I am trying to execute the backward pass for a convolution network with layers (2 layer conv, relu, max pooling and linear, then softmax loss calculation). I have a hard time to retrieve x, y and probs at backward pass, the code does not recognize them as attributes. Please fix my code and show codes and output. Thank you
I am trying to execute the backward pass for a convolution network with layers (2 layer conv, relu, max pooling and linear, then softmax loss calculation). I have a hard time to retrieve x, y and probs at backward pass, the code does not recognize them as attributes. Please fix my code and show codes and output. Thank you
from .softmax_ce import SoftmaxCrossEntropy
from .relu import ReLU
from .max_pool import MaxPooling
from .convolution import Conv2D
from .linear import Linear
class ConvNet:
"""
Max Pooling of input
"""
def __init__(self, modules, criterion):
self.modules = []
for m in modules:
if m['type'] == 'Conv2D':
self.modules.append(
Conv2D(m['in_channels'],
m['out_channels'],
m['kernel_size'],
m['stride'],
m['padding'])
)
elif m['type'] == 'ReLU':
self.modules.append(
ReLU()
)
elif m['type'] == 'MaxPooling':
self.modules.append(
MaxPooling(m['kernel_size'],
m['stride'])
)
elif m['type'] == 'Linear':
self.modules.append(
Linear(m['in_dim'],
m['out_dim'])
)
if criterion['type'] == 'SoftmaxCrossEntropy':
self.criterion = SoftmaxCrossEntropy()
else:
raise ValueError("Wrong Criterion Passed")
def forward(self, x, y):
"""
The forward pass of the model
:param x: input data: (N, C, H, W)
:param y: input label: (N, )
:return:
probs: the probabilities of all classes: (N, num_classes)
loss: the cross entropy loss
"""
probs = None
loss = None
for i,m in enumerate(self.modules):
if i==0:
x=m.forward(x)
else:
x=m.forward(x)
loss=self.criterion.forward(x,y)
probs=x
return probs, loss
def backward(self):
"""
The backward pass of the model
:return: nothing but dx, dw, and db of all modules are updated
"""
for i, m in enumerate(self.modules):
if i==0:
probs, loss = self.criterion.forward(x,y)
dx = self.criterion.backward(probs,y)
if i ==1:
dx, dw, db = self.modules[-1-i].backward(dx)
else:
dx, dw, db = self.modules[-1-i].backward(dx)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

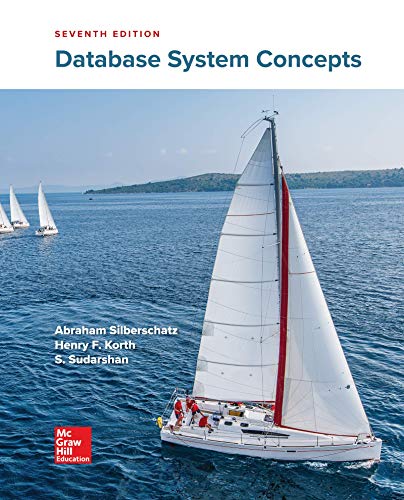
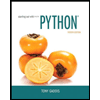
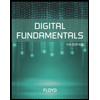
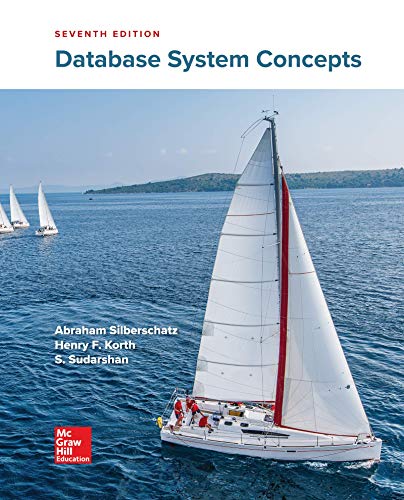
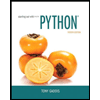
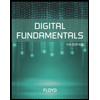
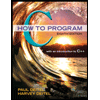
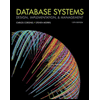
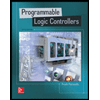