How do I display the highest GPA between three students? The code is below: #include #include using namespace std; //declare Student Class class Student { //private member of class private: string Full_Name; float Student_GPA; int Student_Rank; //public class members public: //function to get student name void setStudentdata(string SN, float GPA, int rank) { Full_Name = SN; Student_GPA = GPA; Student_Rank = rank; } //return student name function void getStudentdata(string &SN, float &GPA, int &rank) { SN = Full_Name; GPA = Student_GPA; rank = Student_Rank; } }; int main() { Student info[3]; //variable declaration string studentName[3]; float studentGpa; int studentRank; //prompt user to enter name GPA and rank for (int i = 0; i < 3; i++) { cout << "\nEnter record for Student " << i + 1; cout << "\nName: "; cin.get(); getline(cin >> ws, studentName[i]); cout << "GPA: "; cin >> studentGpa; cout << "rank: "; cin >> studentRank; //set name GPA and rank in array info[i].setStudentdata(studentName[i], studentGpa, studentRank); } //Shows the students that are eligible for scholarship cout << "\nThe Following students are eligible for scholarship: "; for (int i = 0; i < 3; i++) { info[i].getStudentdata(studentName[i], studentGpa, studentRank); if (studentGpa >= 3.0) { cout << "\n " << studentName[i] << "\n"; cout << "Congratulations!" << endl; } the bold above is where I want it to change to show the highest GPA } system("pause"); return 0; }
How do I display the highest GPA between three students? The code is below:
#include <iostream>
#include <string>
using namespace std;
//declare Student Class
class Student
{
//private member of class
private:
string Full_Name;
float Student_GPA;
int Student_Rank;
//public class members
public:
//function to get student name
void setStudentdata(string SN, float GPA, int rank)
{
Full_Name = SN;
Student_GPA = GPA;
Student_Rank = rank;
}
//return student name function
void getStudentdata(string &SN, float &GPA, int &rank)
{
SN = Full_Name;
GPA = Student_GPA;
rank = Student_Rank;
}
};
int main()
{
Student info[3];
//variable declaration
string studentName[3];
float studentGpa;
int studentRank;
//prompt user to enter name GPA and rank
for (int i = 0; i < 3; i++)
{
cout << "\nEnter record for Student " << i + 1;
cout << "\nName: ";
cin.get();
getline(cin >> ws, studentName[i]);
cout << "GPA: ";
cin >> studentGpa;
cout << "rank: ";
cin >> studentRank;
//set name GPA and rank in array
info[i].setStudentdata(studentName[i], studentGpa, studentRank);
}
//Shows the students that are eligible for scholarship
cout << "\nThe Following students are eligible for scholarship: ";
for (int i = 0; i < 3; i++)
{
info[i].getStudentdata(studentName[i], studentGpa, studentRank);
if (studentGpa >= 3.0)
{
cout << "\n " << studentName[i] << "\n";
cout << "Congratulations!" << endl;
}
the bold above is where I want it to change to show the highest GPA
}
system("pause");
return 0;
}

Step by step
Solved in 2 steps with 1 images

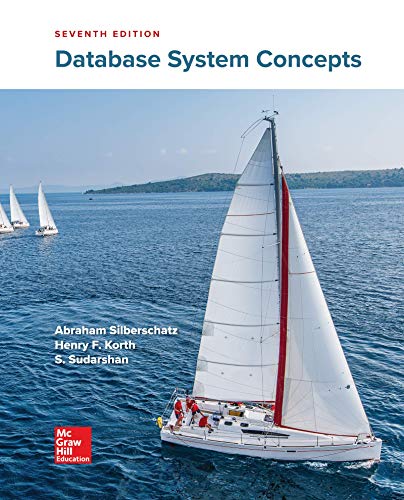
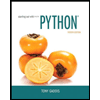
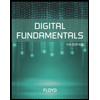
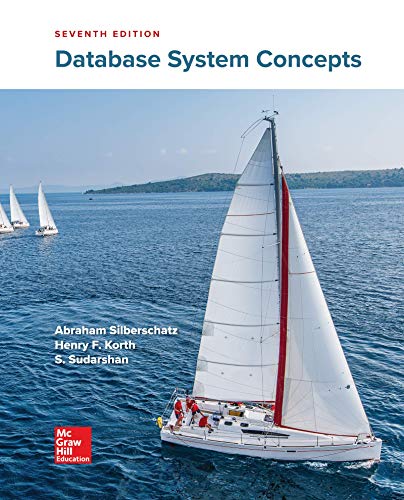
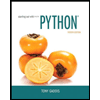
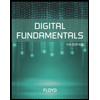
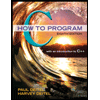
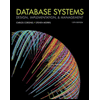
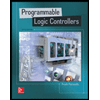