Hello, I need help with this c++ program. Arithmetic.cpp #include #include #include "ArrayStack.h" float eval(std::string); // The following main function should not be modified int main(void) { std::string expression = "Continue"; do { std::cout << "Enter an arithmetic expression on a single line ('q' to end): "; getline(std::cin, expression); if (expression[0] != 'q') { float result = eval(expression); std::cout << expression << " = " << result << std::endl; } } while (expression[0] != 'q'); return 0; } float eval(std::string expr) { // To be implemented // Any number of support functions may also be added std::cout << "eval function not implemented" << std::endl; return 0; } ArrayStack.cpp #include #include "ArrayStack.h" #include "StackException.h" template ArrayStack::ArrayStack() : top(-1) { // top = -1; } //ArrayStack::ArrayStack(const ArrayStack& orig) { //} // //ArrayStack::~ArrayStack() { //} template bool ArrayStack::isEmpty() const { return (top < 0); } template T ArrayStack::peek() const { //If isEmpty(), what do I return? //use precondition, if it fails program halts assert(!isEmpty()); return items[top]; } template bool ArrayStack::pop() { bool result = false; // if (!isEmpty()) { // top--; // result = true; // } // return result; if (!isEmpty()) top--; else throw StackException("Pop called on an empty stack"); return result; } template bool ArrayStack::push(const T& newItem) { bool result = false; if (top < SIZE -1) { top++; items[top] = newItem; result = true; } else { throw StackException("Push called on a full stack"); } return result; } ArrayStack.h #ifndef ARRAYSTACK_H #define ARRAYSTACK_H #include "StackADT.h" const int SIZE = 50; template class ArrayStack : StackADT { public: ArrayStack(); // ArrayStack(const ArrayStack& orig); // virtual ~ArrayStack(); //interface methods bool isEmpty() const; bool push(const T& newItem); bool pop(); T peek() const; private: // static const int SIZE = 50; T items[SIZE]; int top; }; #include "ArrayStack.cpp" #endif /* ARRAYSTACK_H */ Main.cpp #include #include #include "ArrayStack.h" #include "StackException.h" #include using namespace std; /* * */ int main() { ArrayStack intStack; cout << "Beginning " << boolalpha << intStack.isEmpty() << endl; for (int i=1; i<=5; i++) { // try { intStack.push(i); // } catch (StackException e) { // cout << e.what() << endl; // } } cout << "After push " << boolalpha << intStack.isEmpty() << endl; //check copy constructor ArrayStack numStack(intStack); cout << "Copied item: " << numStack.peek() << endl; intStack.pop(); cout << "Copied item: " << intStack.peek() << endl; cout << "Copied item: " << numStack.peek() << endl; cout << "Contents: "; int sum = 0; while (!intStack.isEmpty()) { cout << intStack.peek() << " "; sum += intStack.peek(); try { intStack.pop(); } catch (StackException e) { cout << e.what() << endl; } } cout << endl << "Sum: " << sum << endl; ArrayStack strStack; strStack.pop(); cout << "Did a problem occur with pop? " << endl; // intStack.peek(); // cout << "Did a problem occur with peek? " << endl; return 0; } StackADT.h #ifndef STACKADT_H #define STACKADT_H template class StackADT { public: virtual bool isEmpty() const = 0; virtual bool push(const T& newItem) = 0; virtual bool pop() = 0; virtual T peek() const = 0; }; #endif /* STACKADT_H */ StackException.cpp #include #include "StackException.h" StackException::StackException(const std::string& msg) : logic_error (msg) { } StackException.h #ifndef PUSHEXCEPTION_H #define PUSHEXCEPTION_H #include #include class StackException : public std::logic_error { public: StackException(const std::string& msg = ""); private: }; #endif /* PUSHEXCEPTION_H */
Hello, I need help with this c++ program.
Arithmetic.cpp
#include <cstdlib>
#include <iostream>
#include "ArrayStack.h"
float eval(std::string);
// The following main function should not be modified
int main(void) {
std::string expression = "Continue";
do {
std::cout << "Enter an arithmetic expression on a single line ('q' to end): ";
getline(std::cin, expression);
if (expression[0] != 'q') {
float result = eval(expression);
std::cout << expression << " = " << result << std::endl;
}
} while (expression[0] != 'q');
return 0;
}
float eval(std::string expr)
{ // To be implemented
// Any number of support functions may also be added
std::cout << "eval function not implemented" << std::endl;
return 0;
}
ArrayStack.cpp
#include <cassert>
#include "ArrayStack.h"
#include "StackException.h"
template <class T>
ArrayStack<T>::ArrayStack() : top(-1) {
// top = -1;
}
//ArrayStack::ArrayStack(const ArrayStack& orig) {
//}
//
//ArrayStack::~ArrayStack() {
//}
template <class T>
bool ArrayStack<T>::isEmpty() const {
return (top < 0);
}
template <class T>
T ArrayStack<T>::peek() const {
//If isEmpty(), what do I return?
//use precondition, if it fails program halts
assert(!isEmpty());
return items[top];
}
template <class T>
bool ArrayStack<T>::pop() {
bool result = false;
// if (!isEmpty()) {
// top--;
// result = true;
// }
// return result;
if (!isEmpty())
top--;
else
throw StackException("Pop called on an empty stack");
return result;
}
template <class T>
bool ArrayStack<T>::push(const T& newItem) {
bool result = false;
if (top < SIZE -1) {
top++;
items[top] = newItem;
result = true;
} else {
throw StackException("Push called on a full stack");
}
return result;
}
ArrayStack.h
#ifndef ARRAYSTACK_H
#define ARRAYSTACK_H
#include "StackADT.h"
const int SIZE = 50;
template <class T>
class ArrayStack : StackADT<T> {
public:
ArrayStack();
// ArrayStack(const ArrayStack& orig);
// virtual ~ArrayStack();
//interface methods
bool isEmpty() const;
bool push(const T& newItem);
bool pop();
T peek() const;
private:
// static const int SIZE = 50;
T items[SIZE];
int top;
};
#include "ArrayStack.cpp"
#endif /* ARRAYSTACK_H */
Main.cpp
#include <cstdlib>
#include <iostream>
#include "ArrayStack.h"
#include "StackException.h"
#include <stdexcept>
using namespace std;
/*
*
*/
int main() {
ArrayStack<int> intStack;
cout << "Beginning " << boolalpha << intStack.isEmpty() << endl;
for (int i=1; i<=5; i++) {
// try {
intStack.push(i);
// } catch (StackException e) {
// cout << e.what() << endl;
// }
}
cout << "After push " << boolalpha << intStack.isEmpty() << endl;
//check copy constructor
ArrayStack<int> numStack(intStack);
cout << "Copied item: " << numStack.peek() << endl;
intStack.pop();
cout << "Copied item: " << intStack.peek() << endl;
cout << "Copied item: " << numStack.peek() << endl;
cout << "Contents: ";
int sum = 0;
while (!intStack.isEmpty()) {
cout << intStack.peek() << " ";
sum += intStack.peek();
try {
intStack.pop();
} catch (StackException e) {
cout << e.what() << endl;
}
}
cout << endl << "Sum: " << sum << endl;
ArrayStack<string> strStack;
strStack.pop();
cout << "Did a problem occur with pop? " << endl;
// intStack.peek();
// cout << "Did a problem occur with peek? " << endl;
return 0;
}
StackADT.h
#ifndef STACKADT_H
#define STACKADT_H
template <class T>
class StackADT {
public:
virtual bool isEmpty() const = 0;
virtual bool push(const T& newItem) = 0;
virtual bool pop() = 0;
virtual T peek() const = 0;
};
#endif /* STACKADT_H */
StackException.cpp
#include <string>
#include "StackException.h"
StackException::StackException(const std::string& msg) : logic_error (msg) {
}
StackException.h
#ifndef PUSHEXCEPTION_H
#define PUSHEXCEPTION_H
#include <stdexcept>
#include <string>
class StackException : public std::logic_error {
public:
StackException(const std::string& msg = "");
private:
};
#endif /* PUSHEXCEPTION_H */



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

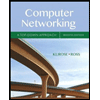
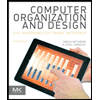
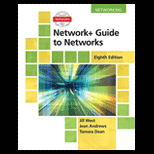
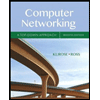
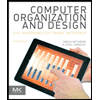
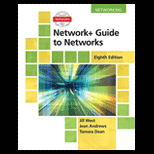
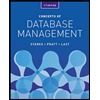
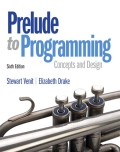
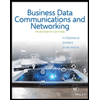