how do i get the main method to work? this is to return an binary tree from lowest to highest in java
how do i get the main method to work?
this is to return an binary tree from lowest to highest
in java
import java.util.*;
import java.io.*;
public class BinarySearchTree {
private int root;
private int free;
private int left[];
private int data[];
private int right[];
private int currentSize;
public BinarySearchTree(int size) {
root = -1;
free =-1;
left = new int[size];
data = new int[size];
right = new int[size];
currentSize = 0;
}
// constructor for initialise the root to null BYDEFAULT
public boolean full() {
return currentSize == data.length;
}
private int createNode(int value){
data[currentSize]=value;
left[currentSize]=-1;
right[currentSize]=-1;
return currentSize++;
}
// insert method to insert the new Date
public void insert(int d) {
if(root==-1){
root=createNode(d);
}
else{
insert(d, 0);
}
}
private void insert( int d, int index) {
if (data[index] == d) {
return;
}
if (data[index] > d) {
if (left[index] == -1) {
left[index] = createNode(d);
} else {
insert(d, left[index]);
}
} else {
if (right[index] == -1) {
right[index] = createNode(d);
} else {
insert(d, right[index]);
}
}
return;
}
public void print(){
print(root);
System.out.println();
}
private void print(int index){
if(index!=-1){
print(left[index]);
System.out.print(data[index] + " ");
print(right[index]);
}
}
public static void main(String[] args) {
// Creating the object of BinarySearchTree class
BinarySearchTree bst = new BinarySearchTree();
// call the method insert
bst.insert(8);
bst.insert(5);
bst.insert(9);
bst.insert(3);
bst.insert(7);
bst.print();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

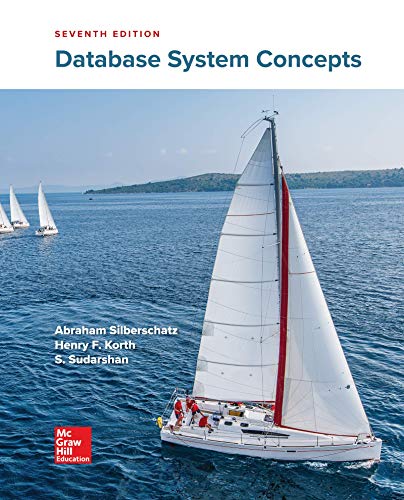
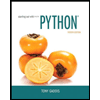
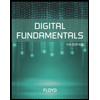
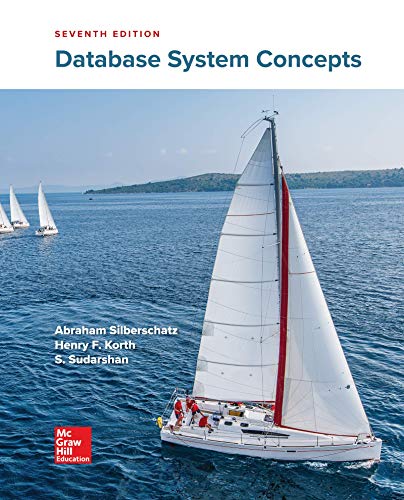
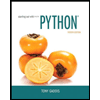
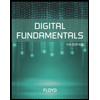
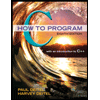
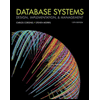
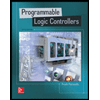