Here is the error I am getting: line 19, in from functions import (convertpd, convertdp) ModuleNotFoundError: No module named 'functions' I am not sure what I am doing with this… here is my code #defining the method convertpd to convert Pounds to Dollars def convertpd(dollars): #Returns the converted values return dollars*1.26634 #defining the method convertdp to convert Dollars to Pounds def convertdp(pounds): #Returns the converted values return pounds/1.26634 #importing the functions from functions import (convertpd, convertdp) #defining the main function def main(): #Prompts the user to enter the value to convert value = float(input('Enter the value to convert: ')) #Prompts the user to enter the choice choice = input('Enter D or P to convert entered values to Pounds(s) or Dollars(s): ') #if the user enters D as choice if choice == 'D': #Calling the convertpd method and displaying the converted value print('The value', value,'Pounds(s) converts to',round(convertpd(value),2),'Dollars(s).') #if user enters P as choice elif choice == 'P': #Calling the convertdp method and displaying the converted value print('The value', value,'Dollars(s) converts to',round(convertdp(value),2),'Pounds(s).') #Calling the main function main()
Create a custom Python module named functions.py. This module must contain two functions: one that converts from Dollars to Pounds and one that converts from Pounds to Dollars. These functions must be named convertdp ( used when P is selected ) and convertpd ( used when D is selected ).
These 2 functions are to return a single full precision float value, all print statements are to be in program53. Use 1.26634 for the conversion factor, example 1 Pound currency would be equivalent to 1.27 Dollars
Write a program named program53.py , which imports the functions from module functions.py. The program's main() should prompt the user to specify D or P as choices then call the appropriate function which was imported. Conversion accuracy should use full precision ( 5 decimal places as provided above ) for the calculations , and display then print the result to two decimal places.
Use the import keyword: import functions from * . The call the functions, would be functionname()
Output should look like this:
Enter the value to covert: 25.5
Enter D or P to convert entered value to Pound(s) or Dollars(s) : D
The Value 25.5 Pounds(s) converts to 32.29 Dollar(s)
Enter the value to covert: 25.5
Enter D or P to convert entered value to Pound(s) or Dollars(s) : P
The Value 25.5 Dollars(s) converts to 20.14 Pound(s)
Here is the error I am getting:
line 19, in <module>
from functions import (convertpd, convertdp)
ModuleNotFoundError: No module named 'functions'
I am not sure what I am doing with this… here is my code
#defining the method convertpd to convert Pounds to Dollars
def convertpd(dollars):
#Returns the converted values
return dollars*1.26634
#defining the method convertdp to convert Dollars to Pounds
def convertdp(pounds):
#Returns the converted values
return pounds/1.26634
#importing the functions
from functions import (convertpd, convertdp)
#defining the main function
def main():
#Prompts the user to enter the value to convert
value = float(input('Enter the value to convert: '))
#Prompts the user to enter the choice
choice = input('Enter D or P to convert entered values to Pounds(s) or Dollars(s): ')
#if the user enters D as choice
if choice == 'D':
#Calling the convertpd method and displaying the converted value
print('The value', value,'Pounds(s) converts to',round(convertpd(value),2),'Dollars(s).')
#if user enters P as choice
elif choice == 'P':
#Calling the convertdp method and displaying the converted value
print('The value', value,'Dollars(s) converts to',round(convertdp(value),2),'Pounds(s).')
#Calling the main function
main()

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

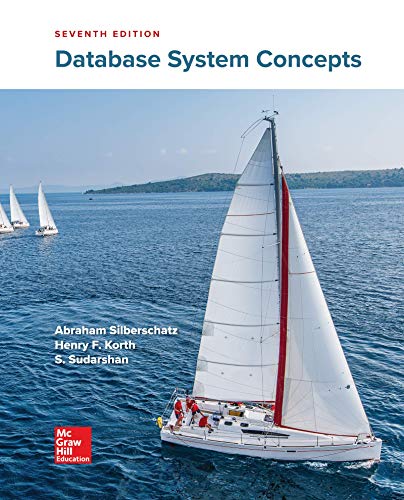
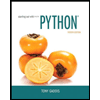
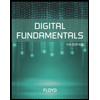
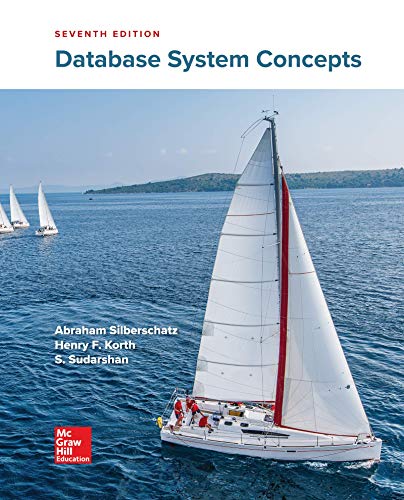
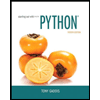
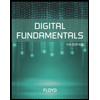
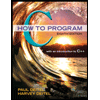
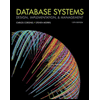
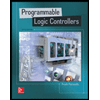