Hello. I need help changing the C++ code so that the highlighted portions are can be entered by the user. See the attached picture for clarity. Thank you. #include #include
Hello. I need help changing the C++ code so that the highlighted portions are can be entered by the user. See the attached picture for clarity. Thank you.
#include <iostream>
#include <iomanip>
#define swap(v1, v2, temp) temp = v1, v1 = v2, v2 = temp;
using namespace std;
inline void generateRC4SBox(uint8_t s[256] /*=sbox*/, const uint8_t* key, int keylength)
{
for(int i = 0; i < 256; i++)
s[i] = i;
uint8_t j = 0, swap;
for(int i = 0; i < 256; i++)
{
j += s[i] + key[i % keylength]; //The addition is in fact modulo 256
swap(s[i], s[j], swap); //Exchange s[i] and s[j]
}
}
inline void RC4(const uint8_t* message, uint8_t* cipher, int start, int stop, uint8_t s[256] /*=sbox*/)
{
uint8_t i = 0, j = 0, swap;
for(int n = start; n < stop; n++)
{
j += s[++i]; //Note that i is effectively increased by one before the rest is evaluated
swap(s[i], s[j], swap); //Exchange s[i] and s[j]
if(message && cipher)
cipher[n] = s[(uint8_t)(s[i]+s[j])]^message[n]; //Cast inside [..] is needed!
}
}
int main(int, char**)
{
const uint8_t *key = (uint8_t *)"66OlSO8L7KoW44awcg2xHJ9X1FbOoF4z", *message = (uint8_t *)"Plaintext";
const int key_length = 32, message_length = 9; //I do not include terminating 0 character. One might as well include it.
uint8_t sbox[256], *cipher = new uint8_t[message_length];
cout<<"RC4 ENCRYPTION"<<endl<<endl<<"preparing sbox (key scheduling) with password \""<<key<<"\"..."<<endl;
generateRC4SBox(sbox, key, key_length);
cout<<"skipping first 3072 bytes and encrypting..."<<endl<<endl;
RC4(0, 0, 0, 3072, sbox); //"Fast forward by 3072 bytes" = "encrypt nonexistent data"
RC4(message, cipher, 0, message_length, sbox); // The actual encryption is done here.
cout<<"MESSAGE: "; for(int n = 0; n < message_length; n++) cout<<hex<<setw(2)<<(int)message[n]<<' '; cout<<" (=\""<<message<<"\")"<<endl;
cout<<"CIPHER: "; for(int n = 0; n < message_length; n++) cout<<hex<<setw(2)<<(int) cipher[n]<<' '; cout<<endl;
delete[] cipher; //Standard cleanup for data allocated on the heap
cipher = 0;
return 0;
}
![int main(int,
{
const uint8_t *key
*message =
|const int key_length = 32, message_length = 9; //I do not include
terminating 0 character. One might as well include it.
uint8_t sbox[256], *cipher
char**)
(uint8_t *)"660lS08L7KoW44awcg2xHJ9X1Fb0oF4z",
(uint8_t *)"Plaintext";
new uint8_t[message_length];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5f9cc468-5045-4ba5-8445-556d55f6f682%2Fbd90141a-6b7b-409c-955b-20a06b4da0c3%2Ffmnzd78_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

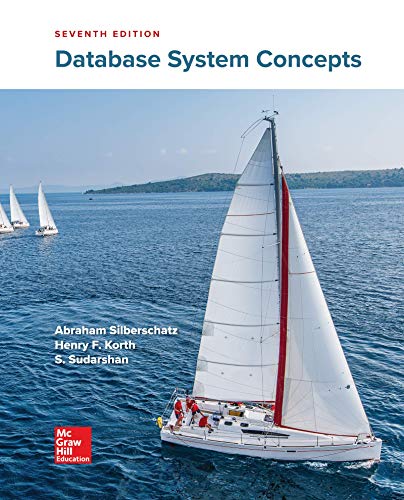
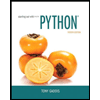
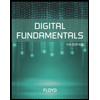
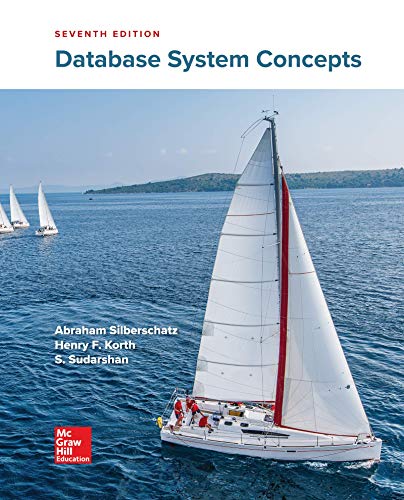
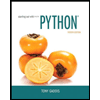
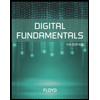
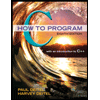
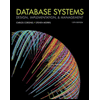
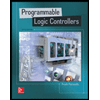