How do I Write a Python program that can convert a Fahrenheit temperature to Celsius, or vice versa. The program should use two custom functions, f_to_c and c_to_f, to perform the conversions. Both of these functions should be defined in a custom module named temps. Custom function c_to_f should be a void function defined to take a Celsius temperature as a parameter. It should calculate and print the equivalent Fahrenheit temperature accurate to three decimal places. Custom function f_to_c should be a value-returning function defined to take a Fahrenheit temperature as a parameter. This function should calculate the equivalent Celsius temperature and return it.
How do I Write a Python program that can convert a Fahrenheit temperature to Celsius, or vice versa. The program should use two custom functions, f_to_c and c_to_f, to perform the conversions. Both of these functions should be defined in a custom module named temps. Custom function c_to_f should be a void function defined to take a Celsius temperature as a parameter. It should calculate and print the equivalent Fahrenheit temperature accurate to three decimal places. Custom function f_to_c should be a value-returning function defined to take a Fahrenheit temperature as a parameter. This function should calculate the equivalent Celsius temperature and return it.

1. Create a custom module to convert Fahrenheit to Celsius. This should be a void method. Add print statement after conversion.
2. Create a custom module to convert Celsius to Fahrenheit. Return the converted value.
3. Using Precision Handling in Python to print Fahrenheit temperature accurate to three decimal places
def c_to_f(C):
a= ((9/5)*C)+32
print(C,"Celsius is equal to %.3f Fahrenheit"%a)
def f_to_c(F):
return (5/9)*(F-32)
temp = float(input("Enter a temperature: "))
choice = input("Is the Unit Fahrenheit or Celsius. c/f?: ")
if choice=="c":
c_to_f(temp)
else:
print(temp,"Fahrenheit is equal to ",f_to_c(temp),"Celsius")
Step by step
Solved in 3 steps with 1 images

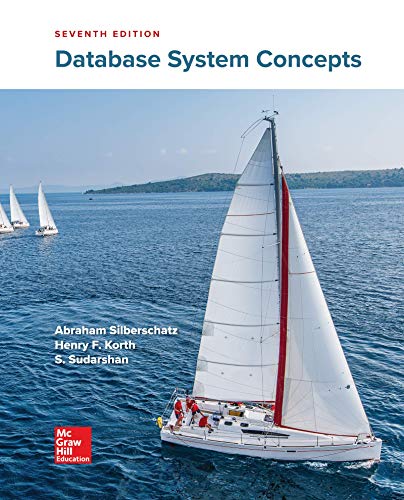
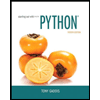
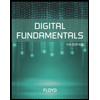
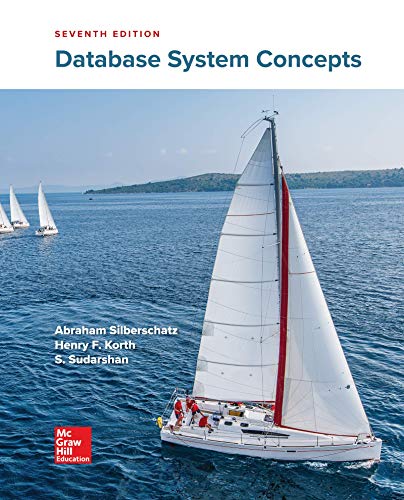
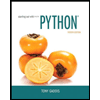
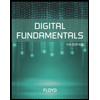
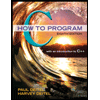
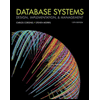
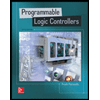