Help me please: 1. Consider the definition of the following class: class employee //Line 1 { //Line 2 public: //Line 3 employee(); //Line 4 employee(string, int, double); //Line 5 employee(int, double); //Line 6 employee(string); //Line 7 void setData(string, int, double); //Line 8 void print() const; //Line 9 void updateSalary(double x); //Line 10 int getNumOfServiceYears() const; //Line 11 double getSalary() const; //Line 12 private: //Line 13 string name; //Line 14 int numOfServiceYears; //Line 15 double salary; //Line 16 }; //Line 17 Now answer the following questions: a. Give the line number containing the constructor that is executed in each of the following declarations: b. employee tempEmployee; c. employee newEmployee("Harry Miller", 0, 25000); d. employee oldEmployee("Bill Dunbar", 15, 55000); e. Write the definition of the constructor in Line 4 so that the instance variables are initialized to "", 0, and 0.0, respectively. f. Write the definition of the constructor in Line 5 so that the instance variables are initialized according to the parameters. g. Write the definition of the constructor in Line 6 so that the instance variable name is initialized to the empty string and the remaining instance variables are initialized according to the parameters.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Help me please:
1. Consider the definition of the following class:
class employee //Line 1 { //Line 2 public: //Line 3 employee(); //Line 4 employee(string, int, double); //Line 5 employee(int, double); //Line 6 employee(string); //Line 7
void setData(string, int, double); //Line 8 void print() const; //Line 9 void updateSalary(double x); //Line 10 int getNumOfServiceYears() const; //Line 11 double getSalary() const; //Line 12
private: //Line 13 string name; //Line 14 int numOfServiceYears; //Line 15 double salary; //Line 16 }; //Line 17
Now answer the following questions:
a. Give the line number containing the constructor that is executed in each of the following declarations:
b. employee tempEmployee;
c. employee newEmployee("Harry Miller", 0, 25000);
d. employee oldEmployee("Bill Dunbar", 15, 55000);
e. Write the definition of the constructor in Line 4 so that the instance variables are initialized to "", 0, and 0.0, respectively.
f. Write the definition of the constructor in Line 5 so that the instance variables are initialized according to the parameters.
g. Write the definition of the constructor in Line 6 so that the instance variable name is initialized to the empty string and the remaining instance variables are initialized according to the parameters.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

I do not understand the answers for a through g. Would someone help explain how to proceed with this question with answers that might be easier to understand?
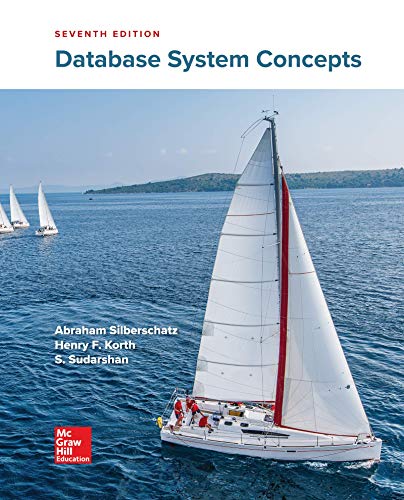
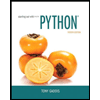
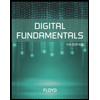
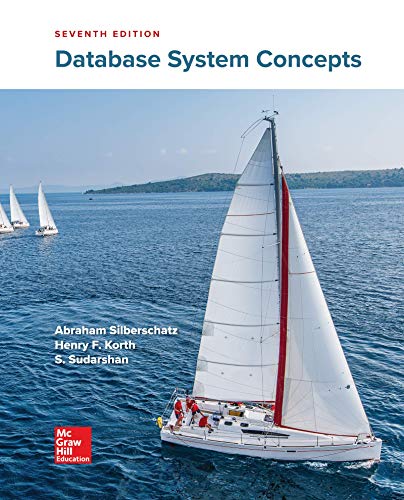
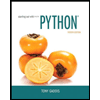
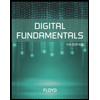
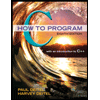
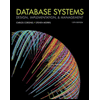
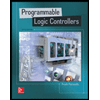