I have to use polymorphism and object oriented programming to write this code. Can someone please help me improve this code I have. The remaining code is in the pictures. Any help is appreciated. Thank You! The simulation should have 4 types of Passengers: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. The simulation should also have 4 types of Elevators: StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers. ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators. FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items. GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile items. Sample Input File Should look like this: # Building parameters floors=30 # Passengers to add to floors add_passenger 1 6 25 Standard 30 add_passenger 2 2 28 VIP 10 add_passenger 3 7 15 Freight 20 add_passenger 4 4 20 Glass 15 # Elevator types elevator_type StandardElevator 10 40 elevator_type ExpressElevator 8 25 elevator_type FreightElevator 5 20 elevator_type GlassElevator 6 15 # Percentage of passenger requests for each elevator type request_percentage StandardElevator 70 request_percentage ExpressElevator 20 request_percentage FreightElevator 5 request_percentage GlassElevator 5 # Percentage of passenger requests for each passenger type passenger_request_percentage Standard 70 passenger_request_percentage VIP 10 passenger_request_percentage Freight 15 passenger_request_percentage Glass 5 # Number of elevators in the system number_of_elevators 8 # Run simulation for 60 iterations run_simulation 60 The project should include these classes: public class SimulatorSettings { SimulatorSettings(String fileName){ } private int nofloors; public int getNofloors() { return nofloors; } public void setNofloors(int nofloors) { this.nofloors = nofloors; } } public abstract class Passenger { public static int passangerCounter = 0; private String passengerID; protected int startFloor; protected int endFloor; Passenger(){ this.passengerID = ""+passangerCounter; passangerCounter++; } public abstract boolean requestElevator(SimulatorSettings settings); } public class StandardPassenger extends Passenger{ private String type; public StandardPassenger() { } public boolean requestElevator(SimulatorSettings settings){ Random rand = new Random(); this.startFloor = rand.nextInt()% settings.getNofloors(); this.endFloor = rand.nextInt() % settings.getNofloors(); while(this.startFloor == this.endFloor){ this.endFloor = rand.nextInt() % settings.getNofloors(); } return true; } } public class VipPassenger extends Passenger { public VipPassenger() { super(); } @Override public boolean requestElevator(SimulatorSettings settings) { // Request an express elevator int expressElevator = settings.getNofloors() / 2; this.startFloor = expressElevator; this.endFloor = expressElevator; return true; } } public class FreightPassenger extends Passenger { public FreightPassenger() { super(); } @Override public boolean requestElevator(SimulatorSettings settings) { Random rand = new Random(); this.startFloor = rand.nextInt(settings.getNofloors()); this.endFloor = rand.nextInt(settings.getNofloors()); while (this.startFloor == this.endFloor) { this.endFloor = rand.nextInt(settings.getNofloors()); } return true; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Help, I making a elevator simulator. I have to use polymorphism and object oriented programming to write this code. Can someone please help me improve this code I have. The remaining code is in the pictures. Any help is appreciated. Thank You!
The simulation should have 4 types of Passengers:
Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators.
The simulation should also have 4 types of Elevators:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile items.
Sample Input File Should look like this:
# Building parameters
floors=30
# Passengers to add to floors
add_passenger 1 6 25 Standard 30
add_passenger 2 2 28 VIP 10
add_passenger 3 7 15 Freight 20
add_passenger 4 4 20 Glass 15
# Elevator types
elevator_type StandardElevator 10 40
elevator_type ExpressElevator 8 25
elevator_type FreightElevator 5 20
elevator_type GlassElevator 6 15
# Percentage of passenger requests for each elevator type
request_percentage StandardElevator 70
request_percentage ExpressElevator 20
request_percentage FreightElevator 5
request_percentage GlassElevator 5
# Percentage of passenger requests for each passenger type
passenger_request_percentage Standard 70
passenger_request_percentage VIP 10
passenger_request_percentage Freight 15
passenger_request_percentage Glass 5
# Number of elevators in the system
number_of_elevators 8
# Run simulation for 60 iterations
run_simulation 60
The project should include these classes:
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
public abstract class Passenger {
public static int passangerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger(){
this.passengerID = ""+passangerCounter;
passangerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public class StandardPassenger extends Passenger{
private String type;
public StandardPassenger() {
}
public boolean requestElevator(SimulatorSettings settings){
Random rand = new Random();
this.startFloor = rand.nextInt()% settings.getNofloors();
this.endFloor = rand.nextInt() % settings.getNofloors();
while(this.startFloor == this.endFloor){
this.endFloor = rand.nextInt() % settings.getNofloors();
}
return true;
}
}
public class VipPassenger extends Passenger {
public VipPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
// Request an express elevator
int expressElevator = settings.getNofloors() / 2;
this.startFloor = expressElevator;
this.endFloor = expressElevator;
return true;
}
}
public class FreightPassenger extends Passenger {
public FreightPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}



Step by step
Solved in 3 steps with 1 images

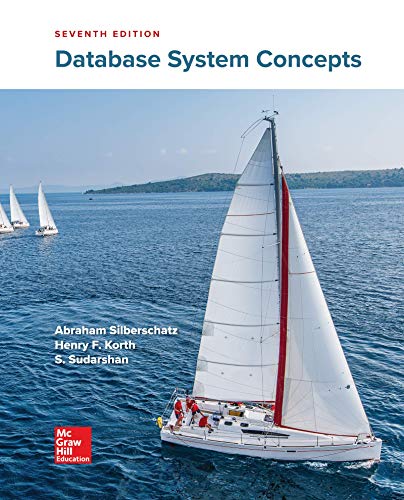
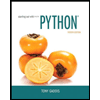
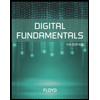
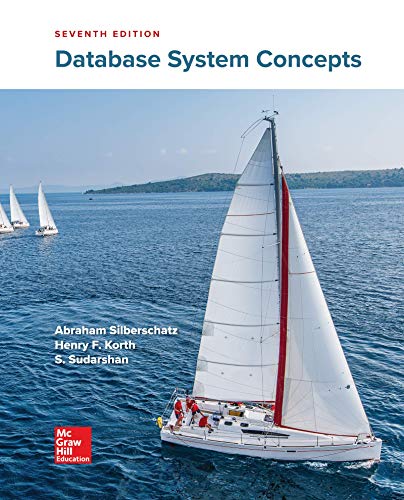
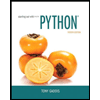
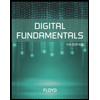
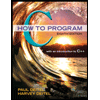
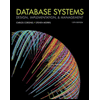
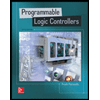