Write a Python program that assists you in managing a network of devices for your company. You need to keep track of the IP addresses, MAC addresses, and device types in your network. List: Create a list named device_ip containing IP addresses as strings. Your network consists of one router and at least one of each of the following devices: switch, server, workstation, printer, and mobile device. Each device should have a unique IP address. The router's IP address should be '192.168.1.1'. Print each IP address on a new line. Tuple: Since MAC addresses of devices are unique and not changing, you decide to store them in a tuple named device_mac. Create this tuple with MAC addresses corresponding to each IP address. A MAC address looks like '00:14:22:01:23:45'. Print each MAC address on a new line. Set: You also want to keep track of the different types of devices in your network. As the device types are distinct and do not have duplicates, create a set named device_type that contains the types of devices you have in your network. These include 'router', 'switch', 'server', 'workstation', 'printer', and 'mobile device'. Print each device type on a new line. Print each device type on a new line. List and Dictionary: Create a list named device_types. This list should contain the device type for each device corresponding to the IP address in your device_ip list and the MAC address in your device_mac tuple. Ensure that 'router' is the first device type in this list, and that the list contains at least one of each other device type. Note: We are creating an additional list named device_types to manage the mapping of device types to their corresponding IP and MAC addresses. This ensures that there's only one router and at least one of each other device type in the network. Now, create a dictionary named network_inventory. The dictionary’s keys should be the IP addresses from your device_ip list. Each value in the dictionary will itself be a dictionary with two keys: 'MAC' and 'Type'. The 'MAC' key corresponds to the MAC address from device_mac, and 'Type' corresponds to a device type from your device_types list. Ensure that the device with the IP '192.168.1.1' is assigned the 'router' type. Print each key-value pair on a new line. When your program runs, it should match the expected output below.
Write a Python program that assists you in managing a network of devices for your company. You need to keep track of the IP addresses, MAC addresses, and device types in your network. List: Create a list named device_ip containing IP addresses as strings. Your network consists of one router and at least one of each of the following devices: switch, server, workstation, printer, and mobile device. Each device should have a unique IP address. The router's IP address should be '192.168.1.1'. Print each IP address on a new line. Tuple: Since MAC addresses of devices are unique and not changing, you decide to store them in a tuple named device_mac. Create this tuple with MAC addresses corresponding to each IP address. A MAC address looks like '00:14:22:01:23:45'. Print each MAC address on a new line. Set: You also want to keep track of the different types of devices in your network. As the device types are distinct and do not have duplicates, create a set named device_type that contains the types of devices you have in your network. These include 'router', 'switch', 'server', 'workstation', 'printer', and 'mobile device'. Print each device type on a new line. Print each device type on a new line. List and Dictionary: Create a list named device_types. This list should contain the device type for each device corresponding to the IP address in your device_ip list and the MAC address in your device_mac tuple. Ensure that 'router' is the first device type in this list, and that the list contains at least one of each other device type. Note: We are creating an additional list named device_types to manage the mapping of device types to their corresponding IP and MAC addresses. This ensures that there's only one router and at least one of each other device type in the network. Now, create a dictionary named network_inventory. The dictionary’s keys should be the IP addresses from your device_ip list. Each value in the dictionary will itself be a dictionary with two keys: 'MAC' and 'Type'. The 'MAC' key corresponds to the MAC address from device_mac, and 'Type' corresponds to a device type from your device_types list. Ensure that the device with the IP '192.168.1.1' is assigned the 'router' type. Print each key-value pair on a new line. When your program runs, it should match the expected output below.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- Write a Python program that assists you in managing a network of devices for your company. You need to keep track of the IP addresses, MAC addresses, and device types in your network.
- List: Create a list named device_ip containing IP addresses as strings. Your network consists of one router and at least one of each of the following devices: switch, server, workstation, printer, and mobile device. Each device should have a unique IP address. The router's IP address should be '192.168.1.1'.
- Print each IP address on a new line.
- Tuple: Since MAC addresses of devices are unique and not changing, you decide to store them in a tuple named device_mac. Create this tuple with MAC addresses corresponding to each IP address. A MAC address looks like '00:14:22:01:23:45'.
- Print each MAC address on a new line.
- Set: You also want to keep track of the different types of devices in your network. As the device types are distinct and do not have duplicates, create a set named device_type that contains the types of devices you have in your network. These include 'router', 'switch', 'server', 'workstation', 'printer', and 'mobile device'. Print each device type on a new line.
- Print each device type on a new line.
- List and Dictionary: Create a list named device_types. This list should contain the device type for each device corresponding to the IP address in your device_ip list and the MAC address in your device_mac tuple. Ensure that 'router' is the first device type in this list, and that the list contains at least one of each other device type.
- Note: We are creating an additional list named device_types to manage the mapping of device types to their corresponding IP and MAC addresses. This ensures that there's only one router and at least one of each other device type in the network.
- Now, create a dictionary named network_inventory. The dictionary’s keys should be the IP addresses from your device_ip list. Each value in the dictionary will itself be a dictionary with two keys: 'MAC' and 'Type'. The 'MAC' key corresponds to the MAC address from device_mac, and 'Type' corresponds to a device type from your device_types list. Ensure that the device with the IP '192.168.1.1' is assigned the 'router' type.
- Print each key-value pair on a new line.
- When your program runs, it should match the expected output below.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I am getting an error that my list index is out of range, I have to have 8 ip addresses so there are 2 types that get used twice. Could this be why my index is out of range for the dictionary?
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
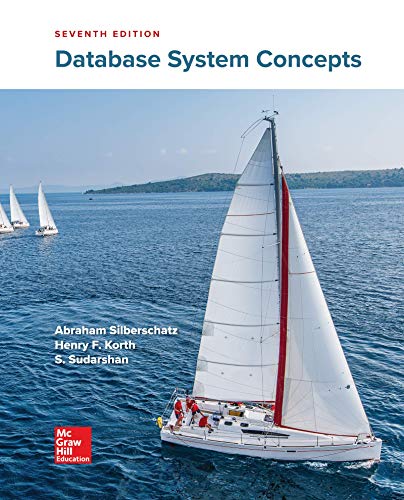
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
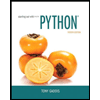
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
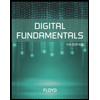
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
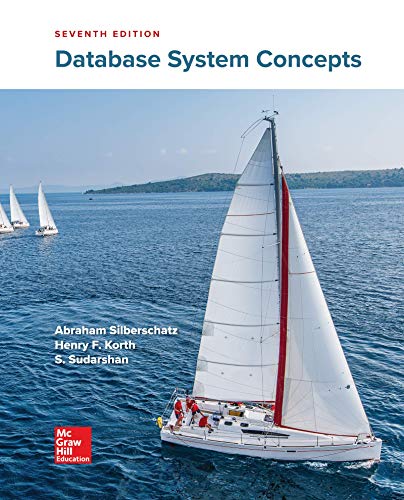
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
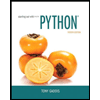
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
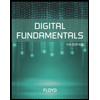
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
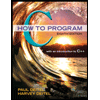
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
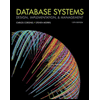
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
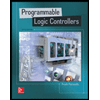
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education