Hello I would like to know how to do this in c++
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello I would like to know how to do this in c++

Transcribed Image Text:### Understanding C++ Constructors
#### Task
Define a constructor as indicated in the code template below. Aim to match the given sample output:
**Sample Output:**
```
Year: 0, VIN: -1
Year: 2009, VIN: 444555666
```
#### Code Template
```cpp
#include <iostream>
using namespace std;
class CarRecord {
public:
void SetVehicleIdNum(int vehIdNum);
void Print() const;
CarRecord();
private:
int yearMade;
int vehicleIdNum;
};
// FIXME: Write constructor, initialize year to 0, vehicle ID num to -1.
/* Your solution goes here */
void CarRecord::SetYearMade(int originalYear) {
yearMade = originalYear;
}
void CarRecord::SetVehicleIdNum(int vehIdNum) {
vehicleIdNum = vehIdNum;
}
```
#### Instructions
1. **Constructor Initialization:**
- Initialize `yearMade` to `0`.
- Initialize `vehicleIdNum` to `-1`.
2. **Explanation of Methods:**
- `SetVehicleIdNum(int vehIdNum)`: Sets the vehicle identification number.
- `SetYearMade(int originalYear)`: Sets the year the car was made.
- `Print() const`: Outputs the current values of `yearMade` and `vehicleIdNum`.
3. **Testing Your Constructor:**
- After implementing the constructor, use the provided `Run` button to test your solution.
- Ensure the sample output is correctly displayed.
#### Interface Features
- To the right of the code, an interface shows test status. Here, one test is passed, and all tests passed indicators confirm your code's correctness.
#### Feedback
- Provide feedback via the "Feedback?" link for improving this educational content.
This exercise provides a fundamental understanding of defining and implementing basic constructors in C++.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
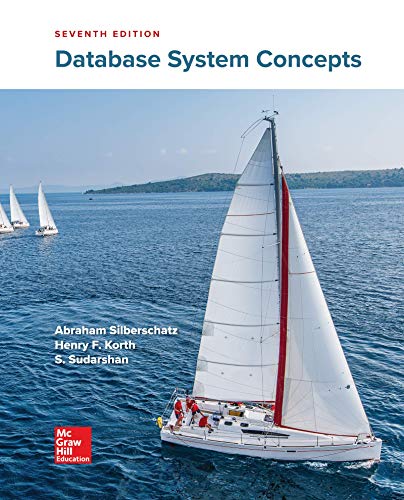
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
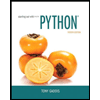
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
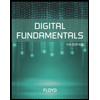
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
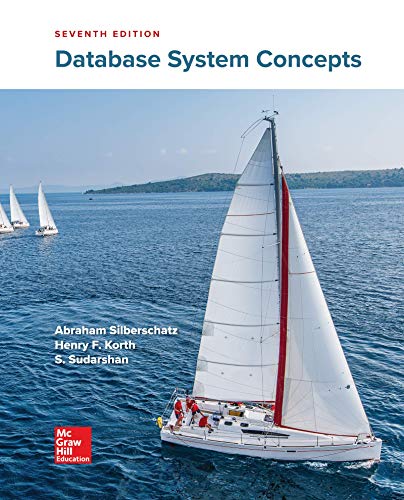
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
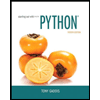
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
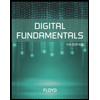
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
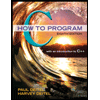
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
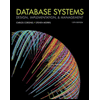
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
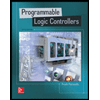
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education