 0; j < 6; j++)
{
in_stream >> test_grades[j];
cout << i << " " << j << " " << test_grades[j] << endl;
average_grade = test_grades[j] + average_grade;
}
average_grade = average_grade / 6;
if (average_grade >= 90)
{
letter_grade = 'A';
}
else if (average_grade >= 80)
{
letter_grade = 'B';
}
else if (average_grade >= 70)
{
letter_grade = 'C';
}
else if (average_grade >= 60)
{
letter_grade = 'D';
}
else
{
letter_grade = 'F';
}
cout << "Student: " << student_name[i] << "\tLetter Grade : " << letter_grade << "\tAverage grade:";
cout << average_grade << endl;
out_stream << "Student: " << student_name << "\tLetter Grade : " << letter_grade << "\tAverage: " << average_grade << endl;
}
in_stream.close();
out_stream.close();
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

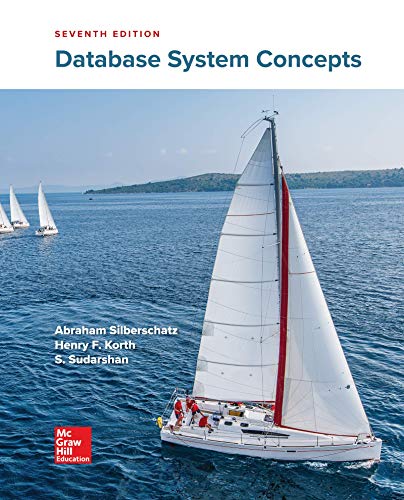
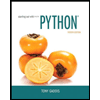
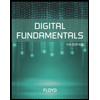
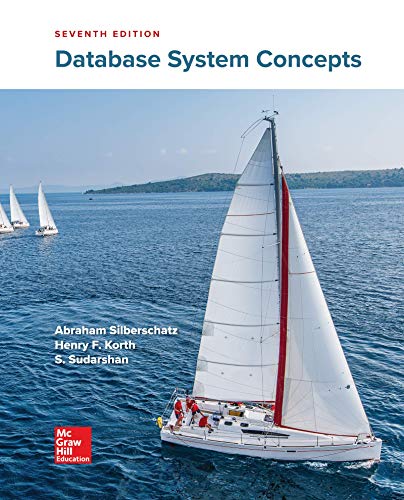
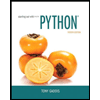
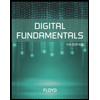
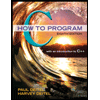
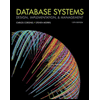
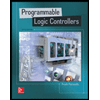