Hello I would like help completing this in c++
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello I would like help completing this in c++

Transcribed Image Text:### Constructing a Simple C++ Class with Constructor: CarRecord
This snippet demonstrates a basic C++ class with the use of a constructor. The task is to initialize certain member variables upon the creation of a class object. Below is the sample output followed by the code.
#### Sample Output
```
Year: 0, VIN: -1
Year: 2009, VIN: 444555666
```
### Code Explanation:
```cpp
#include <iostream>
using namespace std;
class CarRecord {
public:
void SetYearMade(int originalYear);
void SetVehicleIdNum(int vehIdNum);
void Print() const;
CarRecord();
private:
int yearMade;
int vehicleIdNum;
};
// FIXME: Write constructor, initialize year to 0, vehicle ID num to -1.
/* Your solution goes here */
```
### Detailed Breakdown:
1. **Include and Namespace**:
- The `<iostream>` library is included to facilitate input and output.
- The `std` namespace is used for standard C++ library objects and functions.
2. **Class Definition (`CarRecord`)**:
- **Public Members**:
- `SetYearMade(int originalYear)`: Sets the manufacturing year.
- `SetVehicleIdNum(int vehIdNum)`: Sets the vehicle identification number.
- `Print() const`: A method to print the current state of the object.
- `CarRecord()`: Constructor to be defined.
- **Private Members**:
- `yearMade`: An integer to store the year the car was made.
- `vehicleIdNum`: An integer for the vehicle identification number.
3. **Task**:
- Implement the `CarRecord` constructor to initialize `yearMade` to 0 and `vehicleIdNum` to -1.
- Inside the code, there is a section marked with `FIXME` and `/* Your solution goes here */` where the constructor logic needs to be written.
This C++ example is used to teach the fundamentals of class definitions and object initialization using constructors. It is a foundational concept in object-oriented programming.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
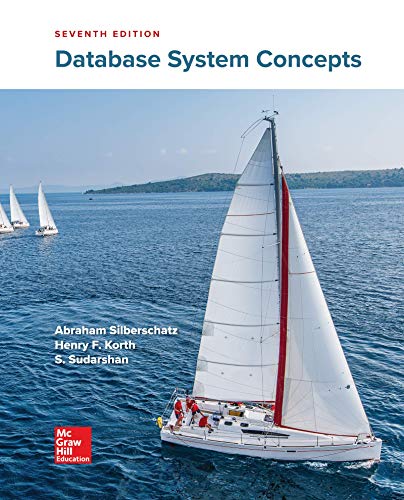
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
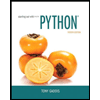
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
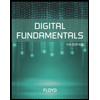
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
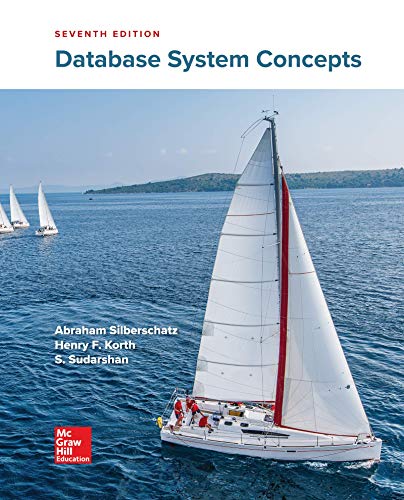
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
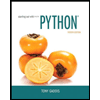
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
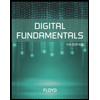
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
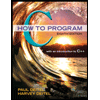
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
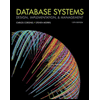
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
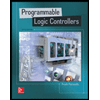
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education